How to Set Up a Node.js Express Server for React
React is a JavaScript library for developing user interfaces. It allows you to create efficient and scalable web applications based on component architecture.
Express.js is a minimalistic and flexible web framework for Node.js that makes it easy to develop web applications and APIs. It provides a simple interface and feature set to quickly create servers and route requests.
Introduction
This guide will help you develop a simple React application and connect it to a server built using Node.js. We’ll start by creating a React app with the command create-react-app
and then set up its connection to the Node.js server with proxy
.
The necessary conditions
To successfully complete this guide, it will be useful to have the following:
Prior experience with Node.js, Express, npm and React.js.
Installed Node.js.
Text editor, preferably VS Code.
A web browser, in this case Google Chrome.
Setting up the folder structure

The first step is to create a root folder for our application named express-react-app
, which will contain all the application files. We will then create a folder client
which will contain all the React application files.
Folder node_modules
will contain all NPM packages for the file server.js
. Folder node_modules
will be automatically created when installing NPM packages.
Next, we need to create a file server.js
. This file will host the Express server, which will act as our backend. File package.json
will be automatically generated when the command is executed in the terminal npm init -y
.
Creating a React App
From the terminal, navigate to the root directory with the command cd
and run the following commands:
$cd express-react-app
$npx create-react-app client
The above commands will create a React app called client
inside the root directory.
Express server setup
The next step is to create an Express server in a file server.js
.
From the terminal, go to the root directory and run the following command:
$npm init -y
The command will automatically generate the file package.json
. We will then need to run the following command to install Express and it will be saved as a dependency in the file package.json
.
$npm install express --save
Now edit the file server.js
in the following way:
const express = require('express'); //Строка 1
const app = express(); //Строка 2
const port = process.env.PORT || 5000; //Строка 3
// Сообщение о том, что сервер запущен и прослушивает указанный порт
app.listen(port, () => console.log(`Listening on port ${port}`)); //Строка 6
// Создание GET маршрута
app.get('/express_backend', (req, res) => { //Строка 9
res.send({ express: 'YOUR EXPRESS BACKEND IS CONNECTED TO REACT' }); //Строка 10
}); //Строка 11
Lines 1 and 2 – include the Express module and allow you to use it inside the file server.js
.
Line 3 – Set the port on which the Express server will run.
Line 6 – A message will be displayed in the console stating that the server is working properly.
Line 9 and 11 set up the GET route that we will later get from our React client application.
Proxy setting
In this step, the Webpack Development Server was automatically generated when the command was run create-react-app
. Our React app is powered by the Webpack Development Server front-end.
The Webpack Development Server (WDS) is a tool that helps developers make changes to the front end of a web application and automatically renders those changes in the browser without the need for a page refresh.
It is unique compared to other tools that do the same because the contents of the package are not written to disk as files, but stored in memory. This advantage is extremely important when debugging code and styles.
We can set up proxying API requests from the client side to the API on the server side. The API on the server side (Express server) will run on port 5000.
First set up a proxy to navigate to the directory client
and find the file package.json
. Add the following line to it.
“proxy”: “http://localhost:5000”
Modified file package.json
will look like this:
{
"name": "client",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.16.5",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
"proxy": "http://localhost:5000"
}
Modified file package.json
will allow Webpack to proxy API requests to the Express backend server running on port 5000.
Calling an Express backend server from React
First go to the folder client/src
and edit the file App.js
so that it looks like this:
import React, { useEffect, useState } from 'react';
import logo from './logo.svg';
import './App.css';
function App() {
const [state, setState] = useState(null);
const callBackendAPI = async () => {
const response = await fetch('/express_backend');
const body = await response.json();
if (response.status !== 200) {
throw Error(body.message)
}
return body;
};
// получение GET маршрута с сервера Express, который соответствует GET из server.js
useEffect(() => {
callBackendAPI()
.then(res => setState(res.express))
.catch(err => console.log(err));
}, [])
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
{/* вывод данных, полученных с сервера Express */}
<div>
{state}
</div>
</div>
);
}
export default App;
Inside the hook useEffect()
function is called callBackendAPI()
. This function will fetch data from a previously created route on the Express server. When receiving a response from a request fetch
meaning res.express
is set to the state state
using the function setState()
. Then the value state
displayed inside the element <div>
to display on the page.
Application launch
To run the application, go to the root directory express-react-app
and run the following command:
$cd express-react-app
$node server.js
After running the file server.js
the next step is to navigate to the web browser at “http://localhost:5000/express_backend” and the following message will be displayed:
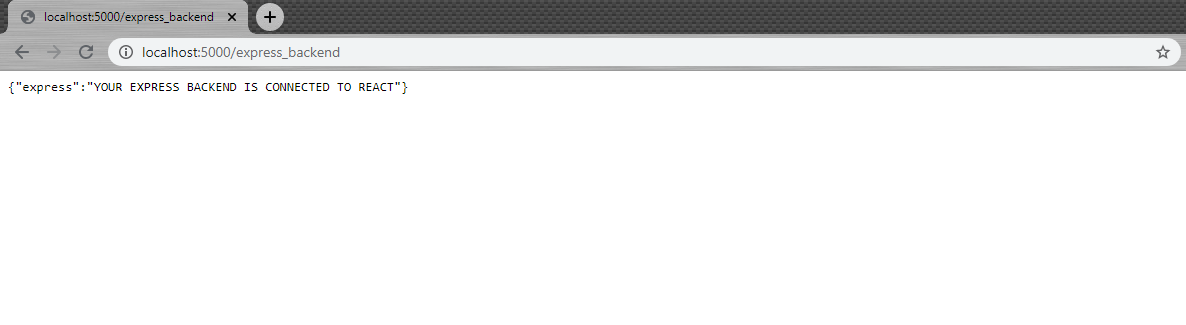
The above demonstrates that our Express server is working properly and the GET route we created is functioning, and it is also possible to get this route from the client side.
Also note that the URL path is the same as the path we specified in our GET route in the file server.js
.
Then go to directory client
in terminal and run the following commands:
$cd client
$npm start
The above commands will start the React development server which runs on port 3000 and will automatically open in a web browser.
The following message will be displayed on the screen:
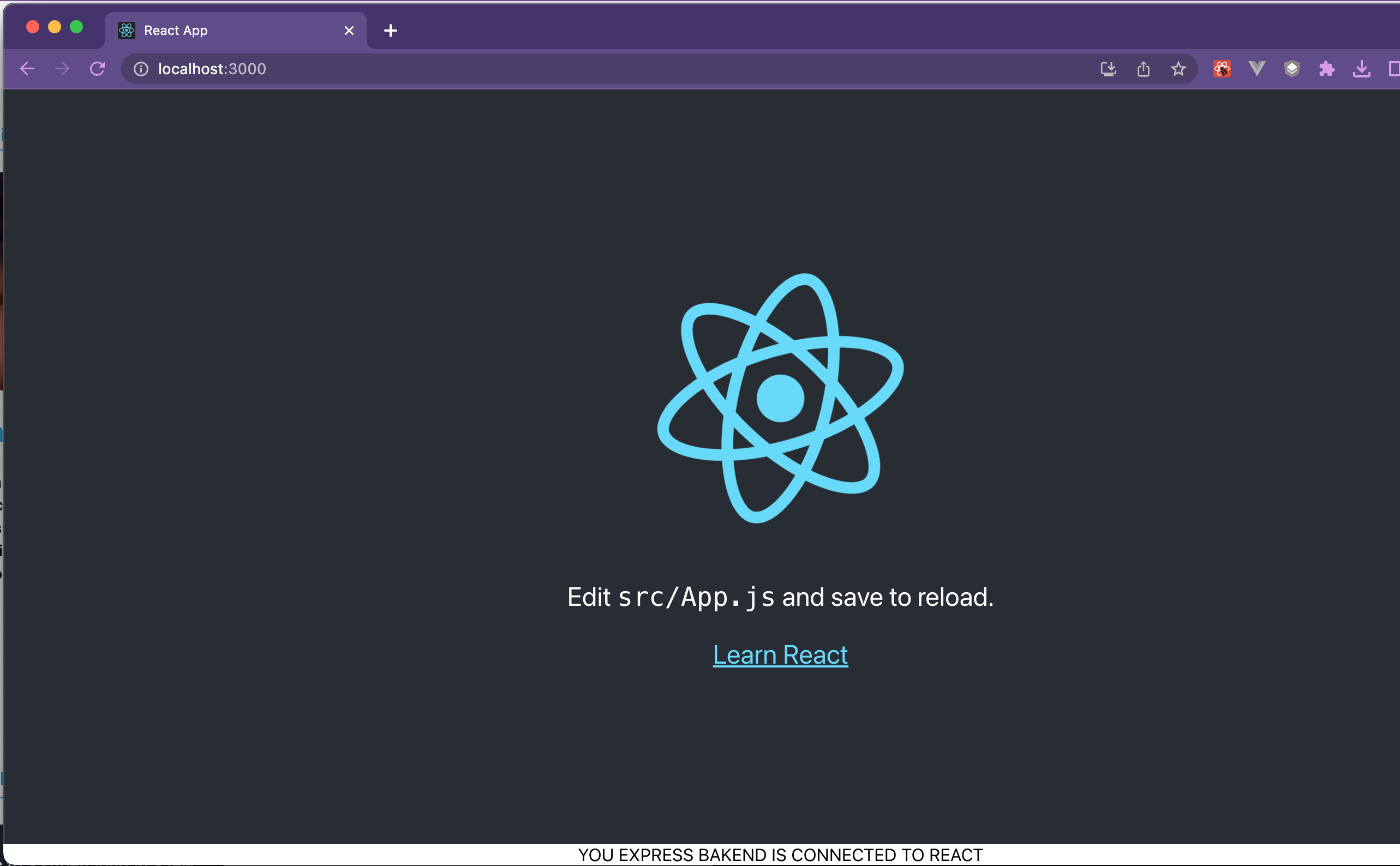
Finally, we have displayed the data received from the GET route in server.js
in our front-end React app, as shown above.
If the Express server is down, the React server will still continue to run. However, the connection to the backend will be lost and nothing will be displayed.
Conclusion
You can do a lot with the Express server, like making calls to a database. However, in this tutorial, we focused on how to quickly connect a React client application to an Express server on the backend side.
The code snippets and files used in this tutorial can be found in the repository GitHub at this link.
Good luck with your project!