Fungible tokens in asynchronous networks
The adoption of EIP-20 on the Ethereum network has enabled the creation of a wide range of coins based on smart contracts. New fungible tokens have become the basis for managing third-party blockchain projects and transferring value within the Ethereum ecosystem. The architecture of the Ethereum blockchain and the early implementation of the protocol led to some implementation flaws, for example, the smart contract of the token stores information about all holders, which greatly increases the physical size of the blockchain.
TIP-3 on Everscale and Venom networks
Since in Everscale a single smart contract can only be processed in one shard, the use of one central token smart contract could greatly reduce the throughput of the entire network. Thus, a new approach to the implementation of smart contracts of fungible tokens has been developed. Today, Everscale recommends writing TIP-3 tokens in accordance with implementation from our developers.
The essence of the implementation is that there is a smart contract in the network TokenRoot
which:
Stores data about the token:
The name of the token;
Token ticker;
The size of the fractional part;
The address of the smart contract that owns the token;
Issue of a token;
Allows the contract owner to issue new tokens;
Allows you to burn tokens.
Allows you to create smart contracts wallets and credit them with tokens via mint.
IN previous article we described the interaction of smart contracts according to the actor model, on the basis of which the Everscale and Venom blockchains are built. Smart contracts exchange messages through which smart contract methods are called. Contract TokenRoot
is no exception. To use its functionality, you must send an internal message to it. All messages sent to the contract address TokenRoot
are being validated. In case the message was sent from a smart contract whose address does not match the address of the contract owner TokenRoot
this message is discarded and not processed by the smart contract.
deployWallet
Method deployWallet
smart contract TokenRoot
can be called by anyone:
function deployWallet(
address walletOwner,
uint128 deployWalletValue
) public override responsiblereturns (address tokenWallet) {
require(
walletOwner.value != 0,
TokenErrors.WRONG_WALLET_OWNER
);
tvm.rawReserve(_reserve(), 0);
tokenWallet = _deployWallet(
_buildWalletInitData(walletOwner),
deployWalletValue,
msg.sender
);
return {
value: 0,
flag: TokenMsgFlag.ALL_NOT_RESERVED,
bounce: false
} tokenWallet;
}
As a result, a new smart contract will appear on the network, on the balance of which you can store contract tokens TokenRoot
.
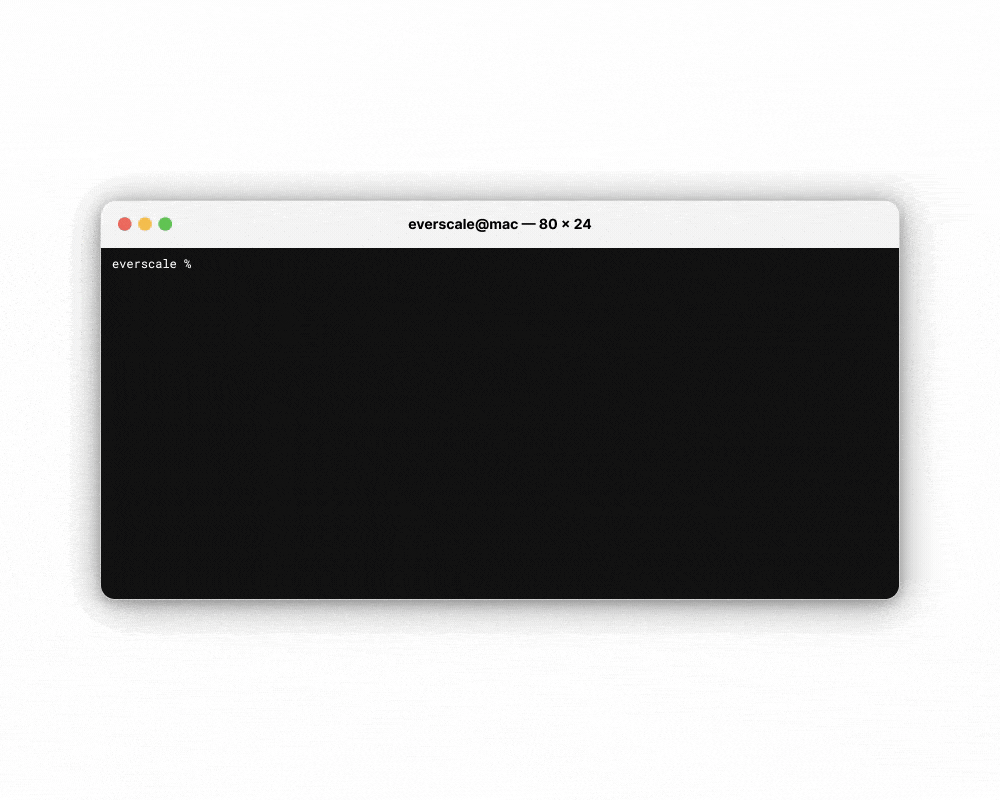
Sending TIP-3 tokens
The smart contract itself Wallet
has all the necessary methods for managing balances, we are interested in transfer and acceptTransfer
:
function transfer(
uint128 amount,
address recipient,
uint128 deployWalletValue,
address remainingGasTo,
bool notify,
TvmCell payload
)
override
external
onlyOwner
{
require(amount > 0, TokenErrors.WRONG_AMOUNT);
require(amount <= balance_, TokenErrors.NOT_ENOUGH_BALANCE);
require(recipient.value != 0 && recipient != owner_, TokenErrors.WRONG_RECIPIENT);
tvm.rawReserve(_reserve(), 0);
// получение кода смарт-контракта получателя
TvmCell stateInit = _buildWalletInitData(recipient);
address recipientWallet;
if (deployWalletValue > 0) {
recipientWallet = _deployWallet(stateInit, deployWalletValue, remainingGasTo);
} else {
recipientWallet = address(tvm.hash(stateInit));
}
balance_ -= amount;
// Вызов метода acceptTransfer у контракта-получателя
ITokenWallet(recipientWallet).acceptTransfer{ value: 0, flag: TokenMsgFlag.ALL_NOT_RESERVED, bounce: true }(
amount,
owner_,
remainingGasTo,
notify,
payload
);
}
Method transfer
, called by the sender, reduces the balance on the account and sends the tokens to the recipient. The sender can also deploy the wallet for the recipient and send funds to it. If the receiving smart contract does not respond with a transfer processing error message, the reduced balance and transaction information is stored in the state network.

Calling a method acceptTransfer
, the sender’s smart contract finds out the possibility of receiving funds by the addressee and, if the transaction is successful, changes in its own balance by the number of sent tokens are stored in the code. The balance of the recipient’s contract, in case of successful completion of the transaction, is increased by the number of sent tokens.
function acceptTransfer(
uint128 amount,
address sender,
address remainingGasTo,
bool notify,
TvmCell payload
)
override
external
functionID(0x67A0B95F)
{
// Проверка адреса контракта-отправителя
require(msg.sender == address(tvm.hash(_buildWalletInitData(sender))), TokenErrors.SENDER_IS_NOT_VALID_WALLET);
tvm.rawReserve(_reserve(), 2);
balance_ += amount;
if (notify) {
IAcceptTokensTransferCallback(owner_).onAcceptTokensTransfer{
value: 0,
flag: TokenMsgFlag.ALL_NOT_RESERVED + TokenMsgFlag.IGNORE_ERRORS,
bounce: false
}(
root_,
amount,
sender,
msg.sender,
remainingGasTo,
payload
);
} else {
remainingGasTo.transfer({ value: 0, flag: TokenMsgFlag.ALL_NOT_RESERVED + TokenMsgFlag.IGNORE_ERRORS, bounce: false });
}
}

Since transactions in Everscale and Venom are executed asynchronously, smart contracts operate on constantly changing data. Accordingly, the mechanism for completely canceling the results of a transaction cannot be laid down at the protocol level, as is typical, for example, of the Ethereum blockchain, where operations are performed atomically on a previously known data array, changes to which will occur only if the transaction is successful.
In TVM networks, the handling of exceptions that occur during the execution of transactions and rollback to the original state are embedded in the logic of smart contracts. TIP-3 tokens are no exception: if the acceptTransfer method cannot be processed, the smart contract sends a message that the sender’s balance change should be cancelled.
Creation of wallets and validation of actors available for interaction
In Everscale, the smart contract address is calculated as follows:
hash(contract code + static variables)
Thus, both the sending contract and the receiving contract know each other’s code, because it is hashed into addresses, and, therefore, both contracts know the tokens of which TokenRoot
will be transferred and that the tokens of this TokenRoot
received legally:

Thanks to this implementation, interaction with the smart contract of the fungible token itself is not required, as happens in Ethereum:
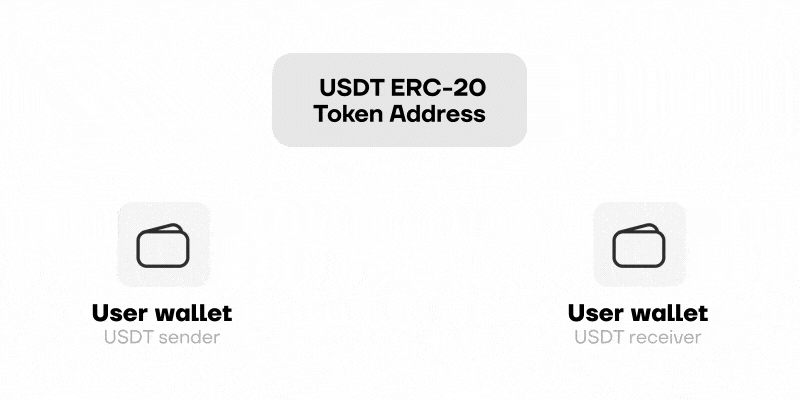
Among other things, the user of the wallet application can be sure that he receives the correct tokens, otherwise, all users of this application will receive tokens of another TokenRoot
contract, which will instantly highlight the error in the integration of the token.
Distributed Computing – Implementing Everscale’s Scaling Mechanisms
Each user balance in a particular token is a separate smart contract, which means that with a high network load and the need to process transactions in several shards, smart contract data transactions can be processed by different validator nodes, which leads to scalability .
As long as the network load is not high, all smart contracts can be in the same shard, and their transactions can be validated by one validator. A similar situation, only on a larger scale, is true for all synchronous blockchains, where the entire blockchain is one shard in an asynchronous network:

However, as the load grows, synchronous networks cannot increase the number of simultaneously validating nodes and parallelize the load. In Everscale and Venom – asynchronous blockchains – shards can be divided into several, and the number of shards in one workchain can grow up to 256 units. Accordingly, up to 256 validators can be simultaneously allocated for transaction processing.
The animation below demonstrates how, with a high load on the network, smart contracts TokenWallet
exchange messages with smart contracts from other shards – the resulting transactions are processed by nodes from the corresponding groups, taking into account the logical time of transactions:

Careful control over the amount of data in the blockchain
Since the balance of a single token is a smart contract owned by a single user, TokenRoot
contract, there is no need to store the address of each token holder.
ERC-20 tokens, in turn, store data about each holder, which leads to an endless accumulation of data and an infinite growth in the physical size of the blockchain:

If a single wallet contract ceases to be used, sooner or later the funds for payment storageFee
run out, and the contract will first be frozen, and then completely removed from the blockchain. Thus, the problem of the endless accumulation of data in the blockchain is partly solved:
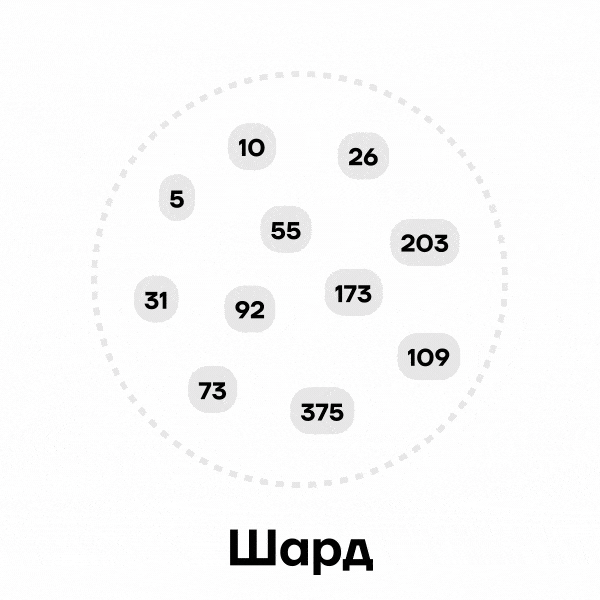
With the mass adoption of blockchain technologies in the future, the requirements for network scalability will only become tougher: the ability to asynchronously execute operations with tokens opens up opportunities for building a CBDC system on a highly scalable blockchain.
In addition, the more familiar on-chain stablecoin transactions may also require more network bandwidth with a significant increase in the number of users. It is obvious that the existing L1 solutions with a synchronous architecture will not be able to provide the proper throughput and, as a result, such blockchains will use mechanisms to reduce the demand for network services, the result of which, for example, may be an excessive increase in network fees.