Ktlint static analyzer for Kotlin in Android
Kotlin is a statically typed JVM-based programming language developed by JetBrains. It was introduced by Google primarily to create mobile applications on the Android platform. Using Kotlin, developers can write code faster and with better quality. However, as with any other programming language, it is important to follow a set of rules to ensure high performance and readable code.
Static code analysis
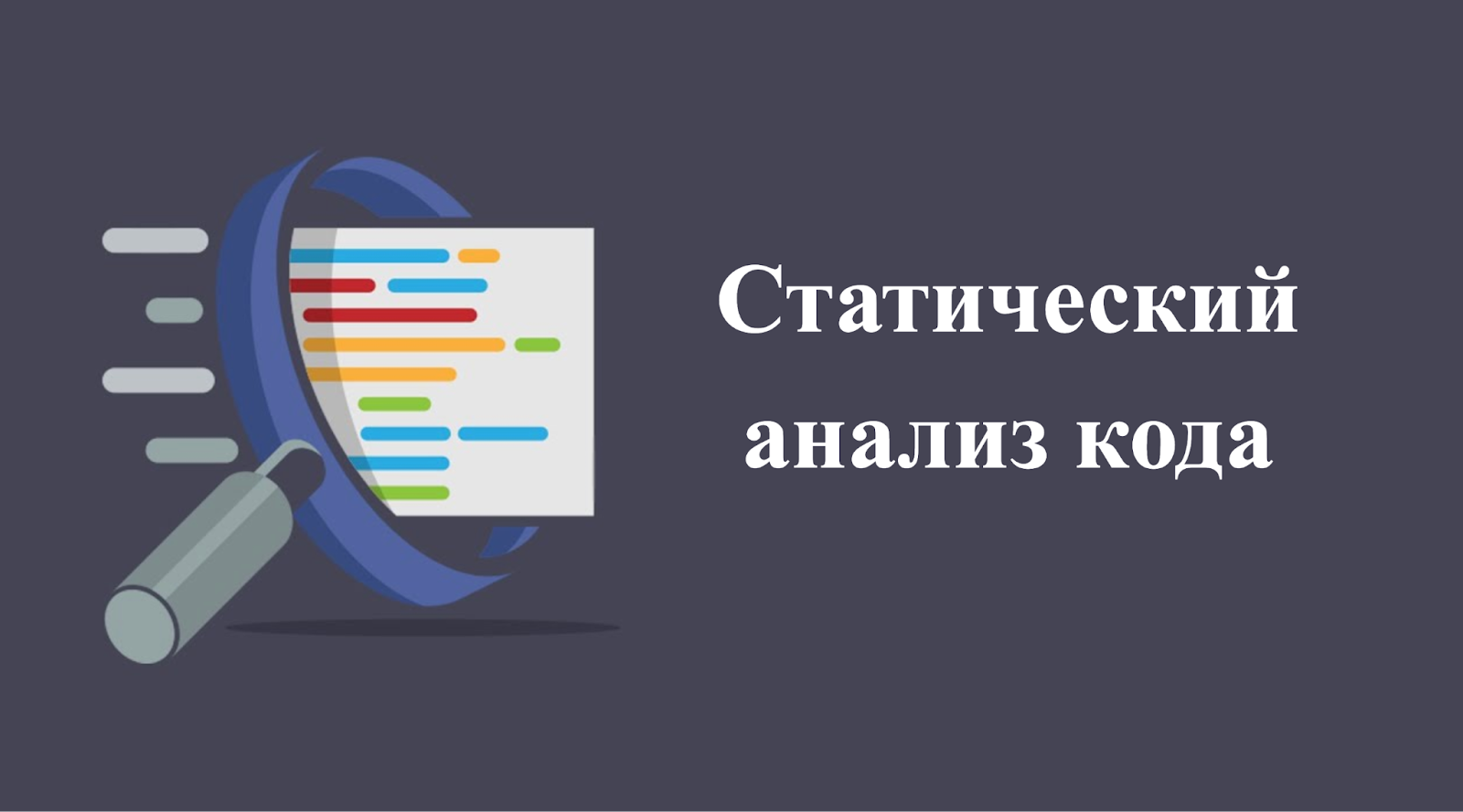
Static code analysis plays a fundamental role in the development process of software projects. It is not only a bug detection tool, but also a tool for improving the quality of your code. It helps you adhere to coding standards, improves development team productivity, and reduces time spent on debugging and refactoring. When creating large-scale applications involving multiple developers, static analysis becomes an integral part of development.
If your project is integrated with a continuous integration and continuous delivery (CI/CD) system, static code analysis is performed automatically during the testing phase. This allows you to identify and correct errors and vulnerabilities already at the stage of creating new assemblies, which increases the reliability and security of your application.
Code analysis tools for Kotlin

When creating applications based on Kotlin, developers may be faced with the need to use specific tools for static code analysis. In the Java world, there are well-known tools such as PMD, FindBugs and Checkstyle. However, with Kotlin, given its distinctive syntax, you need to look at other tools.
Among them are Ktlint, Detekt and the Jetbrains Inspection Plugin. In this article, we will look at how to easily and effectively implement the Ktlint tool in your Android project.
What is Ktlint?
Ktlint is a static code analysis tool written in the Kotlin programming language. It is part of the Kotlin development tools ecosystem and follows the Kotlin coding conventions and Kotlin Style Guide.
Key features of Ktlint
Code style check: Ktlint analyzes your code and checks it for compliance with coding standards, including indentation, use of spaces or tabs, line length, and more. This helps maintain code consistency across the project and makes it more readable.
Automatic formatting: When Ktlint detects violations of code style standards, it can automatically try to correct them. This saves developers time and simplifies the compliance process.
Integration with development environments: Ktlint integrates with popular development environments such as Android Studio and IntelliJ IDEA, allowing developers to see style hints and corrections directly in their IDE.
Customization of rules: You can customize Ktlint to suit your needs by setting your own code style rules or modifying existing ones.
The process of working with Ktlint can be divided into two main parts:
Code analysis(ktlintCheck) — This is the command that is used to run a static code analysis check using Ktlint. It scans and analyzes Kotlin source code according to the configuration rules defined for the project, and reports violations of coding standards if such violations are detected. This team helps developers identify and fix code problems, stylistic errors, or non-compliance with standards.
Code formatting (ktlintFormat) — This is a command that is also related to Ktlint, but instead of reporting errors and violations of standards, it attempts to automatically format the code according to Ktlint configuration rules. This is useful if you want Ktlint to automatically correct stylistic errors in your code.
So, by using Ktlint, you save your time and effort as you don’t have to check your code manually. Instead, you can start designing the logic for your application. Let’s look at how we can integrate Ktlint into a project.
Using Klint in your project
The first step is to create a Gradle file for Ktlint. Name it whatever you like, in our case, we’ll call it “ktlint.gradle”. Place this file at the root level where your “build.gradle” file is located.
// ktlint.gradle (project-level)
configurations {
ktlint
}
dependencies {
ktlint("com.pinterest.ktlint:ktlint-cli:1.0.0") {
attributes {
attribute(Bundling.BUNDLING_ATTRIBUTE, getObjects().named(Bundling, Bundling.EXTERNAL))
}
}
}
tasks.register("ktlintCheck", JavaExec) {
group = "verification"
description = "Check Kotlin code style."
classpath = configurations.ktlint
mainClass = "com.pinterest.ktlint.Main"
args "src/**/*.kt", "**.kts", "!**/build/**"
}
tasks.named("check") {
dependsOn tasks.named("ktlintCheck")
}
tasks.register("ktlintFormat", JavaExec) {
group = "formatting"
description = "Fix Kotlin code style deviations."
classpath = configurations.ktlint
mainClass = "com.pinterest.ktlint.Main"
jvmArgs "--add-opens=java.base/java.lang=ALL-UNNAMED"
args "-F", "src/**/*.kt", "**.kts", "!**/build/**"
}
We then move on to two important Gradle tasks that we detailed above: ktlintCheck and ktlintFormat.
We need to add our Gradle script file to the appropriate project modules. To do this, we go to the build.gradle(:app) file and add it as shown below:
// build.gradle (Module-level)
plugins {
id 'com.android.application'
/* ... */
}
apply from: "../ktlint.gradle"
android {
/* ... */
}
That’s all, really. Once the project is synced, you will be able to see the tasks in the Gradle menu.

Using Klint with a plugin in a project
To integrate the Ktlint plugin into the project, we will use the Ktlint plugin gradle. There are two ways to integrate the Ktlint plugin. If you are using some old project with old version of gradle, you can integrate Ktlint by applying the following code:
// build.gradle (project-level)
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath "org.jlleitschuh.gradle:ktlint-gradle:<current_version>"
}
}
apply plugin: "org.jlleitschuh.gradle.ktlint"
However, if you are using Ktlint in your project with any latest gradle version, you can apply the plugin in the file build.gradle root level:
// build.gradle (project-level)
plugins {
id "org.jlleitschuh.gradle.ktlint" version "<current_version>"
}
Also, if you need to add Ktlint for all modules of your project, write the following lines of code:
// build.gradle (project-level)
subprojects {
apply plugin: "org.jlleitschuh.gradle.ktlint"
ktlint {
debug = true
}
}
After adding these lines, you can sync your project and start using Ktlint.
You can now parse your ./gradlew ktlintCheck code and format it with ./gradlew ktlintFormat.
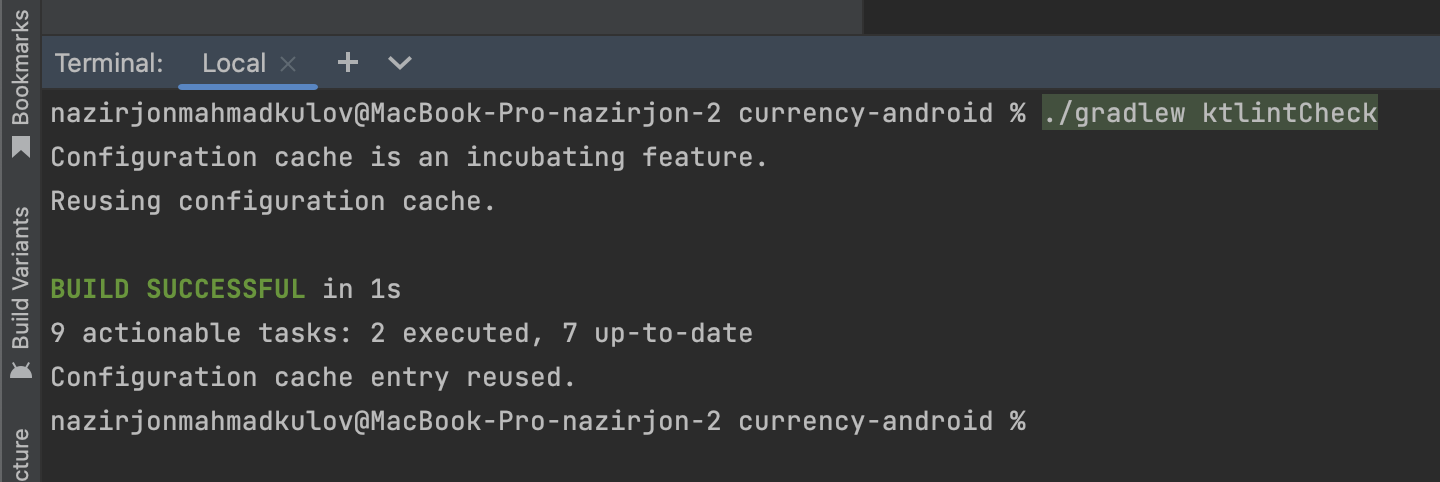
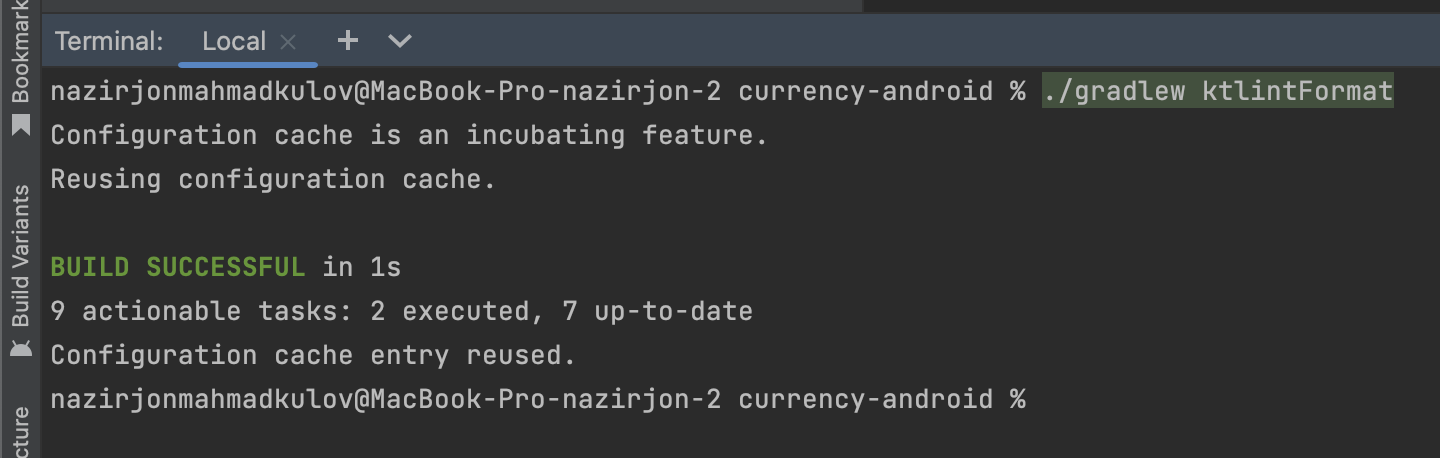
Setting style rules
To configure style rules using the Ktlint tool, you need to create a .editorconfig file in the root of your project.
.editorconfig is a configuration file that allows you to define formatting rules for various files in a project. Create this file in your project’s root directory if it doesn’t already exist.
Here is an example .editorconfig for Kotlin files:
# https://editorconfig.org
root = true
[*]
indent_style = space
indent_size = 4
max_line_length = 120
charset = utf-8
trim_trailing_whitespace = true
insert_final_newline = false
[*.{kt,kts}]
ktlint_standard = enabled
ktlint_disabled_rules=no-wildcard-imports
ktlint_code_style = ktlint_official
The rules in this example mean the following:
root = true: Indicates that this is the root configuration file. It applies to the entire project.
indent_style = space: Sets the indent style as spaces.
indent_size = 4: Sets the indent size to 4 spaces.
max_line_length = 120: Sets the maximum length of a line of code to 120 characters.
charset = utf-8: Specifies the UTF-8 encoding for files.
trim_trailing_whitespace = true: Specifies that whitespace should be removed at the end of lines.
insert_final_newline = false: Specifies that a blank line should not be inserted at the end of the file.
[*.{kt,kts}]: This section defines settings that apply only to files with the extension .kt and .kts (Kotlin files).
ktlint_standard = enabled: Enables code style checking using Ktlint standard rules.
ktlint_disabled_rules = no-wildcard-imports: Disables the no-wildcard-imports rule, allowing imports using an asterisk
.
ktlint_code_style = ktlint_official: Sets the official Ktlint code style.
This is just an example and you can customize .editorconfig according to your preferences. It’s important to note that Ktlint will use these settings to check your code style.
Integrating Ktlint review with Git CI
#ktlint check
stages:
- lint-check
lint-check:
stage: lint-check
before_script:
- chmod +x ./gradlew
- export GRADLE_USER_HOME=$(pwd)/.gradle
script:
- ./gradlew ktlintCheck
Integrating Ktlint review with Git’s continuous integration (CI) system (e.g. GitLab CI/CD, GitHub, etc.) allows you to automatically check Kotlin code style on every commit or pull request. This helps maintain stability and consistency of the code in the project. Here’s the general process for integrating Ktlint with Git CI:
This script performs a Ktlint check at the lint stage and runs the ./gradlew ktlintCheck command to check the code.
Thus, by integrating Ktlint with Git CI/CD, you automate the process of code style checking and ensure that the code entering your repository follows the Kotlin coding conventions and Kotlin Style Guide.
Conclusion
Ktlint is a powerful tool that helps simplify the process of maintaining clean and consistent code style in projects using Kotlin. By automating code review and formatting, it allows developers to focus on creating quality software without the tedious work of maintaining code style.
Ktlint plugin