Musical time and MIDI
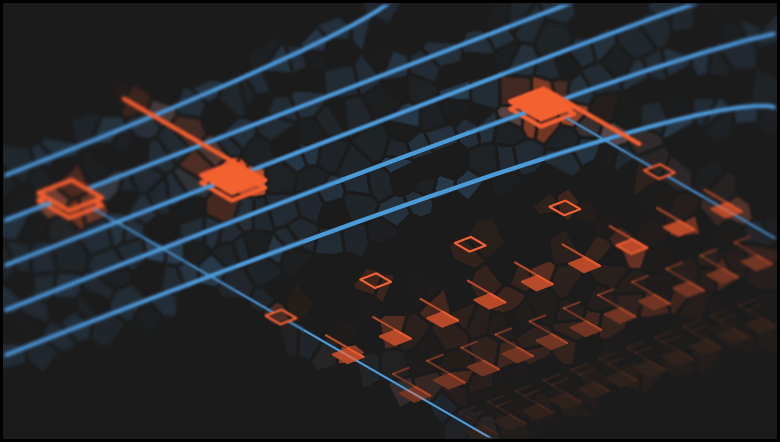
In this article we will complete the series of materials on converting MIDI time to other formats. Along the way, we will encounter an unexpected attack of overengineering, write a microscopic amount of code and discover the wrong music.
How does the process of creating music work in the modern world? Forgetting about fans of exclusively warm analogue methods, the algorithm looks like this in general terms: open on a computer DAWrecord instruments, make software bases for synthesizers, samplers, etc., add the necessary processing, mix, master.
Regarding tracks with virtually created notes, you cannot ignore the miracle of human thought – piano roll:
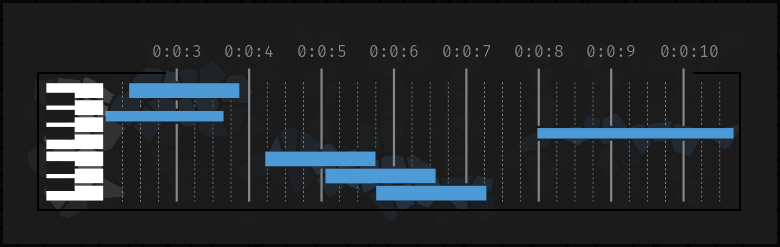
Yes, a link to a description of a tape for a mechanical piano, what does this have to do with computers? Just some interesting facts: used since the 1980s in software music processors, the piano roll has its roots in physical machines. By the way, tapes for them are still being produced. For example, QRS Music Technology offers some with a Christmas theme. Christmas, fireplace, mechanical piano…
If you sit down at an ordinary piano, with keys and strings, then the piece of music will appear before you on a staff, where various geometric figures will provide information on durations. Moreover, these durations are essentially mathematical fractions. This is a quarter note 1/4
half – 1/2
half with a dot – 3/4
and so on.
In MIDI like us we already know, time is represented by numbers that are not directly tied to formats understandable by homo sapiens. And although we have already learned to turn such numbers into measures and beatsas well as in seconds, minutes and others like them, we just have to look at fractions in order to close the topic of converting time from MIDI to various representations. That's what we'll do next.
We will call time in the form of a mathematical fraction musical, in order to somehow distinguish it from the formats we previously studied.
Table of contents
MIDI in musical time
Straight to the point. We have time in ticks T
. We want to understand what musical duration (fractions) x/y
) it matches. Knowing the duration of a quarter note in ticks (ticks per quarter note, TPQN
cm. Time in MIDI), we make the simplest proportion:
x/y = T
1/4 = TPQN
or
x/y = T/(4⋅TPQN)
When simple is not an option
In 2017, this is where the electrical circuitry in my brain malfunctioned. For some reason I thought: “Why not get rid of the fractions and see what happens?”. Multiplying both sides of the equality by 4⋅TPQN⋅y
we get
4⋅TPQN⋅x = T⋅y
or
4⋅TPQN⋅x - T⋅y = 0
“I don’t know how to solve this” — a thought flashed through my head. — “But it seems like we went through something like this at university”. After wandering around the Internet a little, I realized that we really studied such things, and they are called Diophantine equations. More precisely, we have before us a homogeneous Diophantine equation.
Better yet, we know what we're dealing with. But my studies at the university ended 3 years ago, mathematics was not my favorite subject, and therefore we are looking further.
Fortunately, there was a small manual Faculty of Mechanics and Mathematics at Moscow State University, where everything is explained simply and clearly. The homogeneous Diophantine equation in general form is expressed as follows:
a⋅x + b⋅y = 0
That is, we have
a = 4⋅TPQN
b = -T
Without stretching the length of the article, the solution will be like this:
x = T / GCD(a,b)
y = 4⋅TPQN / GCD(a,b)
Where GCD(a, b)
– greatest common divisor (GCD) a
And b
.
Nothing complicated
Let's see where I took a wrong turn. Let's go back to the equation
x/y = T/(4⋅TPQN)
What does a normal person see here? We are looking for x/y
and the answer was immediately written to us: T/(4⋅TPQN)
. In other words,
x = T
y = 4⋅TPQN
All that remains is to reduce the fraction until it stops. That is, divide x
And y
on GCD(T,4⋅TPQN)
. 100% the same answer, but obtained 1000% simpler. Well, we are all susceptible to needless complication sometimes.
The C# program that performs the manipulations above is extremely simple:
private static (long x, long y) MidiToMusical(long t, short tpqn)
{
var gcd = GreatestCommonDivisor(t, 4 * tpqn);
return (t / gcd, 4 * tpqn / gcd);
}
private static long GreatestCommonDivisor(long a, long b)
{
while (b != 0)
{
var remainder = a % b;
a = b;
b = remainder;
}
return a;
}
Two lines of basic code based on calculating the greatest common divisor Euclidean algorithm. All that remains is to make sure the calculations are correct:
const short tpqn = 100;
void TestMidiToMusical(long t)
{
var (x, y) = MidiToMusical(t, tpqn);
Console.WriteLine($"{t} ticks = {x}/{y}");
}
TestMidiToMusical(100);
TestMidiToMusical(200);
TestMidiToMusical(50);
TestMidiToMusical(400);
TestMidiToMusical(600);
TestMidiToMusical(20);
We will use TPQN = 100
i.e. 100
ticks corresponds to a quarter note (1/4
) duration. The program output is correct:
100 ticks = 1/4
200 ticks = 1/2
50 ticks = 1/8
400 ticks = 1/1
600 ticks = 3/2
20 ticks = 1/20
Well, for the sake of curiosity, let’s check some ridiculous number of ticks:
TestMidiToMusical(12345);
Conclusion:
12345 ticks = 2469/80
And it's true 1/80
this is the 20th part of a quarter duration, i.e. 5
ticks, and dividing 12345
on 5
we get the numerator of the shown fraction – 2469
.
Musical time in MIDI
Conversion from musical time x/y
back to MIDI ticks T
unacceptably simple. Returning to proportion
x/y = T/(4⋅TPQN)
we get
T = 4⋅TPQN⋅x / y
Expressing this in code:
private static long MusicalToMidi(long x, long y, short tpqn) =>
(long)Math.Round(4.0 * tpqn * x / y);
And check again:
const short tpqn = 100;
void TestMusicalToMidi(long x, long y)
{
var t = MusicalToMidi(x, y, tpqn);
Console.WriteLine($"{x}/{y} = {t} ticks");
}
TestMusicalToMidi(1, 4);
TestMusicalToMidi(1, 2);
TestMusicalToMidi(1, 8);
TestMusicalToMidi(1, 1);
TestMusicalToMidi(3, 2);
TestMusicalToMidi(1, 20);
TestMusicalToMidi(2469, 80);
Here we use the fractions obtained in previous tests, expecting to return to the numbers passed in TestMidiToMusical
higher:
1/4 = 100 ticks
1/2 = 200 ticks
1/8 = 50 ticks
1/1 = 400 ticks
3/2 = 600 ticks
1/20 = 20 ticks
2469/80 = 12345 ticks
Perfect. But there is a nuance. I already talked about it in the previous articlesearch by word “focus”. The trick is to use Math.Round
. As long as the musical times don't form endless decimals, you're fine. But let's look, for example, at 1/3
:
TestMusicalToMidi(1, 3);
In ticks, according to our algorithm, such a fraction is expressed as a number 133
. Now let's do the reverse transformation:
TestMidiToMusical(133);
The answer will be 133/400
almost 1/3
but still not the same.
Some might argue that such ridiculous durations would not be seen on actual sheet music. Probably yes. At the same time, there are completely legitimate music recordings special rhythmic divisions (triplets, quintuplets, how-to-play-ols, etc.), allowing you to express your creativity like this:

Notes highlighted in red have a duration of just 1/3
(three notes in the space of one whole). I think for different varieties avant-garde musicWhen performers are unable to play exactly what they intended, such things are completely commonplace.
By the way, while I was looking for what music with such strange durations could be called, I came across an article Wrong music. Suddenly, it’s not about music, but about the way guns were installed on German fighters of World War II. English versionas usual, much more detailed.
Conclusion
With this article we covered the topic of converting MIDI time into various human forms. To be fair, there are other ways to represent time, e.g. SMPTE timecode. But such formats are rare and highly specialized. Personally, I have never received any requests regarding other time representations from my library users DryWetMIDI.
Previous articles: