How to set up SFML C++ library in Visual Studio 2022 static linking

This article explains how to set up your statically linked SFML C++ projects using the Visual Studio IDE.
Installing the SFML library
Download the SFML library from official website. We select the latest version of the software, today it is version SFML 2.6.1.

You can unpack the downloaded archive into any folder. I unpack to drive C: in the IT folder (C:\IT).
Setting up an SFML project
Create a console application project in Visual Studio.
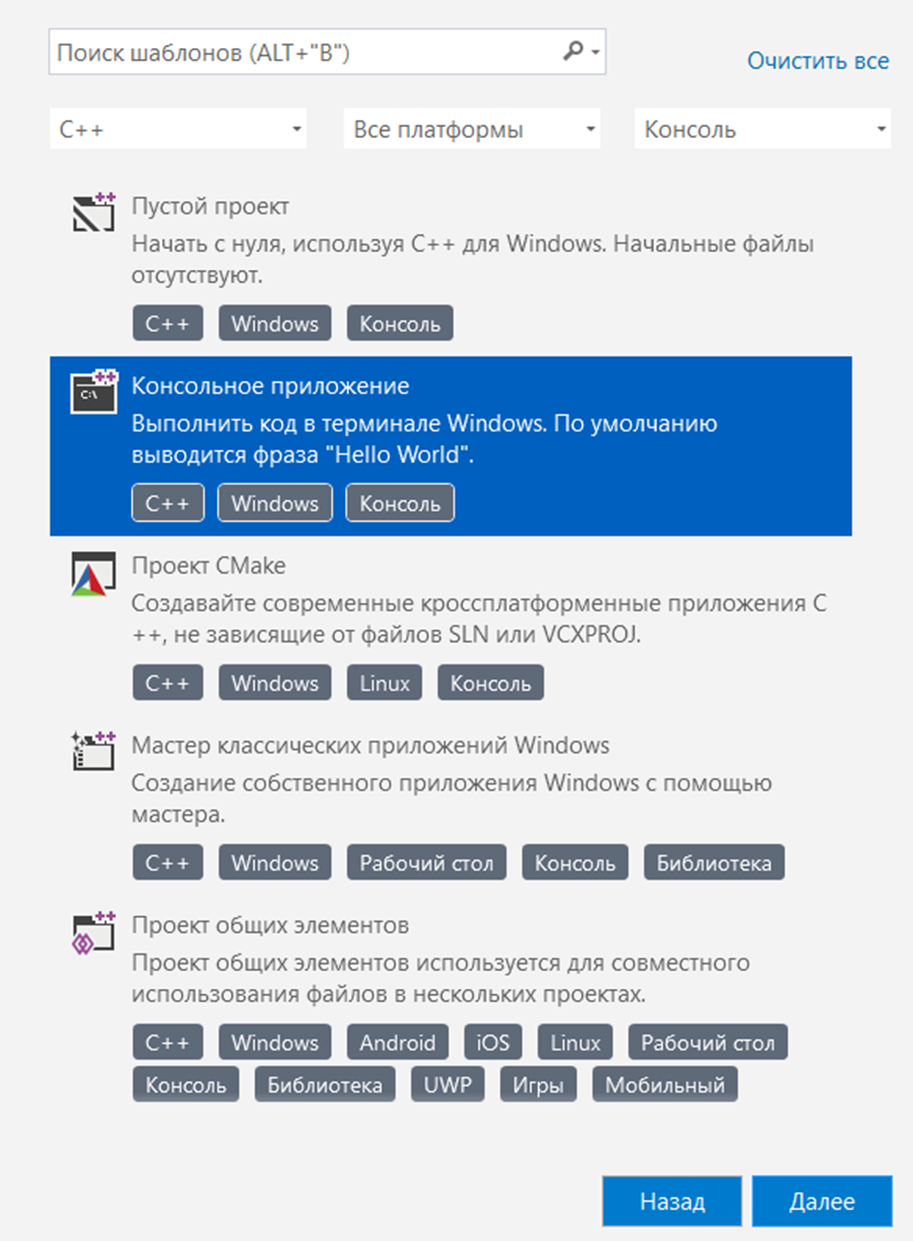
Setting up the created project
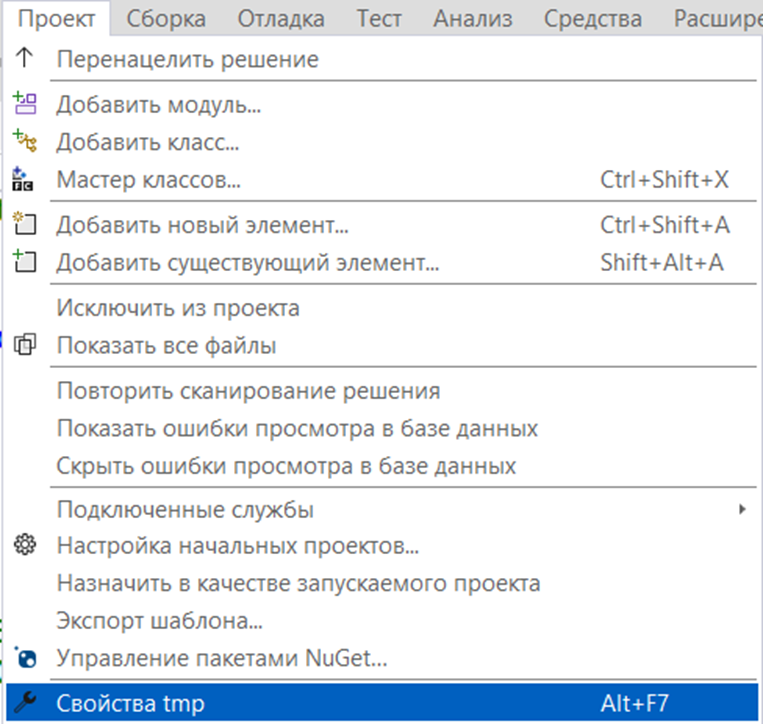
If you are using the 32-bit SFML library then select the win 32 platform, I use the 64-bit and select the x64 platform.
Setting up the C/C++ property
C/C++ -> General -> Additional directories of included files (we include the include directory of the SFML library)
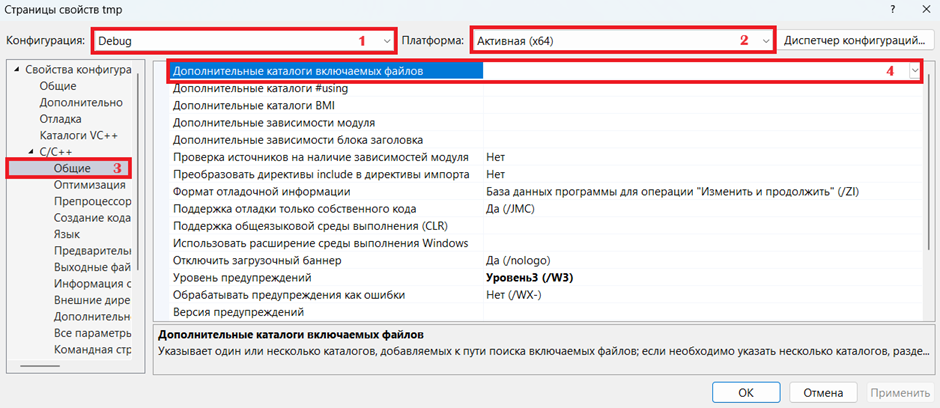

C/C++ -> Preprocessor -> Preprocessor definition (add the SFML_STATIC parameter)
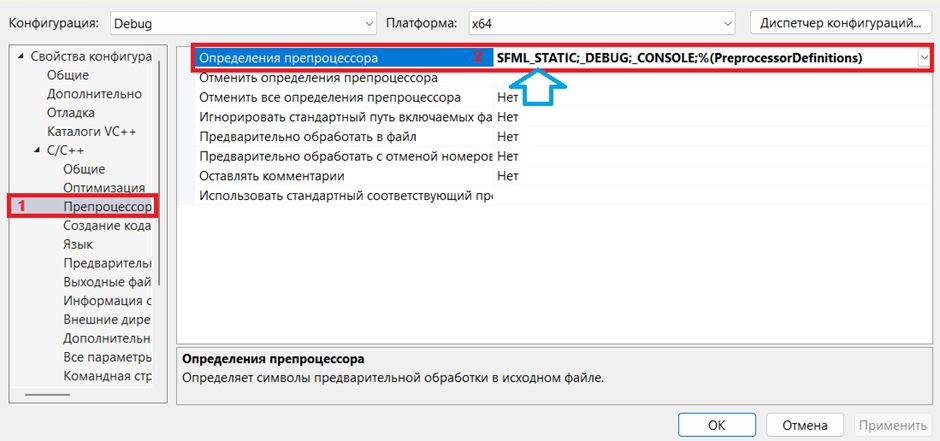
Setting the Linker property
Linker -> General -> Additional library directories (include the lib directory)
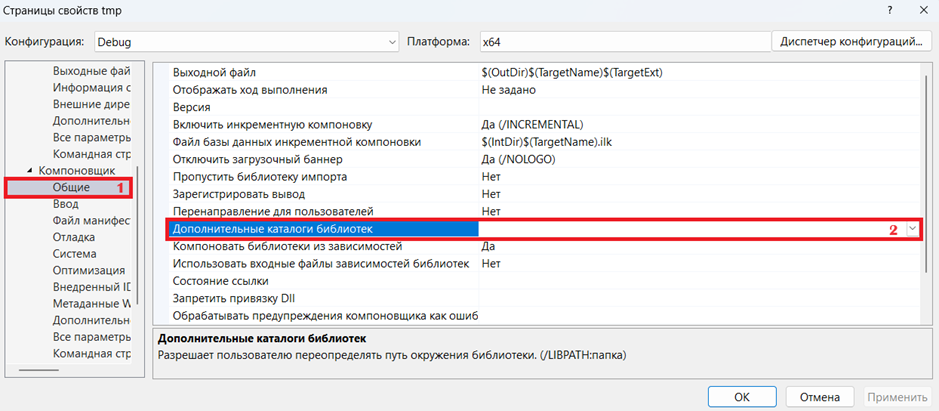

We associate our application with SFML *.lib files
Linker -> Input -> Additional Dependencies

Copy the name of the libraries into the additional dependencies window field
Debug configuration:
sfml-graphics-sd.lib
sfml-window-sd.lib
sfml-system-sd.lib
sfml-audio-sd.lib
sfml-network-sd.lib
opengl32.lib
openal32.lib
freetype.lib
winmm.lib
gdi32.lib
flac.lib
vorbisenc.lib
vorbisfile.lib
vorbis.lib
ogg.lib
ws2_32.lib
Release Configuration:
sfml-system-s.lib
sfml-window-s.lib
sfml-network-s.lib
sfml-audio-s.lib
sfml-graphics-s.lib
opengl32.lib
openal32.lib
ws2_32.lib
winmm.lib
ogg.lib
vorbis.lib
flac.lib
vorbisenc.lib
vorbisfile.lib
freetype.lib
gdi32.lib

To make the console disappear from the screen after launching the SFML project, we make additional settings; they are usually used in the Release configuration.
Linker -> System -> Subsystem (Windows/SUBSYSTEM:WINDOWS)

Create an entry point: mainCRTStartup
Linker -> Advanced -> Entry Point
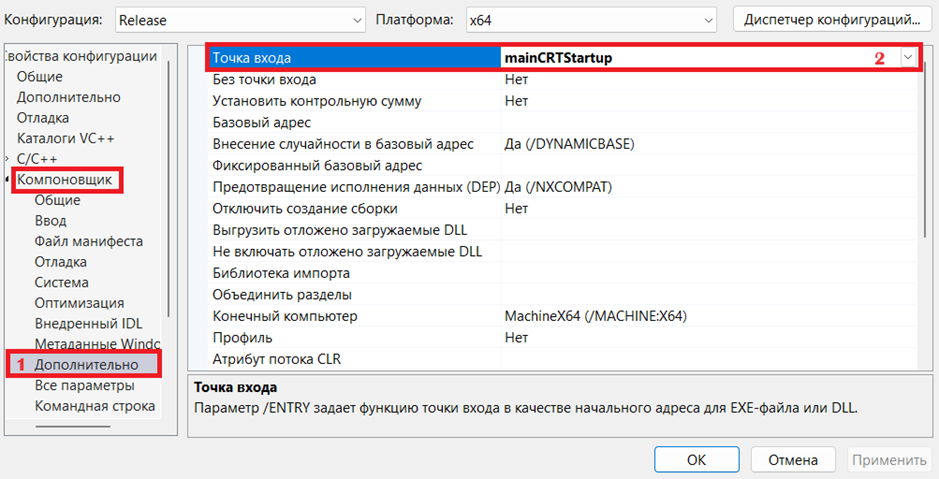
Don't forget to click the apply button at the end.
To work with 3D sound in games, copy the openal32.dll file from the folder SFML-2.6.1\bin\ to a folder Windows\System32\
By default, this file is not present in the Windows operating system.
Code template for SFML C++ library project
#include <SFML/Graphics.hpp>
int main()
{
sf::RenderWindow window(sf::VideoMode(600, 400), L"Иллюзия", sf::Style::Default);
window.setVerticalSyncEnabled(true);
sf::Vector2f a1{100,100};
sf::Vector2f a2{500,200};
sf::Vector2f a3{500,200};
sf::Vector2f a4{100,300};
bool lu = true;
sf::ConvexShape convex;
convex.setPointCount(4);
convex.setFillColor(sf::Color::Magenta);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
if (lu) { a1.y += 1; a4.y -= 1; a2.y -= 1; a3.y += 1; if (a1.y > 200) lu = false; }
else { a1.y -= 1; a4.y += 1; a2.y += 1; a3.y -= 1; if (a1.y < 100) lu = true; }
convex.setPoint(0, a1);
convex.setPoint(1, a2);
convex.setPoint(2, a3);
convex.setPoint(3, a4);
window.clear(sf::Color::Blue);
window.draw(convex);
window.display();
}
return 0;
}

If after executing the program code you see a quadrilateral, then you have done everything correctly by creating your first SFML C++ project with static linking.
Creating a template for SFML C++ projects in the Visual Studio IDE 2022
In our created project, select the menu:
Project->Export Template

In the window that appears, select a project template and click next.

Fill in the following fields: template name, template description, icon image, image view. Place all the checkmarks below and click the done button.
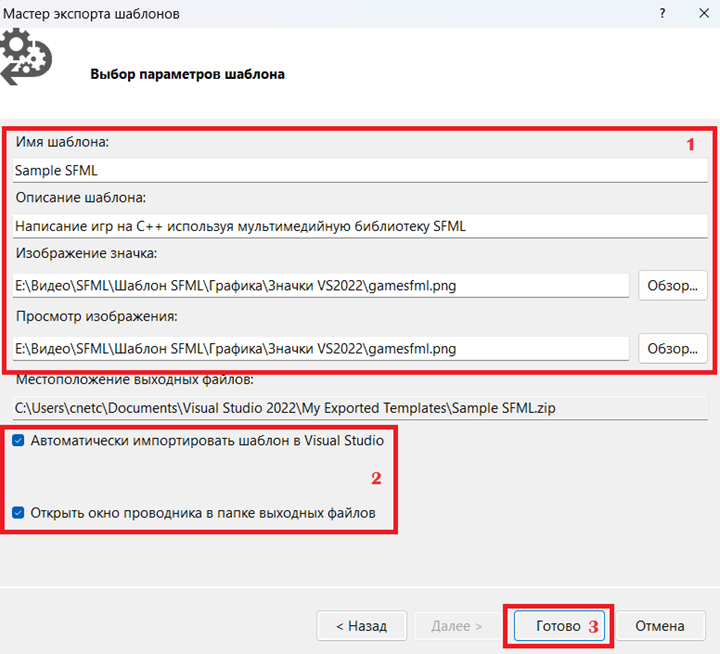
In the explorer window that appears, find the template archive and unpack it.

Go to the folder with the unpacked files and use Notepad to open the MyTemplate.vstemplate file.
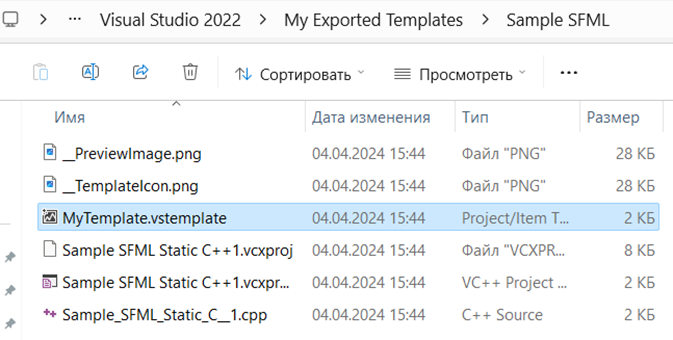
We make the changes indicated in the picture below.
Project file name variable – $safeprojectname$

We add the variable to the next two files.
Sample SFML Static C++1.vcxproj
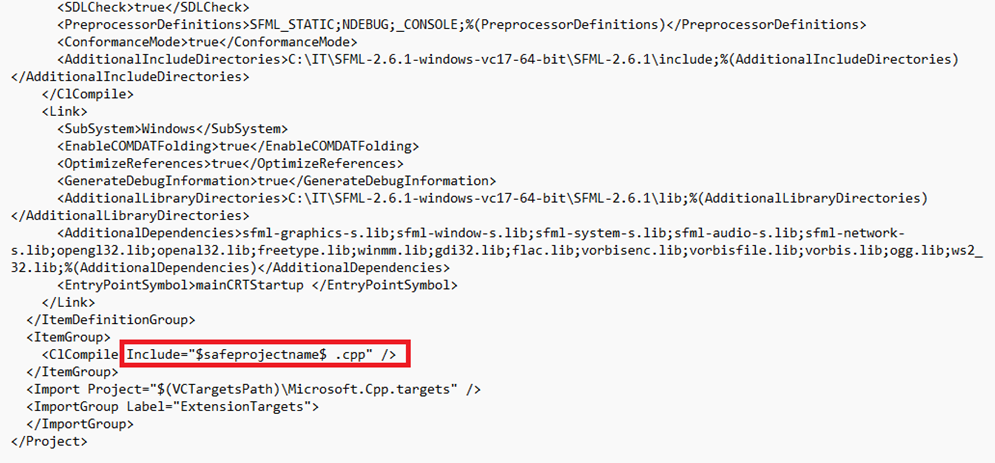
Sample SFML Static C++1.vcxproj.filters

Copy the changed files back to the template archive.

Copy the template archive from the folder
C:\Users\user\Documents\Visual Studio 2022\My Exported Templates
To folder
C:\Users\user\Documents\Visual Studio 2022\Templates\ProjectTemplates
We launch Visual Studio 2022, find our SFML template and use it for our projects.

If something doesn’t work out for you, then you can get more detailed instructions for connecting the SFML library to Visual Studio 2022, as well as creating an SFML library template in Visual Studio, by watching the video “Connecting the SFML C++ library static linking”