Mastering the AWS CDK: Setting Up a Custom Domain for Your HTTP Gateway
In the ever-evolving world of cloud services, the ability to customize and adapt configurations is not just a luxury, but a necessity. For developers and businesses looking to leverage the ecosystem AWS, Cloud Development Kit (CDK) has become an invaluable tool. Not only does it simplify infrastructure management, but it also provides a programmatic way to deploy resources on AWS. One such resource that often requires personalization is the API Gateway.
This article will provide step-by-step instructions for setting up a domain name for your HTTP Gateway using AWS CDK. Whether you’re an AWS user or a newbie, this guide promises to provide valuable information and practical steps to improve your cloud skills.
So grab yourself a hot cup of coffee and let’s get to work!
Typical AWS Infrastructure
Let’s look at the basic infrastructure that is widely used today. When working on one of the projects (TARGPatrol), we followed a similar approach, since it allows us to create flexible and powerful solutions.

What we have:
AWS VPC allows you to group the entire infrastructure into an isolated virtual network. This virtual network mirrors the regular network you manage in your own data center, but uses the scalable infrastructure of AWS.
AWS API Gateway is a fully managed service that makes it easy for developers to create, maintain, control, and secure APIs, no matter their size. Serving as an “entry point” for applications to retrieve data or consume business logic from backend services, this tool allows you to create both RESTful and WebSocket APIs. Additionally, API Gateway is compatible with containerized, serverless, and traditional web workloads. There are two types of gateways: REST and HTTP Gateway. REST is more expensive and provides additional functionality, for example, Client-Edge optimization, additional security settings. HTTP Gateway is simpler and resembles Nginx.
AWS Route 53 is a reliable and scalable Domain Name System (DNS) web service. It routes user requests to web applications hosted on AWS or on-premises installations. Additionally, AWS Route 53 allows you to register and associate custom domains with your AWS API Gateway.
AWS Lambda is a serverless computing service that makes it easy to run code for almost any application or backend process without the need to manage a server. Lambda can be enabled by over 200 AWS services and SaaS applications, allowing you to pay only for actual use.
AWS Lambda is optional. Alternatively, you can use services such as EC2, ECS or EKS.
In the vast world of cloud services, infrastructure as code (IAC) has carved out a significant niche. AWS Cloud Development Kit (CDK), Amazon’s own development in this area, represents a paradigm shift in thinking about cloud resources.
What is AWS CDK?
AWS CDK is an open source software development platform that allows you to define cloud infrastructure in code and expose it through AWS CloudFormation. Unlike traditional CloudFormation, where developers write cumbersome YAML or JSON templates, in CDK they can leverage the power of modern programming languages such as TypeScript, Python, Java or C#. This provides reusability and a more intuitive development experience.
Benefits of AWS CDK:
Intuitive infrastructure design: Developers can use their preferred programming languages, making the process smoother and reducing learning curve.
Reusable components: CDK introduces the concept of constructs – ready-made parts of cloud infrastructure. This allows you to reduce the amount of repetitive code and focus on the unique aspects of a particular project.
Strict Validation: Leveraging the power of major programming languages, CDK provides proactive feedback through the IDE, making it easy to identify problems before deployment.
Seamless integration with AWS services: Because of its native nature, the CDK integrates seamlessly with the broader AWS ecosystem, allowing you to seamlessly incorporate a variety of AWS services into your infrastructure.
Best practics: AWS CDK has built-in default settings that follow AWS best practices for optimal configurations and security.
Flexibility: While the CDK offers high-level components for quick customization, developers also have the ability to drill down and customize low-level resources as needed.
The entire TARGParol framework was built using AWS CDK/TypeScript and our team had no issues with it.
Preparation
First you need to select and register a custom domain. While this can be done through various providers such as GoDaddy, Cloudflare, etc. and subsequently transfer DNS control to AWS, I will demonstrate how to do this directly within AWS.
Go to Route 53 Service and select Domains -> Registered domains.
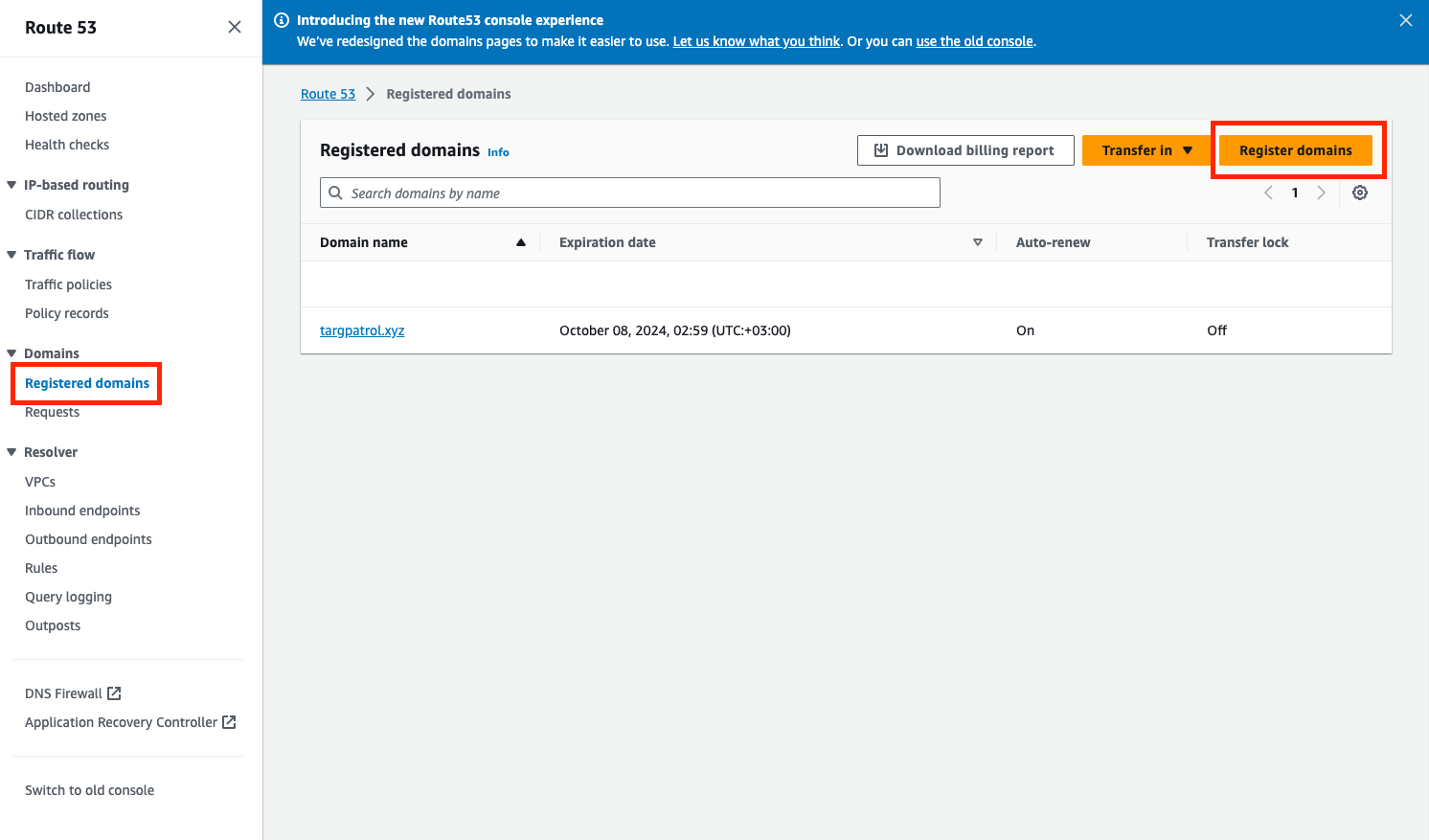
Next, you will need to select a suitable name for your custom domain and purchase it if available. The process will take a few minutes, after which you will be able to view your domain in the Hosted Zones section.

Ordering certificates
Once domain registration is completed, the next step is to obtain a certificate to secure HTTPS traffic.
Although the AWS CDK can make ordering a certificate easier, this process can be completed separately. Go to AWS Certificate Managementr and select “Request”. Select a public certificate. When prompted for a domain name, enter the name you previously purchased. If you want to protect a subdomain, you can specify an additional name, such as subdomain.example.com. You can also use wildcards like *.example.com to protect all subdomains of the main domain. Select function DNS Validation to confirm the connection between the certificate and the domain. You can rely on AWS for this process: when prompted to add DNS verification records, simply click OK.
Generating the certificate may take several minutes. The certificate can then be viewed in the AWS Certificate Manager dashboard.
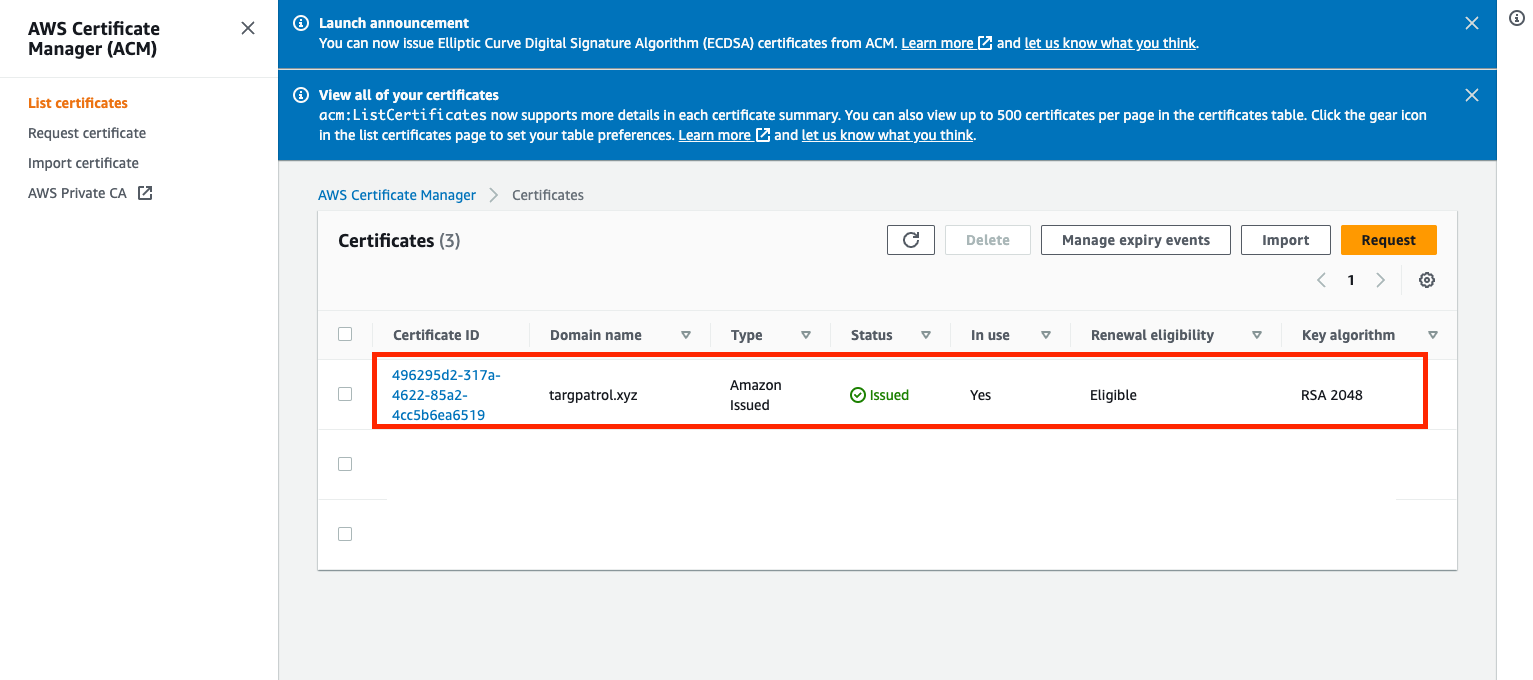
To integrate it into AWS CDK code you will need ARN. Simply click on the certificate and copy the ARN value.
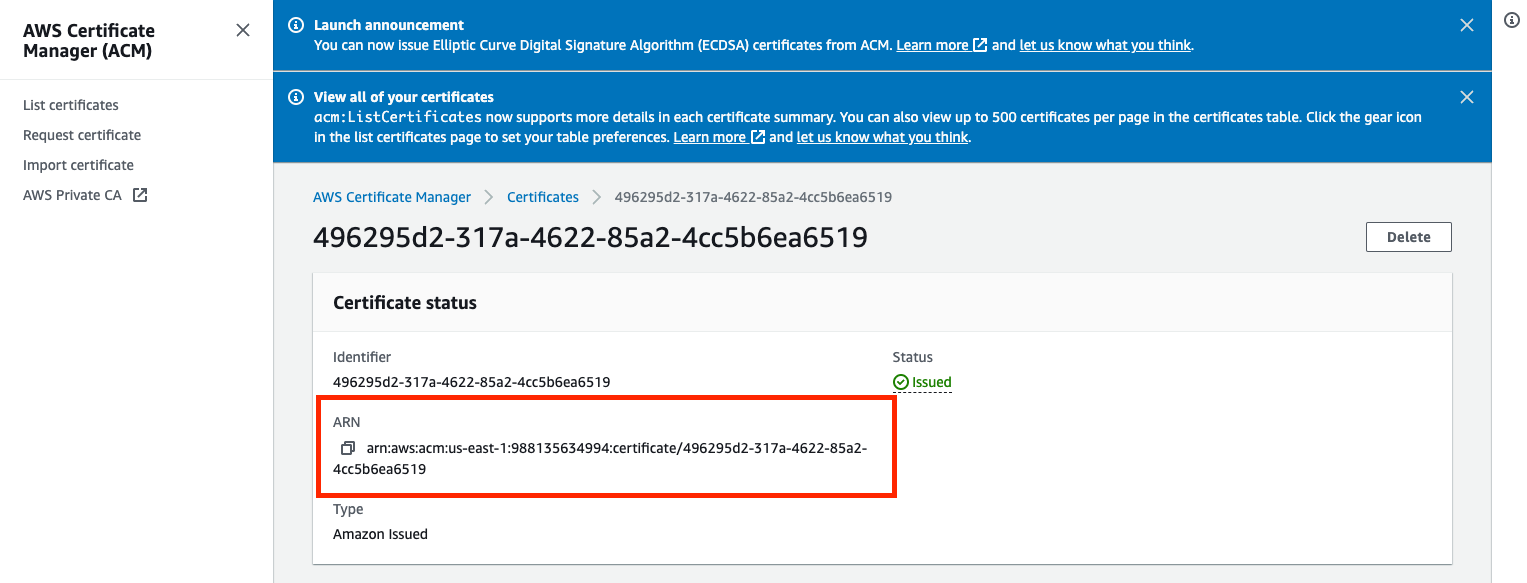
I hope you are familiar with AWS CDK. If not, then in order to take the first steps, you can use great article. In short, you just need to install AWS CDK CLI and create a new project.
The first thing you need to define in the AWS CDK stack is the VPC. It can be created with just a few lines of code:
const vpc = new Vpc(this, "VPC", {
vpcName: "VpcName",
ipAddresses: IpAddresses.cidr("10.0.0.0/16"),
subnetConfiguration: [
{ name: 'Public', cidrMask: 24, subnetType: SubnetType.PUBLIC },
{
name: 'Private',
cidrMask: 24,
subnetType: SubnetType.PRIVATE_WITH_EGRESS,
},
{
name: 'Isolated',
cidrMask: 24,
subnetType: SubnetType.PRIVATE_ISOLATED,
},
],
});
Here we are creating a new VPC with three subnets. Although this is not directly related to the topic of the article, it is wise to prepare yourself for any additional resources you may want to use in the future. Please note that natGateways does not matter if we are using Lambda as the backend. However, if you plan to deploy instances EC2 or ECS/EKS and give them access to the Internet, then at least one natGateway necessary.
We will need several constants from resources that were created manually:
const domainName = "targpatrol.xyz";
const hostedZoneId = "Z01395012LPLFQI32IEEA";
const certArn =
"arn:aws:acm:us-east-1:988135634994:certificate/496295d2-317a-4622-85a2-4cc5b6ea6519";
const lambdaRepository = "111122223333.dkr.ecr.us-east-1.amazonaws.com";
If you are not sure where to find these values, please refer to the screenshot provided earlier.
Now you can import ‘HostedZone’ and create a new domain. In addition, you need to configure a DNS record that will direct traffic to the API gateway, which we will do in the next step.
const hostedZoned = HostedZone.fromHostedZoneAttributes(
this,
"HostedZone",
{
zoneName: props.domainName,
hostedZoneId: props.hostedZoneId,
}
);
const domainName = new DomainName(this, "DomainName", {
domainName: props.domainName,
certificate: Certificate.fromCertificateArn(
this,
"Certificate",
props.certArn
),
securityPolicy: SecurityPolicy.TLS_1_2,
});
new ARecord(this, "DnsRecord", {
zone: hostedZoned,
recordName: props.domainName,
target: RecordTarget.fromAlias(
new ApiGatewayv2DomainProperties(
domainName.regionalDomainName,
domainName.regionalHostedZoneId
)
),
});
With the domain and DNS configured, we can begin working with the HTTP API Gateway. But first, let’s create a simple Lambda function as the backend.
const lambda = new DockerImageFunction(this, "LambdaFunction", {
functionName: "FunctionName",
code: DockerImageCode.fromEcr(
Repository.fromRepositoryName(
this,
"LambdaRepository",
props.lambdaRepository
),
{ tagOrDigest: "latest" }
),
});
Finally, you need to configure the HTTP API Gateway and connect the purchased domain to it. As mentioned earlier, AWS offers different types of API Gateways, including HTTP API and REST API. In short, the REST API provides more capabilities, but its cost is three times higher. The HTTP API can be compared to the basic nginx proxy service, which provides control over HTTP(S) traffic. In this tutorial I will be covering the HTTP API Gateway.
const gateway = new HttpApi(this, "HttpApiGateway", {
disableExecuteApiEndpoint: true,
defaultDomainMapping: {
domainName,
},
});
gateway.addRoutes({
path: "/api/v1/test",
methods: [HttpMethod.POST],
integration: new HttpLambdaIntegration("LambdaIntegration", lambda),
});
In Conclusion: Benefits of AWS CDK
Working in the AWS ecosystem may seem daunting at first, but tools like the AWS CDK are specifically designed to simplify and streamline the process. By allowing developers to use familiar programming languages and providing an intuitive framework for defining and deploying cloud infrastructure, AWS CDK truly revolutionizes the cloud development process.
The examples in this article only scratch the surface of the AWS CDK’s capabilities. As you delve deeper and experiment, you’ll discover a wealth of opportunities for optimization, automation, and innovation.
Using the AWS CDK is a step into the future of cloud deployment, where flexibility meets efficiency. As always, constant learning and adaptation are key to harnessing the full potential of any tool, and the AWS CDK is no exception.
Thank you for joining us on this exploration of the world of AWS CDK. We encourage you to dive in, experiment, and share your experiences with the wider developer community. Happy coding!
PS All code can be found in the repository here
PSS There is no point in discussing which is better Terraform CDK or AWS CDK, since AWS CDK is designed only for AWS. On the other hand, AWS’s documentation is the best I’ve ever seen.