Using PHP for System Administration
The practical importance of PHP for web development is impressive in terms of efficiency and versatility. Most system administrators limit themselves to this. Like C #, PHP can be a powerful tool for the sysadmin, even though PHP is inherently a web development language.
The biggest advantage of PHP for sysadmin is its cross-platform nature. Unlike traditional tools like bash for Linux or bat files and scripts Visual basic for Windows, PHP scripts are easily portable from one operating system to another. All that is needed is compatibility with the PHP interpreter and the installation of the necessary modules.
Read also: An Introduction to Bash Scripting.
How to install PHP on Linux and Windows
If PHP is present on the web server as a web scripting language, then command line capabilities are also present. And that’s enough to use PHP as a system administration tool.
However, the absence services web server does not mean that PHP cannot be installed as a standalone software package. In this case, you must complete the installation according to one of the instructions, depending on the operating system.
Install PHP on Linux
Without getting into distribution-specific package management specifics, the only module that needs to be installed directly is php-cli… “Cli“Means”Command Line Interface”And translates to” Command Line Interface “.
Installation php-cli will set up all the required dependencies for PHP, including the PHP engine itself.
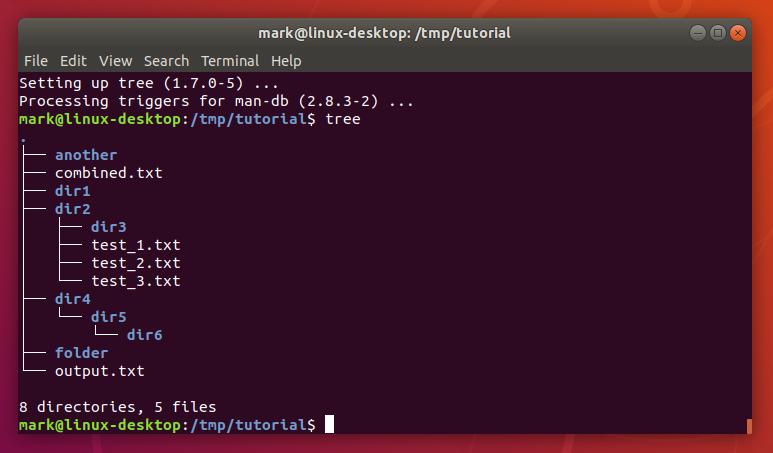
Install PHP on Windows
Typically, PHP is not included in the standard Windows suite, even if installed IIS… The whole PHP package needs to be done manually download and copy it to a separate directory, for example, to the root of the system drive c: php…
After installing PHP in the file php.ini it is necessary to include the various modules to be used.
PHP programming basics and examples
Consider the following code:
<?php
if (isset ($_GET))
print "There are [" . count($_GET) . "] parameters in _GET.n";
if (isset ($_POST))
print "There are [" . count($_POST) . "] parameters in _POST.n";
if (isset ($argc) && isset ($argv))
{
for ($x = 0; $x < $argc; $x++)
{
print "Parameter [" . $x . "] is [" . $argv[$x] . "]n";
}
}
?>
Note that the output lines end with a special sequence / n, providing a new line break. This code will perform a sequential iteration of all command line parameters. The script launch syntax is as follows:
$ php test.php param1 param2
There are [0] parameters in _GET.
There are [0] parameters in _POST.
Parameter [0] is [test.php]
Parameter [1] is [param1]
Parameter [2] is [param2]
In general, executing PHP scripts is no different from web scripts. In both cases, there are input parameters. For HTML, this is data obtained using the methods GET and POST, and for the command line these are variables $ argc and $ argv, the essence of which is the number of arguments, including the name of the script itself, and their values, respectively.
It should be noted that it is considered good practice to check whether a value is set for variables $ _GET, $ _POST, $ argc or $ argv and identify before reading. Especially if the same script will be used both in the web environment and on the command line.
Read also: How to Manage Linux Users from the Terminal.
How to back up MySQL databases using PHP
The advantage of using PHP for sysadmin is that you can use all the extensible features that PHP offers that might not be available in other environments. For example, the only way to interact with the MySQL database is for the shell bash or Windows batch file is the use of pipelined and intermediate files to transfer SQL queries to the MySQL command line. The results should then be redirected to an intermediate file and parsed by the script. This is much the same as using a wrench as a hammer. PHP provides a wide range of reliable tools for working with various databases and other technologies.
The scenario below involves backing up all non-system MySQL databases to SQL dump files and then compressing them. These files are only kept for 2 weeks and each file is deleted after the expiration date. PHP provides built-in support for all of these things.
Consider the code below showing how to work with MySQL databases in PHP:
<?PHP
define ("DB_TYPE", "MYSQLI");
define ("DB_HOST", "localhost");
define ("DB_NAME", "mysql");
define ("DB_USER", "nonadmin_readonly");
define ("DB_PASS", "XXXXXX");
// Get the database list.
$commands = array();
$conn = "";
if ($conn = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME))
{
$x = 0;
$sql0 = "show databases;";
$rs0 = $conn->query($sql0);
while ($row0 = $rs0->fetch_array(MYSQLI_BOTH))
{
$dbName = trim ($row0[0]);
// Exclude system dbs.
if ( ("mysql" != $dbName) && ("information_schema" != $dbName) &&
("test" != $dbName ) && ("performance_schema" != $dbName))
{
//print "Found [" . $dbName . "]n";
$dateStr = date ("Y-m-d");
//print $dateStr . "n";
$cmd = "/usr/bin/mysqldump --user=" . DB_USER. " --password="
. DB_PASS . " " . $dbName . " > /home/dba/database_backups/"
. $dateStr . "_" . $dbName . ".sql";
//print $cmd . "n";
$commands[$x] = $cmd;
$x++;
$commands[$x] =
"/usr/bin/bzip2 --force /home/dba/database_backups/"
. $dateStr . "_" . $dbName . ".sql";
$x++;
}
}
$conn->close();
}
for ($x = 0; $x < count($commands); $x++)
{
print $commands[$x] . "n";
if (1 == $x % 2)
print "n";
//system ($item);
}
// DELETION CODE GOES HERE.
?>
Using relatively simple PHP commands, you can generate timestamped filenames and compress the resulting files. This example runs on Linux and assumes the home directory is / home / dba… However, this code can be easily adapted for execution on Windows, since the MySQL syntax is the same, and bzip2 replace with a compression tool suitable for Windows.
This PHP code example generates the following output:
$ php backup_databases_native.php
/usr/bin/mysqldump --user=nonadmin_readonly --password=XXXXXX wordpress_db1 > ↩︎ /home/dba/database_backups/2021-10-21_wordpress_db1.sql
/usr/bin/bzip2 --force /home/dba/database_backups/2021-10-21_wordpress_db1.sql
/usr/bin/mysqldump --user=nonadmin_readonly --password=XXXXXX wordpress_db2_blog > ↩︎ /home/dba/database_backups/2021-10-21_wordpress_db2.sql
/usr/bin/bzip2 --force /home/dba/database_backups/2021-10-21_wordpress_db2.sql
/usr/bin/mysqldump --user=nonadmin_readonly --password=XXXXXX app_db > ↩︎ /home/dba/database_backups/2021-10-21_app_db.sql
/usr/bin/bzip2 --force /home/dba/database_backups/2021-10-21_app_db.sql
/usr/bin/mysqldump --user=nonadmin_readonly --password=XXXXXX udc1_db > ↩︎ /home/dba/database_backups/2021-10-21_udc1_db.sql
/usr/bin/bzip2 --force /home/dba/database_backups/2021-10-21_udc1_db.sql
Note: ↩︎ indicates that the command continues on the current line, but has been carried over for display convenience.
In general, it is recommended that you use the full path to every external command that will be executed. The reason is that if this code is executed in crontab Linux or as a scheduled Windows task, the system file path may not be available. Using the full path ensures that there are no errors “command not found”. Likewise, using the full path to the target directory in the commands themselves ensures that the generated files are saved in the correct directories.
Finally, add the functionality to delete old backup files, as shown below:
<?PHP
// .. Previous listing code goes here.
// Delete files older than 2 weeks.
$dir = "/home/dba/database_backups";
$maxAge = 14 * 86400;
if ($dh = opendir ($dir))
{
$currentTime = time();
while (($file = readdir($dh)) !== false)
{
if (("." != $file) && (".." != $file))
{
$fullPath = $dir . "/" . $file;
$age = $currentTime - filemtime($fullPath);
echo "filename: " . $fullPath . " - Age is [" . $age
. "] seconds. Max age is [" . $maxAge . "] seconds.n";
if ($age > $maxAge)
{
print "MUST DELETE [" . $fullPath . "]nn";
unlink ($fullPath);
}
else
print "Keep it.nn";
}
}
closedir ($dh);
}
?>
The presented code is not something special in terms of PHP capabilities. This listing is an adaptation of a long-standing PHP functionality.
One of the team bonuses print in the given code it is that if the code is executed in crontab and the parameter is set MAILTO, the output will show up in the emails generated by crontab…
Read also: How to Manage Linux Groups Using Command Line.
PHP code for backing up a database for sysadmins
This simple example of an existing database backup and cleanup process done with PHP is the tip of the iceberg of what PHP can do for a sysadmin. An additional advantage of using PHP is that an administrator who is fluent in PHP does not need to learn different scripting languages for specific operating systems. The use of scripting languages also complicates porting code between the command line and the web.