How to host a telegram bot (and other Python scripts) on Repl.it for free 24
A very common question is: where can I place Python scripts, Flask application, telegram or discord bots?
One option is on your computer if you have an external IP address and experience in setting up port forwarding on your router. Or other services, usually requiring a paid subscription.
The purpose of this article is a detailed instruction on how to make Python script hosting free and available 24/7 using the example of a telegram bot
Step 0 – Bot Registration
There are a huge number of tutorials on how to get a token, so everything is simple. We find BotFather in telegrams, register a new bot, choose a name for it, get a token like: 127466748171:HJfwijfw88jf32lc9FHjwpfkfgwerhjf
We will need it later

Step 1 – register on Repl.it
Create a new Python project
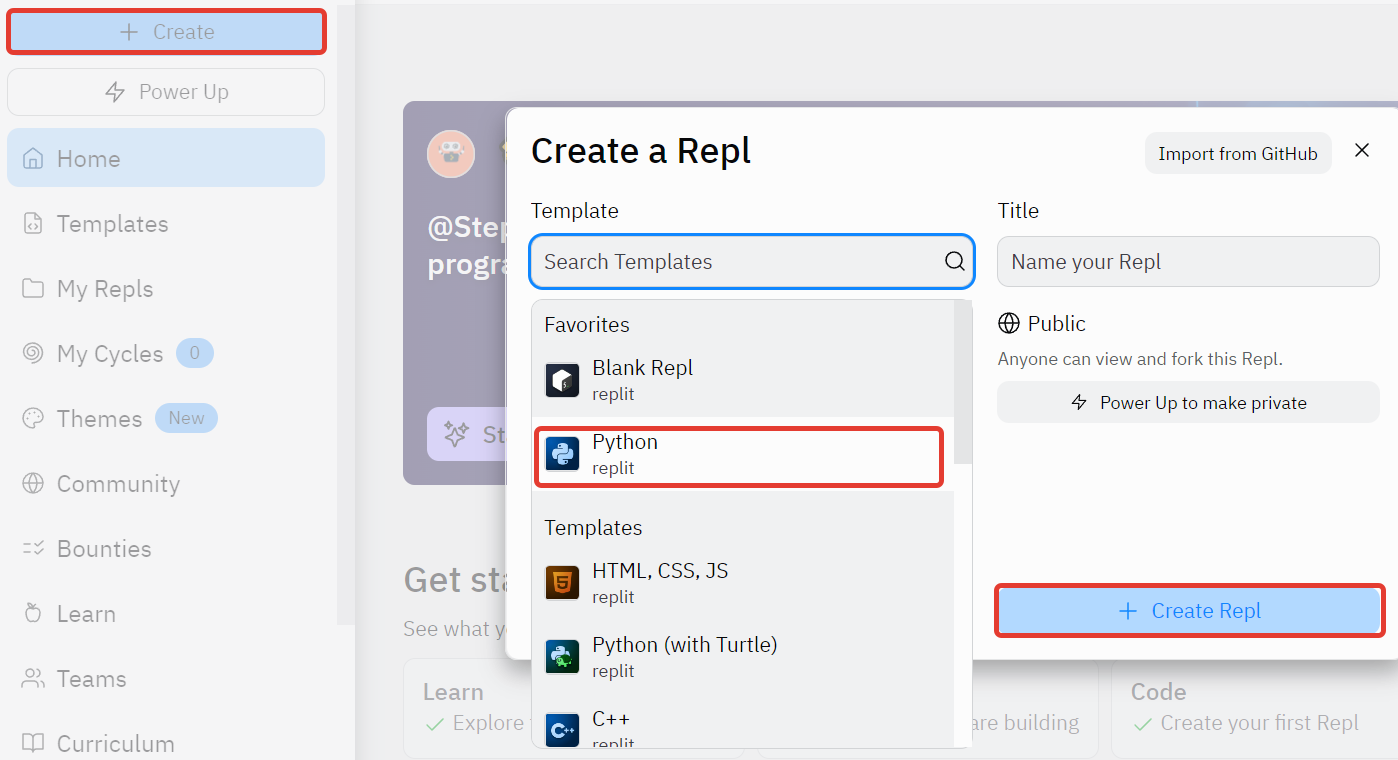
Step 2 – Writing the Bot Code
The main.py file will be created in the project. We place the bot code in it:

Here it is worth paying attention to the installation of the pytelegrambotapi module: import first pip
and then execute it via: pip.main(['install', 'pytelegrambotapi']).
In this case, no additional steps are required for installation at startup.
import os
from background import keep_alive #импорт функции для поддержки работоспособности
import pip
pip.main(['install', 'pytelegrambotapi'])
import telebot
import time
bot = telebot.TeleBot('СЮДА ВСТАВЬТЕ ВАШ ТОКЕН')
@bot.message_handler(content_types=['text'])
def get_text_message(message):
bot.send_message(message.from_user.id,message.text)
# echo-функция, которая отвечает на любое текстовое сообщение таким же текстом
keep_alive()#запускаем flask-сервер в отдельном потоке. Подробнее ниже...
bot.polling(non_stop=True, interval=0) #запуск бота
Step 3 – Create a Flask Server
Create another file in the project background.py
It will run the Flask server, which will receive requests from the monitoring service and be used to keep the ReplIt script running.
Flask is a python module for developing web applications. We will create a server “template” that has only one page, which is required for our task.
The thing is that in the free mode, the running script on Replit will be stopped after some time (10-30 minutes) after closing the browser tab.
However, if a request was made to the web server, the timer is reset and the script continues to run.
from flask import Flask
from flask import request
from threading import Thread
import time
import requests
app = Flask('')
@app.route('/')
def home():
return "I'm alive"
def run():
app.run(host="0.0.0.0", port=80)
def keep_alive():
t = Thread(target=run)
t.start()
It is important that the server is not started directly in the file, but in a separate thread t = Thread(target=run).
This will ensure the simultaneous operation of the Flask server and the telegram bot.

After launch, a link appeared in the upper right corner (will need it later) on which you can see the result of the Flask server (in our case, the message I’m alive).
At this stage, we have an echo-telegram bot and a web server that is accessible from outside at the address of the form: YOUR_REPL.your_nickname.repl.co
However, 10-30 minutes after closing the browser tab, the script will be stopped. The whole trick is that if “someone” periodically opens a link leading to the page of our web server, the scripts will continue to work indefinitely.
Step 4 – Set up the monitoring service
In order for the script to work constantly, we will use the service UpTimerRobot. It will create a request to our web server every 5 minutes and extend its operation time. Registration is not difficult, so let’s move on to the next step.
After logging into your personal account, create a new monitor

In the settings of the new monitor, you need to specify the name and link that we received when running the script above. Time polls indicate – every 5 minutes.
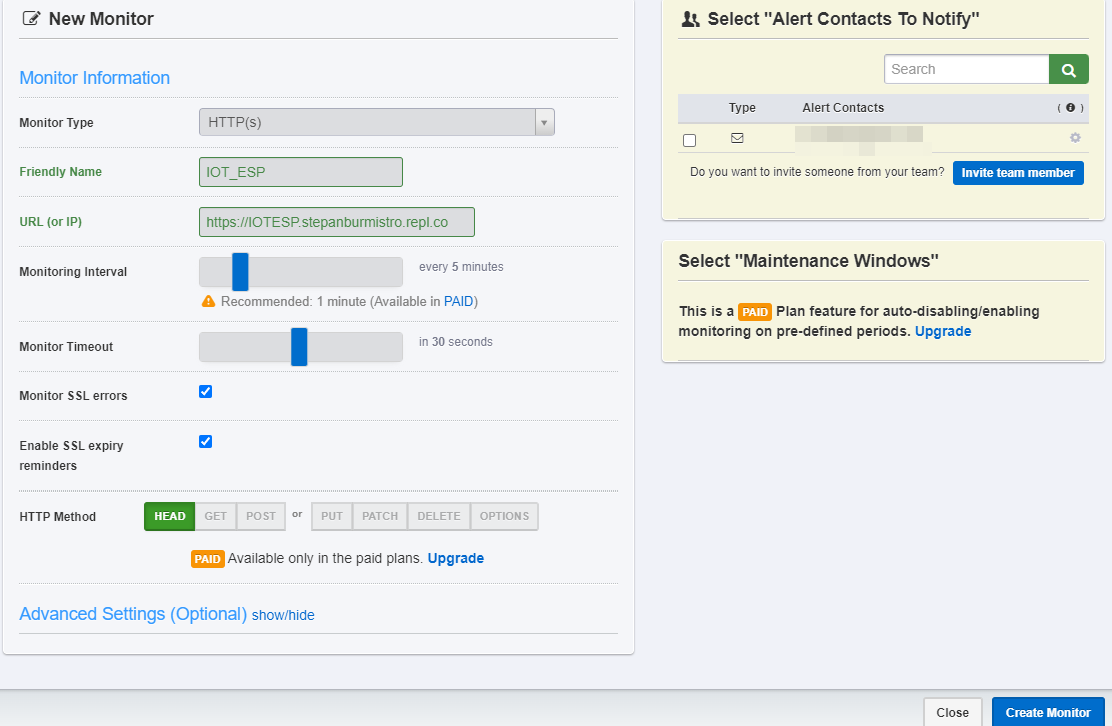
Save the monitor and return to ReplIt. In the server console, we see incoming requests from the monitoring service

This means that everything worked out and our script will work 24/7. You can work on it and develop the project!
So it goes! Good luck!
You don’t need monitoring service : just add new file and run it after keep_live()
the code:
import time
import requests
from datetime import datetime
from threading import Thread
url = ‘WEB_HOST’
headers = {‘User-Agent’: ‘Mozilla/5.0′} # Add your desired headers here
def run():
while True:
# Send GET request with headers
response = requests.get(url, headers=headers)
# Print response status code and timestamp
print(
f’Response status code: {response.status_code} ({datetime.now()})’)
# Wait for 25 minutes before sending the next request
time.sleep(300 * 5)
def code():
t = Thread(target=run)
t.start()