PyTelegramBotAPI using the example of feedback collection project #1
So, we will begin our development with a small technical specification, in which I will list the main requirements for the bot.
The bot should greet the user and ask to indicate the product/service.
The product/service specified by the user is saved.
The bot asks you to write a review text.
After writing the text, the user is asked whether he wants to leave a review anonymously or not.
The written review is sent to the bot administrators via DM; if the review is not anonymous, then a link to the client’s telegram account will be provided.
This is the kind of bot we will develop.
Let’s start by getting a token. For those who don’t know, the token is needed to identify our telegram bot. Simply put, this is an analogue of authorization, only not for the user, but for the program.
To get a token, go to BotFather and click the Run or Start button.
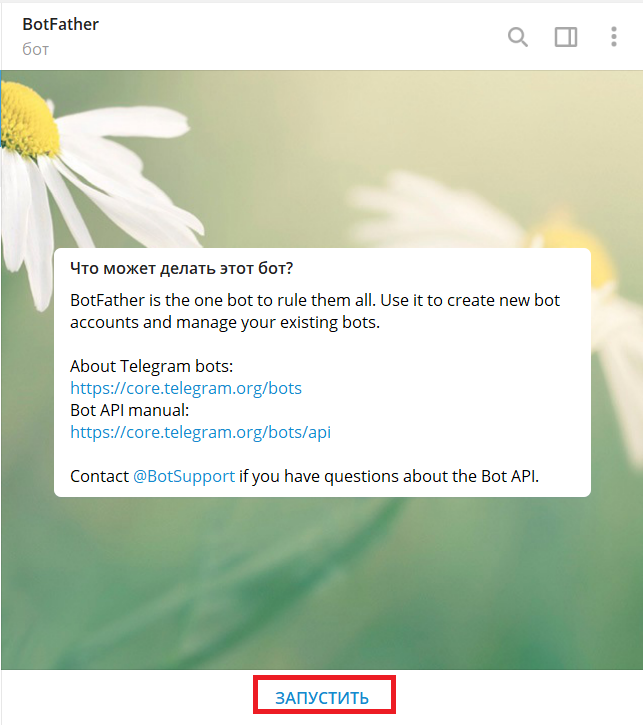
Next, click on /newbot
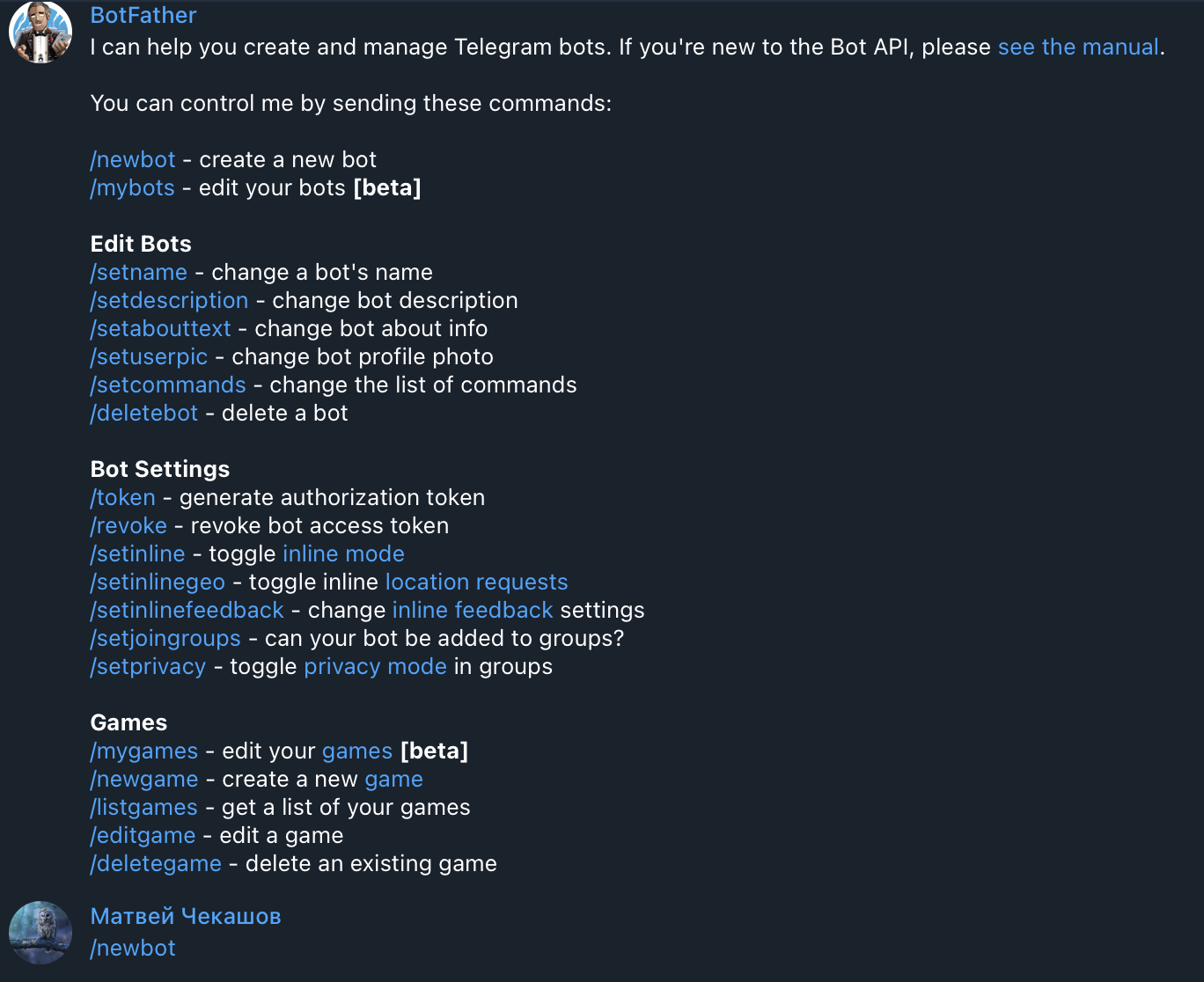
After this, we will indicate the name of the bot, what it will be called (it will be displayed during correspondence), it can be anything.
Next, we’ll indicate the username (analogous to a login on websites). Will be used to indicate links (and more). Must be unique and Necessarily end with the word Bot/bot/_bot

Great! We’ve sorted out the token. Now open our IDE, I will use PyCharm.
Create a project and install the PyTelegramBotAPI library.
pip3 install PyTelegramBotAPI
Next you need to save your token. There are several ways to do this, the best one is environment variables. But at the very beginning, I indicated that it was intended I will not use designs that are difficult for beginners. So let’s use another method, namely: create a new file, call it config.py and write the token there as a constant (A constant is an unchangeable variable. There are no full-fledged constants in Python, so we will use official replacementnamely, we will write the TOKEN variable in capital letters)
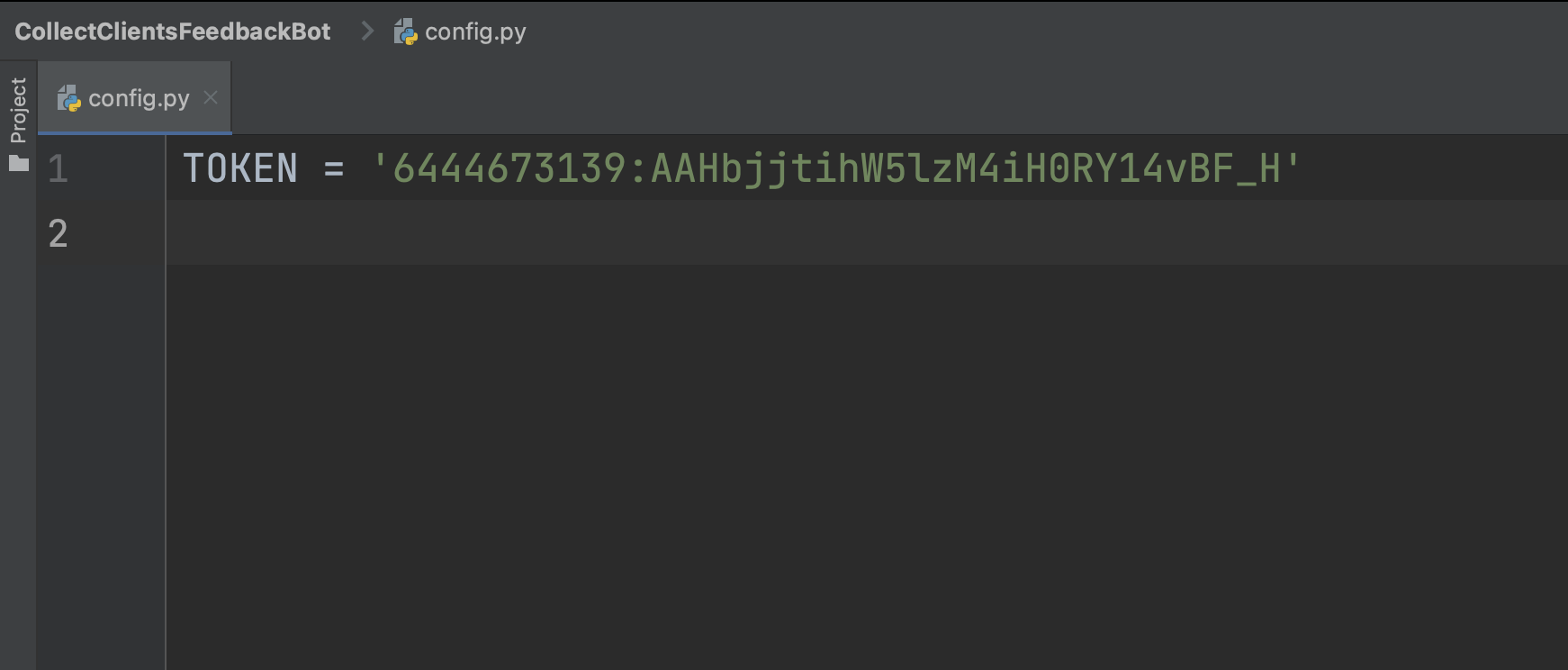
Our bot will be built on functions, many of which will have decorators. If you do not know these topics, I advise you to familiarize yourself, otherwise you will not understand much.
Let’s move on to writing the bot itself. Let’s open the main.py file and create a bot object, which we will work with in the future. We will also launch the bot immediately.
import telebot
from config import TOKEN
bot = telebot.TeleBot(TOKEN)
if __name__ == '__main__':
print('Бот запущен!')
bot.infinity_polling()
The construction __name__ == ‘__main__’ is used here, check it out if you don’t know what it’s for.
Let’s look at the code. In the first line we imported the telebot library, which we will work with. You may be surprised by the name of the library, because we downloaded PyTelegramBotAPI, and we are importing telebot. That’s ok, telebot is the name of the module we are importing from the PyTelegramBotAPI library. This module contains the main classes and functions for working with bots in Telegram.
In the second line we imported the token obtained earlier. In 4 we received an object of the TeleBot class; we will often work with the bot variable in the future (for example, it is with its help that we will send messages).
Next we launch our bot.
Now let’s add some functionality to our bot. Let our bot greet the user when sending the /start command.
To do this, let’s create a function welcome
and frame it with a decorator @bot.message_handler
import telebot
from config import TOKEN
bot = telebot.TeleBot(TOKEN)
@bot.message_handler(commands=['start'])
def welcome(message):
chat_id = message.chat.id
bot.send_message(chat_id,
'Привет! Добро пожаловать в бота сбора обратной связи!')
if __name__ == '__main__':
print('Бот запущен!')
bot.infinity_polling()
Let’s look at the code. We have already considered the first and last parts, we are only interested in the function.
Decorator is used message_handler
it allows you to tell the telegram what command we are monitoring (the command itself is transmitted in the list commands
). It is very convenient that the welcome function will be called only when the user writes /start; for other messages, the function will not be called.
Next, we get the id of the chat from which we received the message and save this id in the chat_id variable. Next, we send a message using the bot.send_message function, the function takes the chat id as the first parameter, and the message itself as the second.
Well, that’s all, the first article in the series “PyTelegramBotAPI using the example of a feedback collection project“ready. The next part will be in 3 days.
I hope everything was clear and interesting to you, if something is not clear to you, you want to complement or correct me – please leave a comment, I will try to read and take everyone into account.
The source code of the current bot is posted in the repository on GitHub: https://github.com/Ryize/CollectClientsFeedbackBot