We are writing a Telegram bot with an application! (Python)
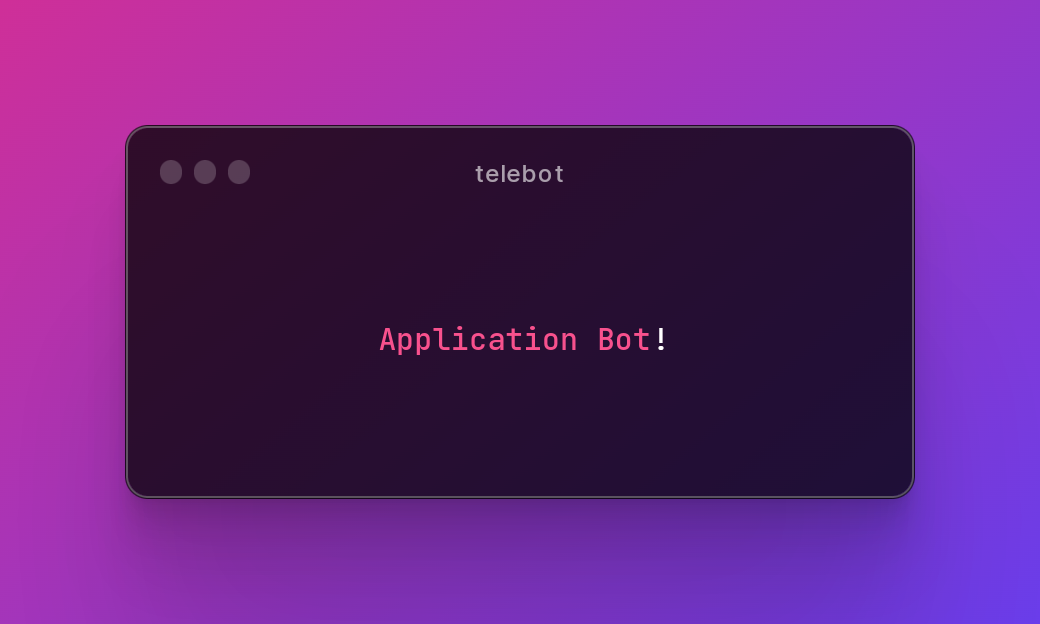
Today, we will write a Telegram Bot with an application. For example: Users fill out questionnaires and then this data is sent to the Administrator.
For this we will use the pyTelegramBotAPI (telebot) library.
pyTelegramBotAPI is a lightweight and good library for writing Telegram Bot!
Library installation
For installation on Windows the telebot library itself, we need to open cmd or a terminal and write:
pip install pyTelegramBotAPI
For installation on macOS you need to write:
pip3 install pyTelegramBotAPI
Beginning of code
First you need to get our bot token. For this you need to go to @BotFather and create a bot. After you get a token and you need to insert it into a variable TOKEN which we will create now, you need to insert instead of YOUR_TOKEN .
And so, we first import the library itself:
import telebot
TOKEN = 'YOUR_TOKEN'
ADMIN_ID = 'YOUR_ID'
In a variable TOKEN we will store our token received from BotFather
In a variable ADMIN_ID we need to insert our id from the telegram account. Your ID can be found through the bot @getmyid_bot
Now let’s write the bot’s greeting:
import telebot
TOKEN = 'YOUR_TOKEN'
ADMIN_ID = 'YOUR_ID'
bot = telebot.TeleBot(TOKEN)
name = None
username = None
user1_mess = None
user2_mess = None
user3_mess = None
@bot.message_handler(commands=['start'])
def start_message(message):
global name, username
name = message.from_user.first_name
username = message.from_user.username
bot.send_message(message.chat.id, "Привет! Это бот для заявок. Чтобы подать заявку, заполните анкеты")
bot.polling()
We have 4 variables. Variables name And username we make it global and they need to store the username of the user from the telegram and the name. Now let’s check if our bot is working. To do this, you first need to write at the end of the code:
bot.polling()
As we can see, the bot works!

Now let’s get down to the surveys:
import telebot
TOKEN = 'YOUR_TOKEN'
ADMIN_ID = 'YOUR_ID'
bot = telebot.TeleBot(TOKEN)
name = None
username = None
user1_mess = None
user2_mess = None
user3_mess = None
@bot.message_handler(commands=['start'])
def start_message(message):
global name, username
name = message.from_user.first_name
username = message.from_user.username
bot.send_message(message.chat.id, "Привет! Это бот для заявок. Чтобы подать заявку, заполните анкеты")
form1(message)
And yes, in a function start_message don’t forget to write at the end form1(message)
thanks to which, after the greeting, it throws us to the next function, which we will now create.
Let’s write the first question:
def form1(message):
bot.send_message(message.chat.id, "1. Как вас зовут?")
bot.register_next_step_handler(message, form2)
Thanks to the code bot.register_next_step_handler(message, form2)
the bot waits until the user sends a message, and after it is sent, it moves to the next function form2, now we will create it
We make the second function:
def form2(message):
global user1_mess
user1_mess = message.text
bot.send_message(message.chat.id, "2. Сколько вам лет?")
bot.register_next_step_handler(message, form3)
Making a variable user1_mess global and change its value to message.text. It will store the user’s text. This variable holds the text entered in the previous function.
We do the same with the third function:
def form3(message):
global user2_mess
user2_mess = message.text
bot.send_message(message.chat.id, "3. Укажите номер телефона:")
bot.register_next_step_handler(message, result)
You probably noticed that in the code bot.register_next_step_handler(message, result)
this time points to a function result. Let’s create it
Making a function result:
def result(message):
global user3_mess
user3_mess = message.text
bot.send_message(message.chat.id, "Спасибо за заявку! Скоро наш менеджер свяжется с вами. Всего доброго!")
admin_app(ADMIN_ID)
Here we just write a message and code admin_app(ADMIN_ID)
which throws to the function admin_app.
Recall that the variables user3_mess, user2_mess And user1_mess store the texts entered in the previous function.
And so, we create a function to send the entered user data to the Administrator / Manager
Making a function admin_app to send data to admin:
def admin_app(ADMIN_ID):
ankets = f'''Новая заявка от {name}!
Имя: {user1_mess}
Возраст: {user2_mess}
Номер телефона: {user3_mess}
Telegram:
Name: {name}
Username: @{username}'''
bot.send_message(ADMIN_ID, ankets)
Create a variable ankets where the completed user profiles will be and sent to the Admin. Do you remember that we created a variable ADMINID? So, user applications will be sent to this account with this ID.
Do not forget to write at the end of the code:
bot.polling()
Full code:
import telebot
TOKEN = 'YOUR_TOKEN'
ADMIN_ID = 'YOUR_ID'
bot = telebot.TeleBot(TOKEN)
name = None
username = None
user1_mess = None
user2_mess = None
user3_mess = None
@bot.message_handler(commands=['start'])
def start_message(message):
global name, username
name = message.from_user.first_name
username = message.from_user.username
bot.send_message(message.chat.id, "Привет! Это бот для заявок. Чтобы подать заявку, заполните анкеты")
form1(message)
def form1(message):
bot.send_message(message.chat.id, "1. Как вас зовут?")
bot.register_next_step_handler(message, form2)
def form2(message):
global user1_mess
user1_mess = message.text
bot.send_message(message.chat.id, "2. Сколько вам лет?")
bot.register_next_step_handler(message, form3)
def form3(message):
global user2_mess
user2_mess = message.text
bot.send_message(message.chat.id, "3. Укажите номер телефона:")
bot.register_next_step_handler(message, result)
def result(message):
global user3_mess
user3_mess = message.text
bot.send_message(message.chat.id, "Спасибо за заявку! Скоро наш менеджер свяжется с вами. Всего доброго!")
admin_app(ADMIN_ID)
def admin_app(ADMIN_ID):
ankets = f'''Новая заявка от {name}!
Имя: {user1_mess}
Возраст: {user2_mess}
Номер телефона: {user3_mess}
Telegram:
Name: {name}
Username: @{username}'''
bot.send_message(ADMIN_ID, ankets)
bot.polling()
Let’s run and check:

Hooray! Everything works great!
Also you can in a variable ADMIN_ID specify the id of your group where you want to send notifications of new applications (do not forget to add the bot to the group).