Adding ads to the mobile app. AdMob for Beginners
Introduction
The monetization policy is one of the most important components of a mobile application. It also comes to the point that the developers first think over the marketing scheme, and only then pull the gameplay on it. Now there are many proven ways to increase the ARPU (Average Revenue Per User) of your game: loot boxes, battle passes, direct sales of upgrades or cosmetic items. In this article, we will look at the simplest, oldest, but no less effective way to make money from a mobile application – in-game advertising, and also talk about how to implement it in Unity using Google AdMob as an example.
What to choose for a beginner? Unity Ads vs AdMob
Unity Ads and AdMob (Google) are two of the most famous advertising services on the market. For each, there are a ton of resources on the Internet, but with which one should you take the first step?
If you read the title of the article (and you read it), it should be obvious to you who my choice fell on. There are several reasons why I advise you to choose a solution from Google:
This service has higher CPC(cost-per-click);
Experience with third-party APIs;
I think you will still have to create an AdMob account, so why not do it now;
We connect AdMob
YouTube has a lot of material on the topic of connecting the service. Personally I have used this video, as well as official documentation. Below is a step-by-step instruction in text format with pictures.
Step by step installation looks like this:
Signing up for AdMob
Create a project and set up blocks (each of the blocks will be discussed below)
Download from github official plugin
Import into Unity (assets – import package)
We prescribe in assets – Google Mobile Ads – Settings ID of your application. It lies on the application page in AdMob: Application settings – Application ID

We initialize advertising
using GoogleMobileAds.Api;
public class AdInitialize : MonoBehaviour
{
private void Awake()
{
MobileAds.Initialize(initStatus => { });
}
}
We create classes of necessary advertising blocks (we will consider below).
Ad blocks
Now let’s discuss what types of ad units AdMob provides us with. In order to create a block, the first thing you need to do is select its type. Here is the list on the site:

We’ll look at each option below. documentation there is also the required code (Ad Formats menu on the left).
IMPORTANT: In the scripts below, instead of the ID of your blocks at the testing stage, you need to use the test IDs that lie here. Otherwise, your application will be banned. My code uses test IDs everywhere.
Banner
A rectangular block that will constantly hang on the screen. Can update automatically. The update frequency can be set manually (Advanced settings – Automatic update – Special). For the call, we use the BannerView class. The constructor specifies the size and position on the screen (in enum format). Rendered automatically when the scene is loaded.
using GoogleMobileAds.Api;
public class BannerAd : MonoBehaviour
{
private BannerView _bannerAd;
private const string _bannerId = "ca-app-pub-3940256099942544/6300978111";
private void OnEnable()
{
_bannerAd = new BannerView(_bannerId, AdSize.Banner, AdPosition.Top);
AdRequest adRequest = new AdRequest.Builder().Build();
_bannerAd.LoadAd(adRequest);
}
public void ShowAd()
{
_bannerAd.Show();
}
}
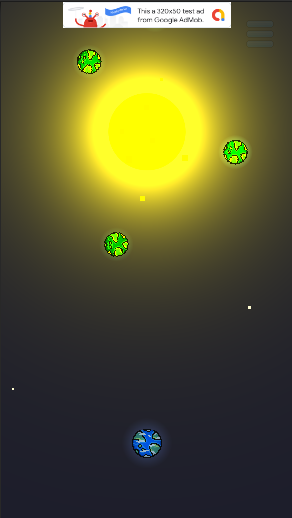
Interstitial ad
Standard full-screen ads. Called by the Show() method. During the show, pauses the game.
using GoogleMobileAds.Api;
public class InterAd : MonoBehaviour
{
private InterstitialAd _interAd;
private const string _interstitialId = "ca-app-pub-3940256099942544/8691691433";
private void OnEnable()
{
_interAd = new InterstitialAd(_interstitialId);
AdRequest adRequest = new AdRequest.Builder().Build();
_interAd.LoadAd(adRequest);
}
public void ShowAd()
{
if (_interAd.IsLoaded())
{
_interAd.Show();
}
}
}

Rewarded interstitial ad
Advertising of the previous format with the only difference – after viewing the callback, you can accrue a reward to the user.
public class RewardedInterAD : MonoBehaviour
{
private RewardedInterstitialAd _rewardAd;
private const string _interstitialId = "ca-app-pub-3940256099942544/5354046379";
private void OnEnable()
{
AdRequest adRequest = new AdRequest.Builder().Build();
RewardedInterstitialAd.LoadAd(_interstitialId, adRequest, adLoadCallback);
}
private void adLoadCallback(RewardedInterstitialAd ad, AdFailedToLoadEventArgs error)
{
if (error == null)
{
_rewardAd = ad;
}
}
public void ShowAd()
{
if (_rewardAd != null)
{
_rewardAd.Show(userEarnedRewardCallback);
}
}
private void userEarnedRewardCallback(Reward reward)
{
// Начисляем награду
}
}

With reward
Almost the previous option, only users must agree to view.
public class RewardAd : MonoBehaviour
{
private RewardedAd _rewardAd;
private const string _adId = "ca-app-pub-3940256099942544/5224354917";
private void OnEnable()
{
_rewardAd = new RewardedAd(_adId);
AdRequest adRequest = new AdRequest.Builder().Build();
_rewardAd.LoadAd(adRequest);
_rewardAd.OnUserEarnedReward += HandleUserEarnedReward;
}
public void ShowAd()
{
if (_rewardAd.IsLoaded())
{
_rewardAd.Show();
}
}
private void HandleUserEarnedReward(object sender, Reward args)
{
// Начисляем награду
}
}
Extended native
Ads embedded directly into the game scene. Piece by piece we collect ads using various Get-methods. I advise you to look YouTube guide to quickly understand the embedding process and read the API here.
The end result looks like this:
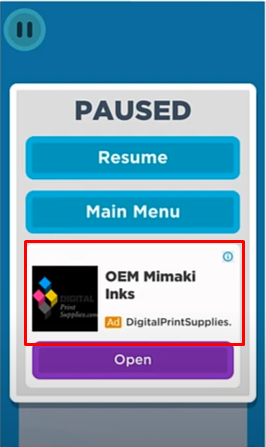
Application launch
An ad that appears before the app launches. The application will run in parallel, so this format can be used instead of a heavy scene loading screen. We take all the code from this pages.
The end result looks like this:
public class AppOpenAdManager
{
private const string AD_UNIT_ID = "ca-app-pub-3940256099942544/3419835294";
private static AppOpenAdManager instance;
private AppOpenAd ad;
private bool isShowingAd = false;
public static AppOpenAdManager Instance
{
get
{
if (instance == null)
{
instance = new AppOpenAdManager();
}
return instance;
}
}
private bool IsAdAvailable
{
get
{
return ad != null;
}
}
public void LoadAd()
{
AdRequest request = new AdRequest.Builder().Build();
// Load an app open ad for portrait orientation
AppOpenAd.LoadAd(AD_UNIT_ID, ScreenOrientation.Portrait, request, ((appOpenAd, error) =>
{
if (error != null)
{
// Handle the error.
Debug.LogFormat("Failed to load the ad. (reason: {0})", error.LoadAdError.GetMessage());
return;
}
// App open ad is loaded.
ad = appOpenAd;
}));
}
public void ShowAdIfAvailable()
{
if (!IsAdAvailable || isShowingAd)
{
return;
}
ad.OnAdDidDismissFullScreenContent += HandleAdDidDismissFullScreenContent;
ad.OnAdFailedToPresentFullScreenContent += HandleAdFailedToPresentFullScreenContent;
ad.OnAdDidPresentFullScreenContent += HandleAdDidPresentFullScreenContent;
ad.OnAdDidRecordImpression += HandleAdDidRecordImpression;
ad.OnPaidEvent += HandlePaidEvent;
ad.Show();
}
private void HandleAdDidDismissFullScreenContent(object sender, EventArgs args)
{
Debug.Log("Closed app open ad");
// Set the ad to null to indicate that AppOpenAdManager no longer has another ad to show.
ad = null;
isShowingAd = false;
LoadAd();
}
private void HandleAdFailedToPresentFullScreenContent(object sender, AdErrorEventArgs args)
{
Debug.LogFormat("Failed to present the ad (reason: {0})", args.AdError.GetMessage());
// Set the ad to null to indicate that AppOpenAdManager no longer has another ad to show.
ad = null;
LoadAd();
}
private void HandleAdDidPresentFullScreenContent(object sender, EventArgs args)
{
Debug.Log("Displayed app open ad");
isShowingAd = true;
}
private void HandleAdDidRecordImpression(object sender, EventArgs args)
{
Debug.Log("Recorded ad impression");
}
private void HandlePaidEvent(object sender, AdValueEventArgs args)
{
Debug.LogFormat("Received paid event. (currency: {0}, value: {1}",
args.AdValue.CurrencyCode, args.AdValue.Value);
}
}

Afterword
In reality, the world is not divided into Unity Ads and AdMob, but you need to approach the choice of a network based on your target audience. You can expand your horizons about the existing options by reading this article. Also, usually no one uses one service when mediation exists – an automatic choice of a solution that will pay more in a particular situation. Traditionally, I refer you to separate sourceif you are interested in this topic.
I wish you creative and commercial success!