Data Visualization with Python Dash
Using the data visualization interface, also known as creating dashboards, is an integral part of the data analyst skill set. Dashboards and data apps are everywhere these days, from presenting analysis results with a range of visuals to showcasing your machine learning applications.
If it’s about data, then it’s about Python too. In particular, we are talking about the Dash library, which is built on top of one of the most popular charting libraries – Plotly.
Dash makes it easy to create and share data analysis results with interactive dashboards using only Python code. No need to learn HTML, CSS, or complex JavaScript frameworks like React.js.
In this guide, you’ll get an idea of what Dash is capable of and how to integrate it into your workflow.
Installing Dash and Plotly
You can install Dash with pip
. You also need to install the pandas library to work with datasets:
pip install dash pandas
The above command also installs plotly
. Plotly is known for its interactive charts, and Plotly and Dash are from the Plotly Software Foundation, so the libraries work quite well together.
Requirements for using Dash
A powerful framework like Dash has several requirements. First, you need to know Plotly Python as Dash can only display interactive Plotly plots.
Next, you need a basic understanding of HTML and CSS. Dash is similar to React but in Python. This is a template framework where you can create a website without JavaScript.
The dashboard contains a lot of graphics, and it is up to the user to decide how to display everything on one page. Plotly handles images, but the layout aspect depends on Dash and its HTML components.
Creating a Dash Application
Let’s create our Dash application. After installation, import the following libraries:
import dash
import dash_core_components as dcc
import dash_html_components as html
import plotly.express as px
import pandas as pd
dash
is a global library containing all the main functions. dash_core_components
And dash_html_components
are libraries that are installed with dash
default. They include Dash-specific features and a Python representation of HTML components (tags). More on them later.
Any Dash application is launched with the following command:
app = dash.Dash(name="my_first_dash_app")
>>> app
<dash.dash.Dash at 0x1ee6af51af0>
The above code completely creates a boilerplate for a clean website. We don’t need a blank page, so let’s fill it up.
First, we will load the embedded dataset from Plotly and create a simple scatterplot:
# Load dataset using Plotly
tips = px.data.tips()
fig = px.scatter(tips, x="total_bill", y="tip") # Create a scatterplot
We then add this image to the attribute layout
our application inside the tag div
with small texts:
app.layout = html.Div(children=[
html.H1(children='Hello Dash'), # Create a title with H1 tag
html.Div(children='''
Dash: A web application framework for your data.
'''), # Display some text
dcc.Graph(
id='example-graph',
figure=fig
) # Display the Plotly figure
])
if __name__ == '__main__':
app.run_server(debug=True) # Run the Dash app
Now we create HTML tags using the library dash_html_components (html)
and the image using the main components (dcc) library
.
Behind the title tag H1
should div
containing plain text, then the graph itself using the library’s Graph function dcc
. All this is inside the attribute children
one div tag.
At the end, we also add a command that runs our application in debug mode, meaning changes take effect as changes are made to the script. Here is the complete code for now:
import dash
import dash_core_components as dcc
import dash_html_components as html
import plotly.express as px
import pandas as pd
# Create the app
app = dash.Dash(__name__)
# Load dataset using Plotly
tips = px.data.tips()
fig = px.scatter(tips, x="total_bill", y="tip") # Create a scatterplot
app.layout = html.Div(children=[
html.H1(children='Hello Dash'), # Create a title with H1 tag
html.Div(children='''
Dash: A web application framework for your data.
'''), # Display some text
dcc.Graph(
id='example-graph',
figure=fig
) # Display the Plotly figure
])
if __name__ == '__main__':
app.run_server(debug=True) # Run the Dash app
Put it in a python script and run it. In the terminal, you will receive a message that you need to follow this link: http://127.0.0.1:8050/.
So let’s go:

In the following sections, we will detail what happened here.
Create app.layout
Let’s start with the attribute layout
. This is the only attribute that contains all of your HTML components and images. You must pass all your graphics and HTML tags to it in the final DIV tag.
Depending on the size of your project, this attribute can get quite large, so I recommend creating all your HTML tags and shapes in separate variables and then passing them to layout
.
For example, here’s what the above application would look like:
app = dash.Dash(name="app")
# Load dataset using Plotly
tips = px.data.tips()
fig = px.scatter(tips, x="total_bill", y="tip") # Create a scatterplot
title = html.H1("Hello Dash!")
text_div = html.Div("Dash: A web application framework for your data.")
graph_to_display = dcc.Graph(id="scatter", figure=fig)
app.layout = html.Div(children=[title, text_div, graph_to_display])
It’s much neater and more compact, and it’s one of the things you don’t learn about in the Dash documentation. There’s a bunch of nested code instead of doing the above.
HTML and CSS components in Dash
Let’s discuss how HTML and CSS work in Dash. Sublibrary dash_html_components
contains the most common HTML tags such as divs, buttons, text fields, titles, heading tags (H1-6), etc.
They are implemented in Python code under appropriate names as a representation of their HTML counterparts. So, code like the one below:
import dash_html_components as html
html.Div([
html.H1('Hello Dash'),
html.Div([
html.P('Dash converts Python classes into HTML'),
html.P("This conversion happens behind the scenes by Dash's JavaScript frontend")
])
])
Will be interpreted by your browser as follows:
<div>
<h1>Hello Dash</h1>
<div>
<p>Dash converts Python classes into HTML</p>
<p>This conversion happens behind the scenes by Dash's JavaScript front-end</p>
</div>
</div>
All HTML tags in this sublibrary contain the following main arguments:
id
: same as attributeid
HTML tagsclassName
: same as attributeclass
HTML tagsstyle
: same as attributestyle
HTML tags, but only accepts a CSS style dictionarychildren
: the first argument of most HTML components
Here is an example div
with some custom settings:
app = dash.Dash(name="app")
app.layout = html.Div(
children=html.H3("Simple Div"),
id="sample_div",
className="red_div",
style={"backgroundColor": "red"},
)
if __name__ == "__main__":
app.run_server(debug=True)

Argument children
is unique – it can accept numbers and strings, but more often than not, you’ll pass other HTML components as a list if that’s tag-containersuch as div.
A note about CSS styles: Most CSS style attributes use hyphens to separate words. When you pass them into an argument style Dash
they must apply camelCase
For example backgroundColor
instead of background-color
.
I highly recommend that you look into these HTML tags, as they are the only thing that keeps the layout of your application intact. Here full list HTML tags supported by Dash.
Key Components of Dash
Another important part of Dash is its main components. Library dash_core_components
contains a few other HTML tags, but has some CSS and JavaScript already embedded in it.
Some examples include dropdowns, sliders, upload and download functionality, and a Plotly plotting component.
Here are a few samples of these components, starting with a dropdown list:
import dash
import dash_core_components as dcc
import dash_html_components as html
app = dash.Dash(__name__)
app.layout = html.Div([
dcc.Dropdown(
options=[
{'label': 'FC Barcelona', 'value': 'FCB'},
{'label': 'Real Madrid', 'value': 'RM'},
{'label': 'Manchester United', 'value': 'MU'}
],
value="FCB" # The default value to display
)
])

Multi-select dropdown list:
app = dash.Dash(__name__)
app.layout = html.Div([
dcc.Dropdown(
options=[
{'label': 'FC Barcelona', 'value': 'FCB'},
{'label': 'Real Madrid', 'value': 'RM'},
{'label': 'Manchester United', 'value': 'MU'}
],
multi=True,
value="FCB"
)
], style={"width": 200})
if __name__ == '__main__':
app.run_server(debug=True)

Slider with breakpoints marked:
import dash
import dash_core_components as dcc
import dash_html_components as html
app = dash.Dash(__name__)
app.layout = html.Div([
dcc.Slider(
min=0,
max=9,
marks={i: 'Label{}'.format(i) for i in range(10)},
value=5,
)
])
if __name__ == '__main__':
app.run_server(debug=True)
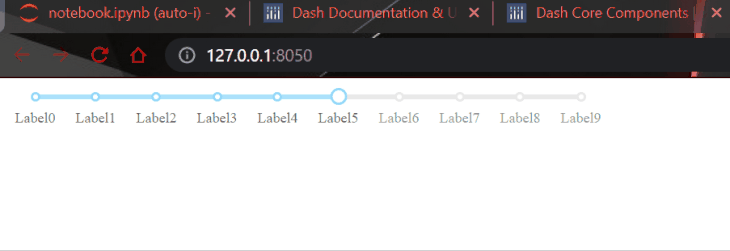
There is a generic value attribute that represents the default value when the component is first rendered.
See the full list of main components Here.
Creating the final data visualization interface with Python Dash
As a final example, take a look at the application below:
import seaborn as sns
app = dash.Dash(__name__)
diamonds = sns.load_dataset("diamonds")
scatter = px.scatter(
data_frame=diamonds,
x="price",
y="carat",
color="cut",
title="Carat vs. Price of Diamonds",
width=600,
height=400,
)
histogram = px.histogram(
data_frame=diamonds,
x="price",
title="Histogram of Diamond prices",
width=600,
height=400,
)
violin = px.violin(
data_frame=diamonds,
x="cut",
y="price",
title="Violin Plot of Cut vs. Price",
width=600,
height=400,
)
left_fig = html.Div(children=dcc.Graph(figure=scatter))
right_fig = html.Div(children=dcc.Graph(figure=histogram))
upper_div = html.Div([left_fig, right_fig], style={"display": "flex"})
central_div = html.Div(
children=dcc.Graph(figure=violin),
style={"display": "flex", "justify-content": "center"},
)
app.layout = html.Div([upper_div, central_div])
if __name__ == "__main__":
app.run_server(debug=True)
We import the Diamonds dataset from Seaborn and create three graphs: scatterplot, histogram and violin diagram. We want to display the scatterplot and the histogram next to each other and place the violin chart right below them in the center.
To do this, we create two divs containing a scatterplot and a histogram, left_figure
And right_figure
. Then, for simplicity, these two divs are placed in another div – upper_div
.
We’re setting the flex-box CSS style for this div, which lays out the shapes side-by-side.
We then create a central div containing the violin chart and center align it with flex-box and its justify-content attribute.
Finally, we place everything in a layout inside the last DIV and run the script. Here is the end result:

Conclusion
Here is a summary of the steps to create a basic Dash application:
Create an app with
dash.Dash
and give it any name.Sketch out the layout of your charts in the dashboard before writing the code.
Create charts for your dashboard
Create a Template Layout with Dash HTML Components
Add your images to the appropriate containers
Finally, add all the HTML components to the layout attribute
While we’ve covered many of the basics such as HTML, core components, and application layout, this is only a superficial introduction to Dash’s capabilities.
I showed you many examples of interactive HTML components, but didn’t tell you how to integrate them into your application. How can you update graphs based on user input such as sliders, text, or something similar?
This is where callbacks come to the rescue. This is a very powerful and core feature of Dash. To use callbacks, you define functions in Dash that fire when the user changes the component, and the function updates the new component based on that event.
Dash dedicates a large section of the documentation just to explaining callbacks, as they are difficult to understand at first. I suggest you contact this topic at the next stage.
Look into gallery of examples, where you can see and enjoy some very cool projects created with Dash. The best part is that many of them are open source, which means that you can learn a lot for yourself and be inspired by new ideas.
Thanks for reading!
In conclusion, we invite you to an open lesson “Working with Power BI. Building a dashboard. Customization and interaction of visual elements. In the lesson, we will load data into Power BI Desktop from the source, build a small dashboard, look at the purpose and options for setting up basic visual elements. You can sign up link.