what you need to know about it to implement it in a project
At work, I was faced with the task of implementing the Privacy Manifest into a project. The study of the issue began with reading Apple documentation, and continued by watching the WWDC session on the topic of privacy. After implementation, it turned out that in order to work on the task correctly, it was necessary to study more information. Therefore, I decided to share my experience in the format of instructions. I'll show you how to implement the Privacy Manifest using a fictitious project as an example, and give practical advice on how to implement a privacy manifesto.
Purpose of implementation
Users are often interested in what categories of data applications collect about them. Is this information used to track them? Is there data associated with them? To promote transparency on this issue, Apple has created a Privacy Manifest to help define privacy rules within an app's dependencies and within the app itself.
It will now be easier to provide accurate Nutrition Labels and integrate app tracking transparency and give people control over how and when their data is used for tracking. And that's not it. Applications that reference the API and can potentially be used to fingerprinting (a practice banned in the App Store) will now be required to select a permitted reason for using the API and declare that use in a privacy manifest. This is done to provide additional protection to users. As part of this process, applications must accurately describe the use of these APIs, and their operation is only permitted in the cases described in the Manifest.
Privacy Manifest for third-party SDKs
Much of an app's privacy often depends on third-party SDKs. At the same time, it is very difficult to obtain from them all the necessary information on which our applications depend.
Please remember that when you use third-party SDKs in your application, we are responsible for all code that the SDK includes in the application. We need to know how data is collected and used.
Privacy manifests are a new way for third-party SDK developers to provide information about their privacy policies. They can also include the manifest into their SDK themselves.
The manifest file is a list of properties that specifies what types of data the SDK collects, how each is used, whether it is associated with the user, and whether it is used for tracking as defined by the tracking transparency policy. When developers prepare to publish an app, Xcode combines the privacy manifests of all third-party SDKs used in the app into a single, easy-to-use report. This will make it easier to create more accurate Privacy Nutrition Labels.
What are SDK signatures for?
Apple is trying to help developers improve the integrity of the software supply chain. When using third-party SDKs, it can be difficult for developers to understand that the code they downloaded was written by the developer they expect. To solve this problem, they introduced SDK signatures so that when a developer uses a new version of a third-party SDK in their app, Xcode can verify that it was signed by the same developer. This feature will be useful for both developers and users.
SDKs that require a privacy manifest and signature
Below are the most commonly used SDKs in apps on the App Store. Starting in Spring 2024, developers must include a privacy manifest for any SDK listed below. This rule works when you submit new apps to App Store Connect that contain these SDKs, or when you submit an app update that adds one of the listed SDKs as part of the update. Signatures are also required in cases where the listed SDKs are used as binary dependencies. Any version of the listed SDK, as well as all SDKs that repackage those listed, are included in the requirement.
Let's get started with the Privacy Manifest
SDK List
Abseil
AFNetworking
Alamofire
AppAuth
BoringSSL/openssl_grpc
Capacitor
Charts
connectivity_plus
Cordova
device_info_plus
DKImagePickerController
DKPhotoGallery
FBAEMKit
FBLPromises
FBSDKCoreKit
FBSDKCoreKit_Basics
FBSDKLoginKit
FBSDKShareKit
file_picker
FirebaseABTesting
FirebaseAuth
FirebaseCore
FirebaseCoreDiagnostics
FirebaseCoreExtension
FirebaseCoreInternal
FirebaseCrashlytics
FirebaseDynamicLinks
FirebaseFirestore
FirebaseInstallations
FirebaseMessaging
FirebaseRemoteConfig
Flutter
flutter_inappwebview
flutter_local_notifications
fluttertoast
FMDB
geolocator_apple
GoogleDataTransport
GoogleSignIn
GoogleToolboxForMac
GoogleUtilities
grpcpp
GTMAppAuth
GTMSessionFetcher
hermes
image_picker_ios
IQKeyboardManager
IQKeyboardManagerSwift
Kingfisher
leveldb
Lottie
MBProgressHUD
nanopb
OneSignal
OneSignalCore
OneSignalExtension
OneSignalOutcomes
OpenSSL
OrderedSet
package_info
package_info_plus
path_provider
path_provider_ios
Promises
Protobuf
Reachability
RealmSwift
RxCocoa
RxRelay
RxSwift
SDWebImage
share_plus
shared_preferences_ios
SnapKit
sqflite
Starscream
SVProgressHUD
SwiftyGif
SwiftyJSON
Toast
UnityFramework
url_launcher
url_launcher_ios
video_player_avfoundation
wakelock
webview_flutter_wkwebview
What should be done
In order for our application to comply with the requirements of the privacy manifesto, we need to solve two problems:
Create a Privacy Manifest for our project.
Update specific external dependencies (SDKs).
To create a Privacy Manifest, you need to understand what it is and how to add it to your project. To add to a project in Xcode, select Choose File > New File (⌘+N) and find a file called App Privacy.
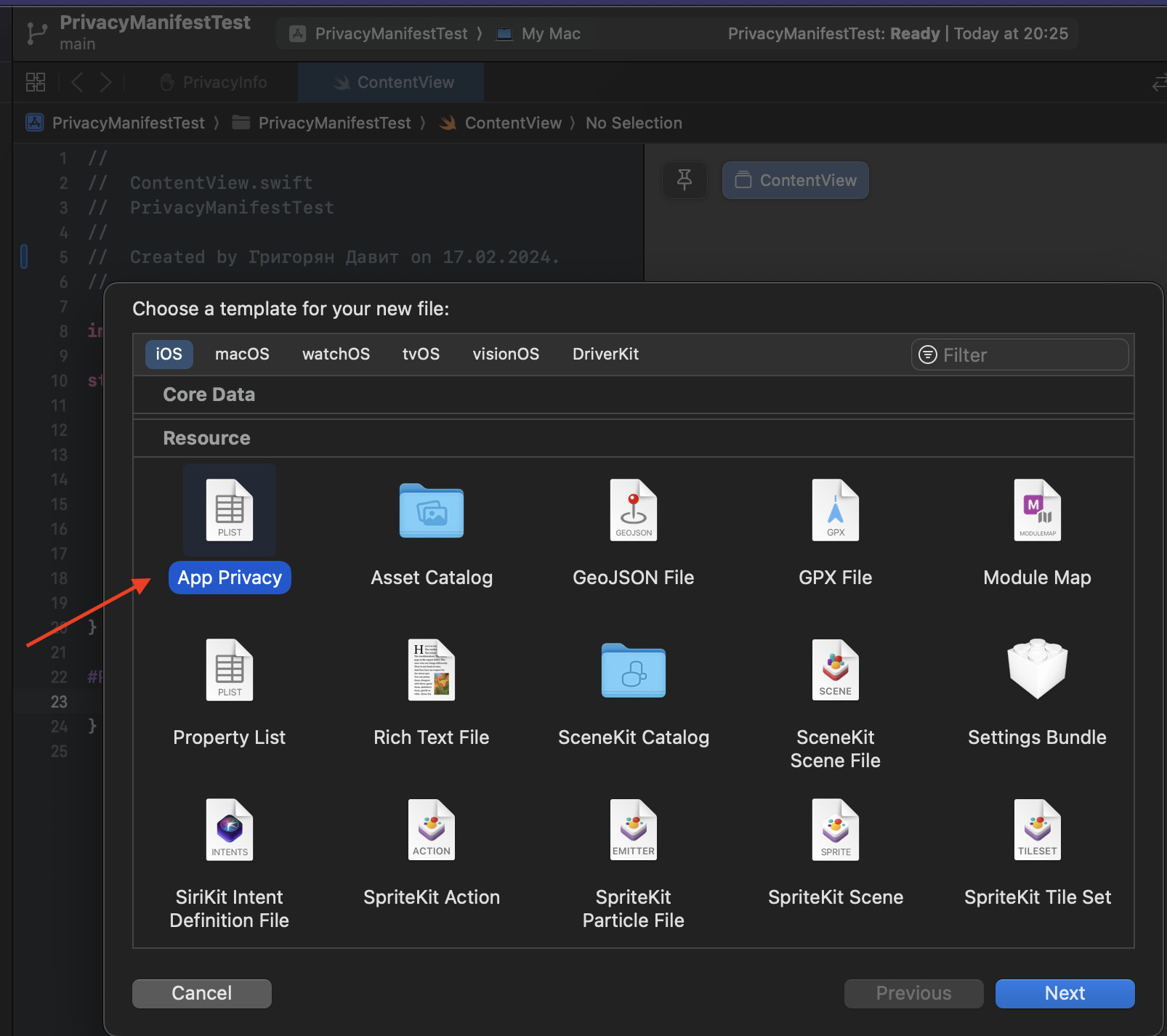
We add it to the root folder of the project with our application target enabled.
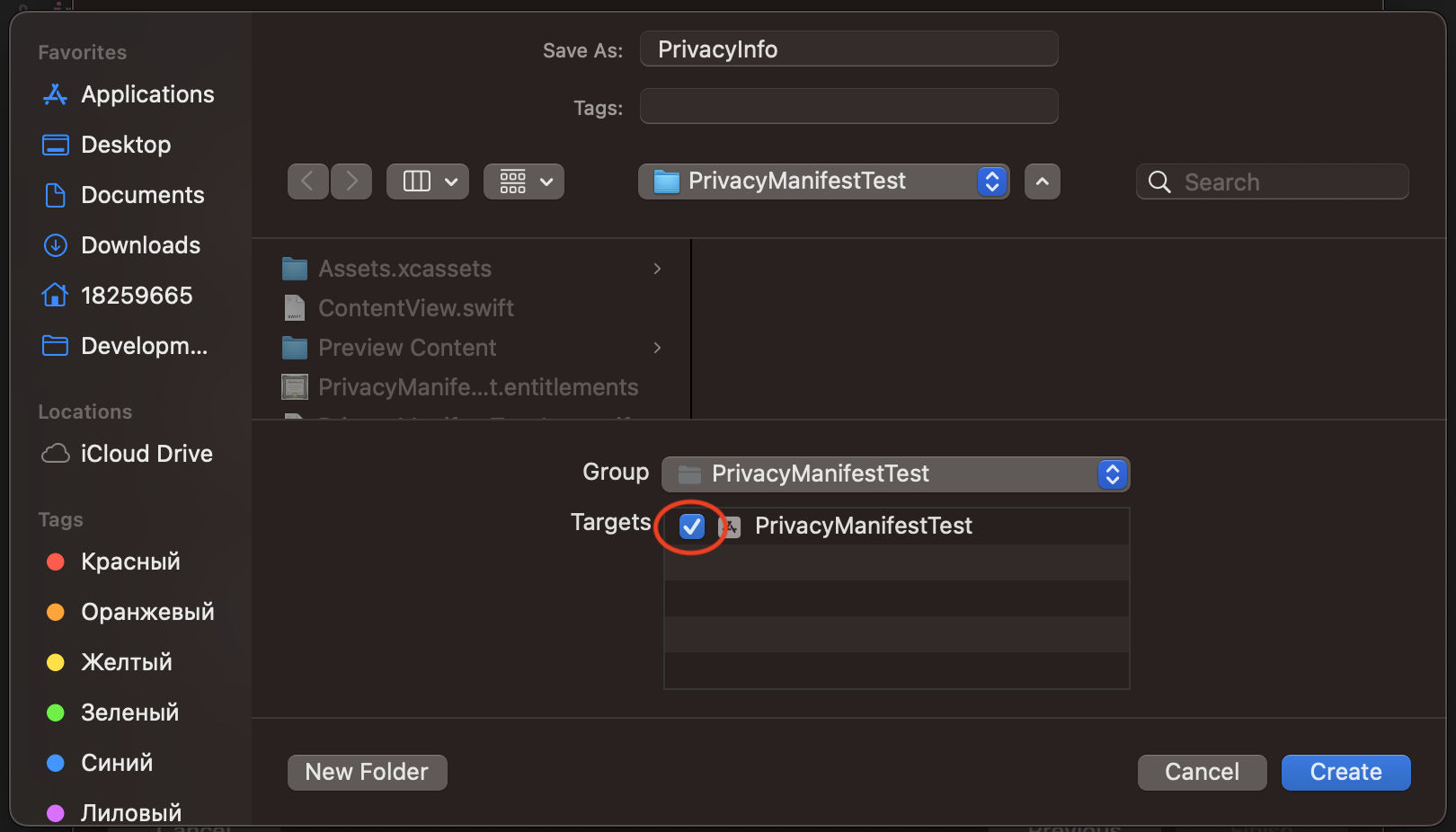
The manifesto in the project is called PrivacyInfo.

What is a manifest file?
Manifest file plist with extension .xcprivacy contains the following sections for filling in with data:
Privacy Tracking Enabled. A Boolean value indicating whether the application or third-party SDK uses tracking data as defined by the framework App Tracking Transparency. If your application has user tracking then you should provide a value YES. Let's assume we have it.
Privacy Tracking Domains. An array of strings that lists the Internet domains that the app or third-party SDK connects to and participates in tracking. If for NSPrivacyTracking (Privacy Tracking Enabled) set value YESthen you must specify at least one Internet domain.
Let's say we're tracking a user by domain tracking.domain.example and specified it in the manifest file. Apple's documentation states that if a user has not granted tracking permission through the App Tracking Transparency framework, then network requests to those domains fail and the app receives an error. What does this mean? As of iOS 14.5, there is a mandatory requirement to request user permission in cases of data tracking. In these scenarios, when we log in, we see a notification like this:

If the user has iOS 17.0 or higher and has selected Ask App Not to Track, then requests to our domain tracking.domain.example will not be executed and we will receive an error. This scenario was reproduced on an iOS 17.2 device, but the error did not occur, and requests to the tracking domain also went through. We hope that in the future everything will work as Apple says. But how do we understand that no one else (for example, the SDK included in our application) is tracking the user and our domain is the only one? To do this, Apple offers to analyze the network behavior of the application using the tool Points of Interest.
Necessary:
Open the application in Xcode.
Connect the device, since network behavior cannot be tested on the simulator.
Select Product > Profile, it will open Instruments, there select the Network template.
Select Xcode > Open Developer Tool > Instruments and there select the Network template.
Then you need to click on the entry and go to the lower tool called Points of Interest. In recording mode, you can navigate through the entire application on your device. The moment the domain is accessed, a dot with details about it will appear on the Points of Interest track.

This way, you can identify hidden requests to tracking domains and eliminate them, or specify their use in the manifest.
Unfortunately, this tool also does not work perfectly. I added a second tracking domain to my application, but I was unable to determine either the primary domain or the secondary one. There are currently open bugs on the Apple forum regarding incorrect operation Points of Interest.

The Privacy Manifest with the Privacy Tracking Enabled and Privacy Tracking Domains data filled in looks like this.
Privacy Nutrition Label Types. An array of dictionaries that describes the types of data that the application or third-party SDK collects. Nutrition Labels are needed so that users can find out exactly what data it collects before downloading an application from the App Store. In the App Store, the specified data looks like this:

It's worth noting that Nutrition Labels are not new, and if your app is on the App Store, then you've already filled out a form in App Store Connect indicating the data your app collects. Now you just need to rewrite the information from App Store Connect into the manifest. And if you are just going to release an application for the first time, then you need to decide on the data that it collects. They are divided into the following categories:
Contact Information.
Health and fitness.
Financial information.
Geolocation.
Sensitive information.
Contacts.
User Content.
Browser history.
Search history.
Identifiers.
Purchases.
Usage data.
Diagnostics.
Environment.
Body.
Other data.
Let's assume our application collects the following data:


And now we need to transfer all this to the manifest. To populate Nutrition Label types, the following dictionary keys exist:
NSPrivacyCollectedDataType
. A string that identifies the type of data that the application or third-party SDK collects.NSPrivacyCollectedDataTypeLinked
. A Boolean value indicating whether the application or third-party SDK associates this data type with the user's identity.NSPrivacyCollectedDataTypeTracking
. A Boolean value indicating whether the application or third-party SDK uses this type of tracking data.NSPrivacyCollectedDataTypePurposes
. An array of strings that lists the reasons why the application or third-party SDK collects data.
Values can be selected either from the list of data types found in the documentation or in the manifest file by clicking on the arrow:

After filling out the Nutrition Labels, our manifest looks like this:

It's worth noting that if your app's third-party SDK also collects data, it must also have its own manifest. In this case, there is no need to include information about the data the SDK collects in your manifest.
To understand whether you created and filled out the manifest file correctly, you can generate a report. To do this, select Product > Archive in Xcode.

In the archive, call up the options and select Generate Privacy Report.

The generated report for our application looks like this:

If third-party SDKs of our application collect data and include a manifest file, then the report should also display information about the data collected by the SDK.
APIs used
Privacy Accessed API Types – this is an array of vocabularies that describe the types of APIs accessed by an application or third-party SDK that are designated as APIs and require reasons to access.
Apple is concerned that our apps or third-party SDKs may use code to access device signals for the purpose of identifying the device or user, i.e. fingerprinting Regardless of whether the user allows the application to track, fingerprinting prohibited. Therefore, Apple has compiled a list of “potentially harmful” APIs for the user with specific approved reasons for use.
We are required to analyze our application code, and if it turns out that we are using these APIs, then we need to check the reason for use and correlate it with Apple's stated reasons. When comparing, there can be three outcomes of events:
If we are using an API for reasons other than those specified in Apple's documentation, and if using the API does not provide a specific benefit to the user, then we must not use that API.
If we are using the API for reasons other than those stated in Apple's documentation, but using the API provides a specific benefit to the user, then we can send request for approval of the reason for use.
If we use an API for reasons specified in Apple's documentation, we list the APIs used in the manifest file and indicate the reasons for use.
If a third-party SDK uses such APIs, then it must indicate the reasons for use in its manifest file.
APIs are divided into the following categories
File timestamp API
creationDate
modificationDate
fileModificationDate
contentModificationDateKey
creationDateKey
getattrlist(_:_:_:_:_:)
getattrlistbulk(_:_:_:_:_:)
fgetattrlist(_:_:_:_:_:)
stat
fstat(_:_:)
fstatat(_:_:_:_:)
lstat(_:_:)
getattrlistat(_:_:_:_:_:_:)
System boot time API
systemUptime
mach_absolute_time()
Disk space API
volumeAvailableCapacityKey
volumeAvailableCapacityForImportantUsageKey
volumeAvailableCapacityForOpportunisticUsageKey
volumeTotalCapacityKey
systemFreeSize
systemSize
statfs(_:_:)
statvfs(_:_:)
fstatfs(_:_:)
fstatvfs(_:_:)
getattrlist(_:_:_:_:_:)
fgetattrlist(_:_:_:_:_:)
getattrlistat(_:_:_:_:_:_:)
Active keyboard API
User defaults API
Most likely, you will need to automate detection of the use of the above APIs in your application code. The best way to do this is to write scripts, but we won't write scripts in this article.
Let's assume we use the following APIs and for the following reasons:
Attribute
creationDate
from File timestamp API due to the use of DDA9.1.Property
systemUptime
from System Boot Time due to the use of 35F9.1Class
UserDefaults
from User defaults API due to the use of CA92.1
After filling out this data in our manifest file we see:

Now we have a final completed version of the manifest file:

We've completed the first task and it's time to move on to the second: updating certain external dependencies (SDK) of our application. You need to find in it all the SDKs from the list published by Apple that require a manifest or manifest and signature. If SDKs are used as binary dependencies, then you must have a signed binary that also contains a Privacy Manifest file. Xcode 15 can show information about the SDK signature on the Inspector tab called Signature, where information about the signature method, its status, etc. is indicated.

This way we check our binary SDKs. If they are not signed, then you need to ask the SDK owners for signed binaries with the contents of the Privacy Manifest file. The owner of the SDK can sign them as a participant in the Apple Developer Program and as Self-Signed. The global difference is that to identify the Apple Developer Program, the company can check the validity of the certificate used, including if it has been revoked. This ensures that multiple developers cannot register under the same name. If the certificate used is Self-Signed, then application developers can confirm its validity and authenticity directly with the dependency author.
Some SDK authors on Apple's list have already started adding manifest files to their SDKs. You can go to their Github pages and try to find information about the manifesto or signature. If you haven’t found them, you can open a discussion or problem yourself with the topic of updating the SDK version with the contents of the manifest or manifest and signature.
There is a risk that there may be SDKs that do not want to be updated on time. Or some problems arise with the update, and you decide that it is better to look for alternative SDKs. Therefore, I recommend that you analyze your SDKs as early as possible and make a list for updating or replacing them with alternative ones.
Listings of SDKs, APIs, Nutrition Label data categories, etc. provided in this article are current at the time of writing. For up-to-date listings, you'll need to keep an eye on the following Apple documentation pages.
Sources:
WWDC 2023: Get started with privacy manifests, Privacy manifest files, Describing use of required reason API, App privacy details on the App Store, Describing data use in privacy manifests, WWDC 2022: Create your Privacy Nutrition Label, User Privacy and Data Use, WWDC 2022: Explore App Tracking Transparency, What's new in privacy on the App Store, Privacy updates for App Store submissions, Upcoming third-party SDK requirements, WWDC 2023: Verify app dependencies with digital signatures, Detecting when your app contacts domains that may be profiling users, Privacy updates for App Store submissions