strings, tuples, sets, dictionaries
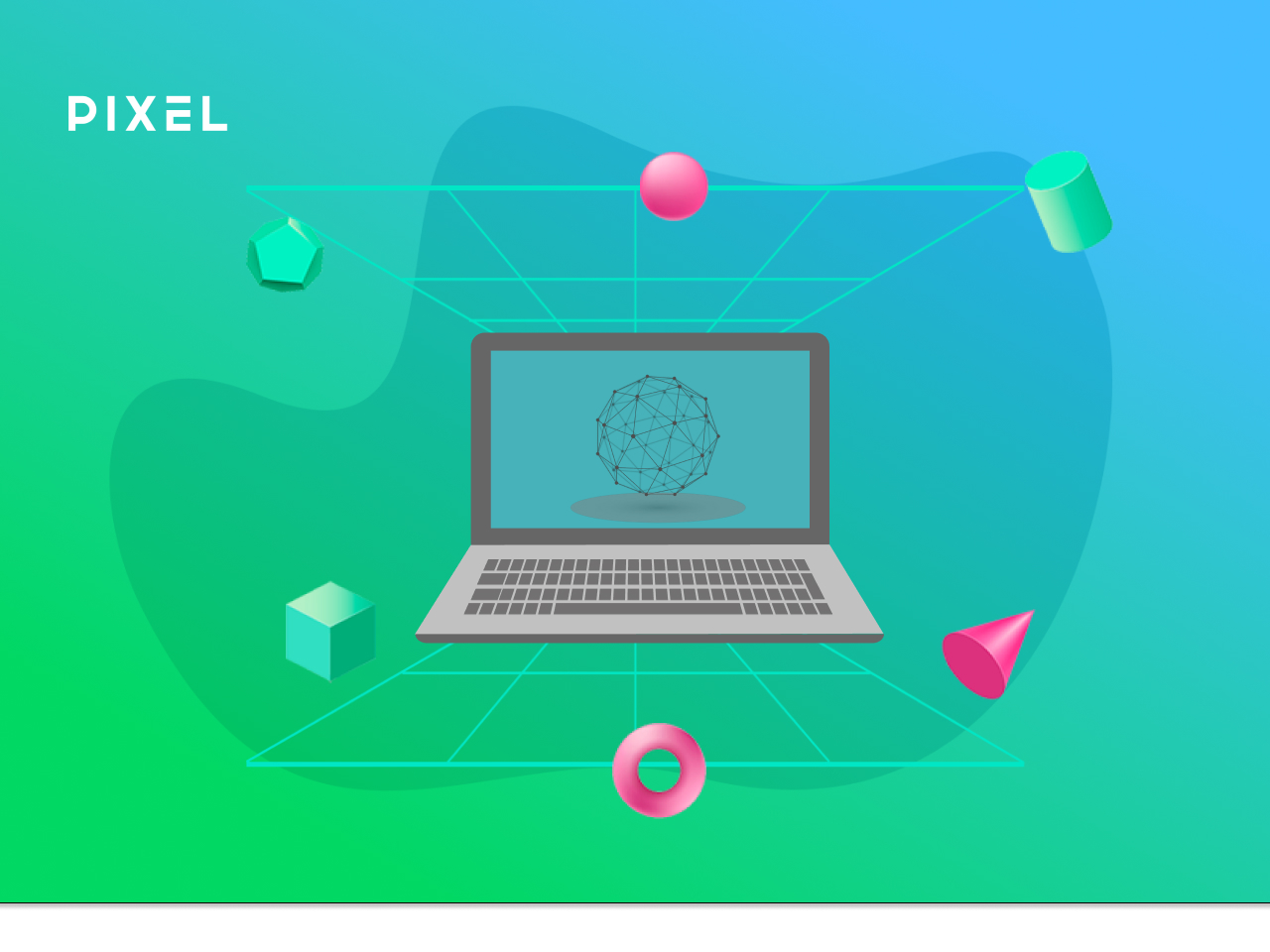
Programming is like magic. You give the command spells to the machine, and it does whatever you want.
Want a flower pot to water itself? Build an Arduino robot kit and write a simple program for it. The irrigation system is ready. You will only need to add water occasionally, but you can teach your robot this too. The spell will need to be complicated. If you have already started learning Python programming, then you don’t know so many “spells” yet.
In this article, you will learn about the Python tool called lists. With them, you will be able to pour information like a magician pours elixirs to get some kind of witchcraft potion.
Python language for children: explaining what lists are (lists)
Datasets can also be stored as a string. Here is a string type variable that lists various spells from Harry Potter:
wizard_list="Accio - приносит потеряные предметы, Alohomora - открывает двери, Avada Kadavra - вызывает мгновенную смерть, Brackium Emendo - лечит сломанные кости"
print(wizard_list)
And here is what we got in the developer console after running this code:

But we have one more possibility: we can make a list of Python, that is, we can create such a magical construct of this language as list to solve the same problem. With it, we can then perform a variety of manipulations.
wizard_list = ['Accio - приносит потеряные предметы', 'Alohomora - открывает двери', 'Avada Kadavra - вызывает мгновенную смерть', 'Brackium Emendo - лечит сломанные кости']
print(wizard_list)

You may have noticed that it takes a little more typing to create the list, but it’s worth it. Lists allow you to manage data much more freely. For example, you can very easily get and, for example, print the third element of the list to the console. By the way, this is a common Python task for kids in programming courses. Try to remember how it’s done. To solve it, you need to specify the element number in square brackets next to the name of the variable that you named your list of spells. Numbers, elements that are assigned to them when creating a list automatically, without our participation, are called identifiers and we can use them if these numbers help to solve a particular problem.
wizard_list = ['Accio - приносит потеряные предметы', 'Alohomora - открывает двери', 'Avada Kadavra - вызывает мгновенную смерть', 'Brackium Emendo - лечит сломанные кости']
print(wizard_list[2])

“Aha!” you say. “This is not the second, but the third element!”
Yes, but in the lists the numbering starts from “0”. Don’t ask why it happened. This tradition goes back to the dark magic of the C programming language, which Python itself is written in. It turns out that the first element has the identifier “0”, the second – “1”, and so on.
Also, with lists, we can change the element we need much more easily than if the dataset is represented by a string. Imagine that our list of spells is a register for beginning magicians from the computer of the Hogwarts library. Just like you take a Python course for kids from scratch, magicians learn the basics of witchcraft as children! If we are talking about initial training, then a spell that causes instant death will be superfluous in it. Let’s add something less terrible there.
wizard_list = ['Accio - приносит потеряные предметы', 'Alohomora - открывает двери', 'Avada Kadavra - вызывает мгновенную смерть', 'Brackium Emendo - лечит сломанные кости']
wizard_list[2] = 'Riddikulus - специальное заклинание для борьбы со зверем боггартом из мира Хогвартса'
print(wizard_list)

See? The item with ID “2” has been replaced with a new one. That’s far from everything a Python list can do. By adding a construction of the form to the list name [0:2] we will get a selection from the list from zero to the second element. Here’s how it works:
wizard_list = ['Accio - приносит потеряные предметы', 'Alohomora - открывает двери', 'Avada Kadavra - вызывает мгновенную смерть', 'Brackium Emendo - лечит сломанные кости']
print(wizard_list[0:3])

Write [0:3] is equivalent to saying, “Give me all the elements of the list from zero to third.” In lists, you can store data of any type available to Python. It can be strings, it can be numbers, or you can put both in the list.
wizard_list = ['Accio - приносит потеряные предметы', 1000555,
'Alohomora - открывает двери', 555,
'Avada Kadavra - вызывает мгновенную смерть',
'Brackium Emendo - лечит сломанные кости']
print(wizard_list)

Finally, an option that is extremely useful for magic: storing other lists inside a list.
wizard_list = [[555, 1000555, 345], ['Accio - приносит потеряные предметы',
'Alohomora - открывает двери',
'Avada Kadavra - вызывает мгновенную смерть',
'Brackium Emendo - лечит сломанные кости']]
print(wizard_list)

You can add new items to the list. To do this, use the special function “append”. Perhaps you are not yet familiar with the concept of “function”? This is a block of code that does something. In this case, “append” adds the new spell to the end of the wizard_list.
wizard_list = ['Accio - приносит потеряные предметы',
'Alohomora - открывает двери',
'Avada Kadavra - вызывает мгновенную смерть',
'Brackium Emendo - лечит сломанные кости']
wizard_list.append('Expecto patronum - накладывает защитные чары')
print(wizard_list)
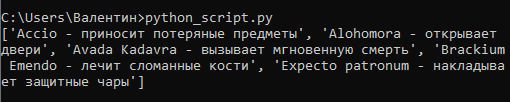
We can remove elements from a list using the “del” command. This is an abbreviation for “delete” – to delete. And the identifier will help us with this again. del[4] and the function just added with the “append” function is gone. You can do a lot more with lists. You can add two lists. “general_list = wizard_list + creature_list”. In this case, you get one big list, in which the abilities of magical creatures are added after all the elements of the magician’s list. You can also multiply the list. wizard_list*5 will result in four copies being added to the end of the original list. In other words, you will have five Emendo spells on the list, just with different identifiers. Other list arithmetic operations are not available. If you try to add a number to a list or divide by a number, Python will return an error.
Python Language for Kids: Tuples
The complexity of Python list operations is that the data of the process of metamorphosis is easy to change or distort. Tuples come to the rescue. The tuple cannot be changed. It is important for a magician to make sure that in a certain place he will find only what he expects, and not “mandrake root”. Unlike lists, you create tuples using parentheses. It can also store data of any type. Store in tuples data that you are not going to change, thanks to this you save “manna” – computer memory. The example below creates a tuple and attempts to change one of its elements. The Python interpreter naturally refuses this.
numbers_tuple = (345,
985656,
4566,
7889)
numbers_tuple[0] = 10
print(numbers_tuple)
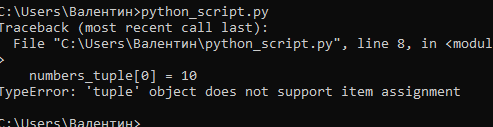
Python Language for Kids: Dictionaries
Dictionaries in Python are useful when you need to store key-value pairs. By the way, in English this type can be called differently: map, dict, dictionary, in all three cases the same thing is meant. If similar items that are listed in the dictionary have the same key, then on this basis they are easy to select and process in the code, even if they are mixed with a variety of other data. There are countless situations where having a key can be very useful. Suppose we store data in the Hogwarts library computer using a dictionary. Let’s quickly find the Helga Hufflepuff Cup from the mass of recorded items.
marvelous_things = {'diadem': "'Rowena ravenclaw's diadem'",
'sword': 'sword of Gryfindor',
'locket': "'salazar slytherin's locket'",
'cup': "'Helga Hufflepuff's cup'"}
print(marvelous_things['cup'])
See how useful the list is?
Python Language for Kids: Sets
Sets in Python are another useful tool. It differs from all others in the absence of identifiers. Elements are written to it without specifying their order. In practice, this is a very useful property, which, most likely, does not impress you now, but which you will definitely remember when faced with a specific task. A number of useful properties are associated with the absence of “numbers”. For example, only unique elements are written to sets, so sets are good for quickly removing duplicates in a particular dataset. The code below illustrates the absence of numbers in sets, we access the element by id and Python returns an error.
name_of_malefactor = {'L', 'o', 'r', 'd', 'V', 'o', 'l', 'd', 'e', 'm', 'o', 'r', 't'}
print(name_of_malefactor[5])

Python Language for Kids: Collections
You can often find the term “collections” in Python materials. This is a general designation for all the tools listed above. A collection is a programmatic object that stores a set of elements of one or more types. The line in “Python” also applies to collections.
Python for Kids: Learning the Mechanics of Magic
Try to remember as many ways as you can to work with different types of collections. As you know, “dry theory”, and “the tree of life turns green.” Reading time-grayed magical Python books in a tall stone reading room can get you bored at some point. But you will thank yourself for not giving up a boring theory when you can “summon the dragon.” If you can’t concentrate, try to figure out how you can apply what you’re reading about. What would you be interested in conjuring?
The material was prepared and published by the school of programming for children “Pixel”.