How to make your telegram bot better? Of course, add analytics to it
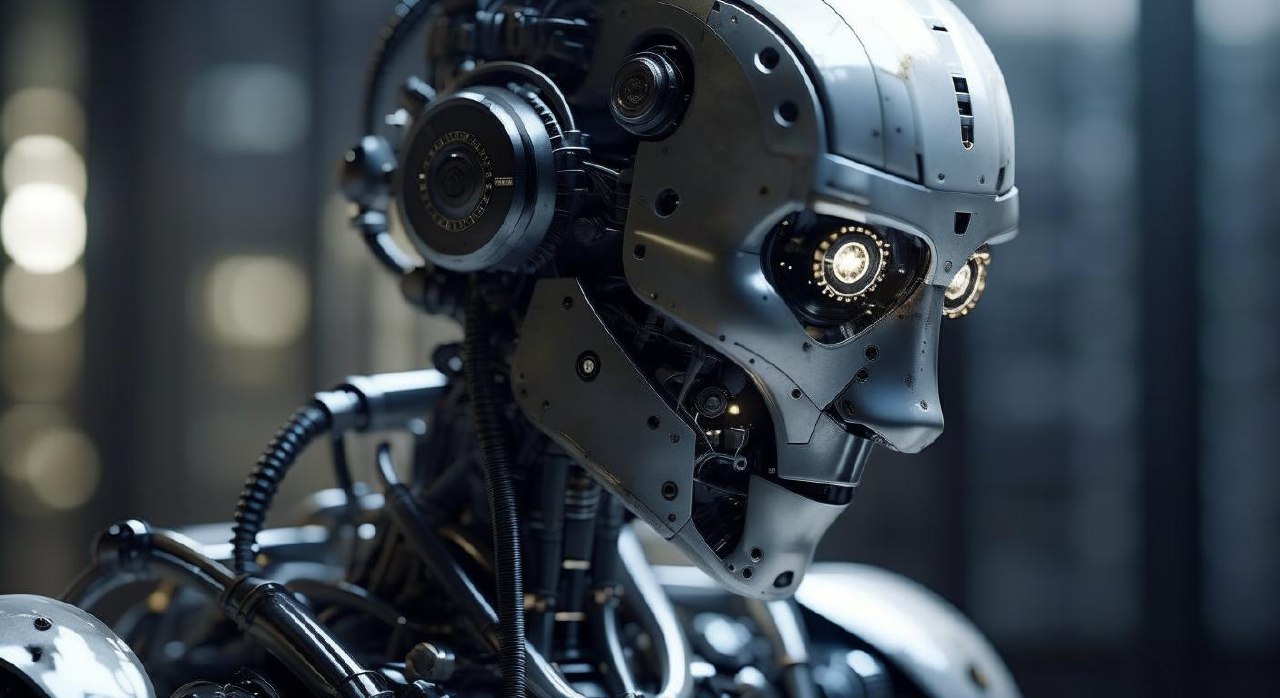
Nowadays, telegram bots have become indispensable tools for many tasks: from automating routine operations to providing high-quality customer service. They create new opportunities for businesses and public organizations by enabling real-time user interaction. However, successfully using telegram bots requires not only technical literacy, but also an understanding of how users interact with your bot and how this process can be improved.
Analytics for telegram bots is a key tool for understanding how users interact with your bot, what requests they make, what bot features are most popular, and what points require optimization. This data provides valuable information that allows you to make informed decisions and improve your bot over time.
Analytics for telegram bots not only helps improve the user experience, but also helps achieve your business goals. This could be an increase in the number of subscribers, an increase in conversion, a reduction in response time to requests, or any other metric that is important to your project.
Basics of creating telegram bots in Python
Let’s take a brief overview of the Telegram Bot API. It is a set of interfaces and methods provided by Telegram that allows developers to create and manage bots in the Telegram messenger.
Basic concepts that are important to understand:
Bot Token: Each bot in Telegram receives a unique token, which is necessary for authentication and interaction with the API. Getting a token is the first step when creating a bot.
Webhook: This is a callback method that allows Telegram to send your bot data about incoming messages and events in real time. Webhook is a more efficient way to get updates than polling the server.
Methods and Updates: The Telegram Bot API provides a variety of methods for sending messages, managing chats and users, and working with media content. All interaction with the API is based on sending HTTP requests.
Long Polling: Before the introduction of Webhook, a fairly common method for receiving updates was “long polling”. In this mode, the bot regularly checks for new updates, without waiting for them to arrive from Telegram.
Now that we understand the basic principles of the Telegram Bot API, let’s move on to creating our own telegram bot in Python. We will use the library telebot which provides convenient tools for interacting with the Telegram API.
Basic steps:
Installing the telebot library: Let’s start by installing the telebot library using pip:
pip install pyTelegramBotAPI
Creating a bot and receiving a token: To do this you need to contact BotFather in Telegram and follow the instructions to create a bot. The resulting token must be saved; it will be used for authentication.
Importing the library and setting up the bot:
import telebot # Замените 'YOUR_TOKEN' на фактический токен вашего бота bot = telebot.TeleBot('YOUR_TOKEN')
Writing commands and handlers: Create handlers for the commands and actions your bot will perform. For example:
@bot.message_handler(commands=['start', 'help']) def send_welcome(message): bot.reply_to(message, "Привет! Я ваш телеграм-бот. Для начала работы введите /start") @bot.message_handler(func=lambda message: True) def echo_all(message): bot.reply_to(message, message.text)
Launching a bot:
bot.polling()
Now your basic telegram bot is ready to work. He responds to commands /start
And /help
and repeats any incoming message.
We also looked at Telegram bots from the inside in more detail in this article.
Data collection for analytics
Understanding how to interact with the Telegram API to retrieve messages and user information is a key step in creating analytics for your telegram bot. Let’s look at several important aspects of this process.
Receiving messages from users: To collect message data from users, you can create a message handler that will be called every time a user sends a message to the bot. Example:
@bot.message_handler(func=lambda message: True) def process_message(message): # Здесь можно обрабатывать и анализировать сообщение от пользователя # Например, сохранить текст сообщения или данные о пользователе user_id = message.from_user.id user_message = message.text # Дополните этот код в соответствии с вашими потребностями
Getting information about users: For analytics, it is also important to get information about the users who interact with your bot. You can use Telegram API methods to get data such as user ID, first name, last name, and other parameters. Example:
@bot.message_handler(func=lambda message: True) def process_message(message): user_info = bot.get_user_info(message.from_user.id) user_id = user_info.id user_first_name = user_info.first_name user_last_name = user_info.last_name # Дополните этот код для сохранения информации о пользователе
Data storage and organization
Once you have received data from the Telegram API, it is important to store and organize it efficiently and securely. Here are some best practices:
Using the Database: To store large amounts of data and provide fast access, it is recommended to use a database such as SQLite, PostgreSQL or MongoDB. This will allow you to structure and store information about users, messages and analytics.
Anonymization of data: Be sure to consider issues of confidentiality and data anonymity. When analyzing user data, remove or anonymize personal information to comply with data protection regulations and respect user privacy.
Data backup: Back up your data regularly to prevent loss of information in the event of a failure or error.
Below is a Python code example that demonstrates how to use an SQLite database to store user and message information. In addition, the code will also show how to anonymize user data before saving:
import telebot
import sqlite3
# Подключение к Telegram API
bot = telebot.TeleBot('YOUR_TOKEN')
# Создание или подключение к базе данных SQLite
conn = sqlite3.connect('telegram_bot_data.db')
cursor = conn.cursor()
# Создание таблицы для хранения информации о пользователях
cursor.execute('''CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
username TEXT,
first_name TEXT,
last_name TEXT
)''')
# Обработчик сообщений для сбора информации о пользователях
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_info = message.from_user
user_id = user_info.id
username = user_info.username
first_name = user_info.first_name
last_name = user_info.last_name
# Анонимизация данных пользователя (для примера - просто удаляем фамилию)
last_name = None
# Сохранение информации о пользователе в базу данных
cursor.execute("INSERT INTO users (id, username, first_name, last_name) VALUES (?, ?, ?, ?)",
(user_id, username, first_name, last_name))
conn.commit()
# Запуск бота
bot.polling()
In this example, we are creating a SQLite database named telegram_bot_data.db and table users
to store information about users. The message handler writes the user’s data to this table and also anonymizes the user’s last name (for example).
Apart from SQLite, you can use other databases such as PostgreSQL or MongoDB depending on your needs and preferences.
Ethical and legal considerations for data analytics
When collecting and analyzing data from a telegram bot, you must adhere to high standards of ethics and legality. Below are some important aspects:
User consent: Make sure your bot users have provided consent for their data to be collected and analyzed. This is especially important if you are collecting personal information.
Data protection: Ensure strong data protection to prevent information leaks and unauthorized access.
Legal Compliance: Comply with local and global data protection and privacy laws. Work with legal experts if necessary.
Transparency: Inform users about what data you collect and how it will be used. Create a privacy policy for your bot and make it available to users.
Understanding the ethical, legal, and technical aspects of data collection is fundamental to successful bot analytics. In the following sections of the article, we’ll look at how to analyze this data and extract valuable insights to improve your bot and user experience.
Analytics functionality development
In this section, we will delve into the process of developing analytics functionality for your telegram bot. These steps will allow you to extract valuable data and insights from user interactions.
Defining key metrics and indicators
Before you start collecting data, you need to determine what metrics and metrics are important to your bot. This may include the following metrics:
Number of active users: Determine how many users actively interact with your bot on a daily, weekly, and monthly basis.
Frequency of use: Find out how often users access your bot and what commands they use most often.
Response time to requests: Measure how quickly your bot responds to user requests. Long delays can discourage users.
Conversion: If you have targeted actions (for example, signing up for a newsletter), determine user conversion.
Sample code to track the number of active users:
# Импортируем библиотеки для работы с базой данных (SQLite)
import sqlite3
from datetime import datetime
# Создаем или подключаемся к базе данных
conn = sqlite3.connect('telegram_bot_data.db')
cursor = conn.cursor()
# Функция для записи активности пользователя
def log_user_activity(user_id):
timestamp = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
cursor.execute("INSERT INTO user_activity (user_id, timestamp) VALUES (?, ?)", (user_id, timestamp))
conn.commit()
# Обработчик сообщений, вызывается при каждом сообщении от пользователя
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
log_user_activity(user_id)
# Остальной код обработки сообщений
# Запуск бота
bot.polling()
User activity analysis
User activity analysis allows you to understand how users interact with your bot and which features are most popular. You can analyze, for example, which commands users send most often or which keywords are most often mentioned in messages.
Sample code for analyzing user activity:
# Функция для анализа наиболее частых команд
def analyze_command_usage():
cursor.execute("SELECT text, COUNT(*) FROM messages WHERE text LIKE '/%' GROUP BY text")
command_usage = cursor.fetchall()
# Проанализировать результаты запроса и вывести статистику
# Обработчик сообщений
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
user_message = message.text
log_user_activity(user_id)
# Другой код обработки сообщений
# Запуск бота
bot.polling()
User segmentation and data grouping
User segmentation allows you to divide your audience into groups with common characteristics. For example, you can categorize users by location, language, or preferences. Grouping data allows you to analyze the activity and interests of each group separately.
Example code for user segmentation and data grouping:
# Функция для сегментации пользователей по языку
def segment_users_by_language():
cursor.execute("SELECT language, COUNT(*) FROM users GROUP BY language")
user_segments = cursor.fetchall()
# Проанализировать результаты запроса и вывести статистику
# Обработчик сообщений
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
user_language = message.from_user.language_code
log_user_activity(user_id)
# Другой код обработки сообщений
# Запуск бота
bot.polling()
Data visualization
Data visualization helps make analytics more visual and understandable. You can use data visualization libraries such as Matplotlib or Plotly to create graphs and charts based on your data.
Example code for creating a graph:
import matplotlib.pyplot as plt
# Функция для создания графика активности пользователей по дням
def plot_daily_activity():
cursor.execute("SELECT DATE(timestamp), COUNT(*) FROM user_activity GROUP BY DATE(timestamp)")
data = cursor.fetchall()
dates, counts = zip(*data)
plt.plot(dates, counts)
plt.xlabel('Дата')
plt.ylabel('Количество активных пользователей')
plt.title('График активности пользователей')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
# Запуск функции для создания графика
plot_daily_activity()
In this example, we are creating a graph that displays user activity by day.
Extracting Information from Text Messages
Extracting information from users’ text messages can be very useful for analytics. For example, you can analyze the text of messages to identify popular queries or topics that interest users. Example code for analyzing message text:
# Функция для анализа текста сообщений
def analyze_message_text():
cursor.execute("SELECT text FROM messages")
messages = cursor.fetchall()
# Проанализировать текст каждого сообщения и извлечь информацию
for message in messages:
text = message[0]
# Реализовать анализ текста и извлечение информации
# Обработчик сообщений
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
user_message = message.text
log_user_activity(user_id)
analyze_message_text(user_message)
# Другой код обработки сообщений
# Запуск бота
bot.polling()
Bot performance monitoring
For effective analytics, it is also important to track the performance of your bot. This includes monitoring request response times, error rates, resource usage, and other performance metrics. Example code for performance monitoring:
import time
# Функция для мониторинга времени ответа на запросы
def monitor_response_time():
start_time = time.time()
# Ваш код обработки запросов
end_time = time.time()
response_time = end_time - start_time
print(f"Время ответа на запрос: {response_time} секунд")
# Обработчик сообщений
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
user_message = message.text
log_user_activity(user_id)
monitor_response_time()
# Другой код обработки сообщений
# Запуск бота
bot.polling()
Integration with analytical tools
For more advanced analytics, you can integrate your bot with analytics tools such as Google Analytics to track user behavior on web pages associated with the bot. You can create goals and reports to measure the bot’s effectiveness in achieving specific goals.
Example of integration with Google Analytics:
from pygoogleanalytics import webapp
from pygoogleanalytics.exceptions import BadAuthentication
from pygoogleanalytics.exceptions import GoogleAnalyticsServerError
from pygoogleanalytics.exceptions import InvalidQueryParameters
from pygoogleanalytics.exceptions import QueryError
# Инициализация Google Analytics
ga = webapp('YOUR_USERNAME', 'YOUR_PASSWORD', 'YOUR_PROFILE_ID')
# Функция для отслеживания события
def track_event(category, action, label, value):
try:
ga.event(category, action, label, int(value))
except (BadAuthentication, GoogleAnalyticsServerError, InvalidQueryParameters, QueryError) as e:
print(f"Ошибка при отправке данных в Google Analytics: {str(e)}")
# Обработчик событий
@bot.message_handler(commands=['buy'])
def handle_purchase(message):
user_id = message.from_user.id
track_event('Purchase', 'Buy', 'Product', 1)
# Остальной код обработки покупки
# Запуск бота
bot.polling()
These additional steps will allow you to more deeply and fully analyze user interaction with your telegram bot and optimize its functionality and performance.
Integration of third party tools
Your telegram bot can become an even more powerful analytics tool if you integrate third-party tools and services. This will allow you to obtain additional data sources and analyze them using data analysis libraries. Let’s look at these aspects in more detail.
Using libraries for data analysis
The pandas library is a powerful tool for analyzing and processing data in Python. You can use it to aggregate, filter and visualize data received from your telegram bot:
import pandas as pd
import sqlite3
# Подключение к базе данных
conn = sqlite3.connect('telegram_bot_data.db')
# Загрузка данных о пользователях в DataFrame
users_df = pd.read_sql_query("SELECT * FROM users", conn)
# Вывод общей информации о данных
print("Общая информация о пользователях:")
print(users_df.info())
# Статистика по количеству пользователей по языкам
language_counts = users_df['language'].value_counts()
print("\nСтатистика по языкам пользователей:")
print(language_counts)
# Группировка данных по местоположению
location_grouped = users_df.groupby('location').size().reset_index(name="user_count")
print("\nГруппировка данных по местоположению:")
print(location_grouped)
This code demonstrates how you can use pandas to load data from a database and perform analysis, including displaying general information about the data, statistics by user language, and grouping data by location.
Integration with external services for additional data sources
Integration with external services allows you to receive additional data for analysis, for example, information about weather, financial quotes or news. You can use the APIs of these services to automatically retrieve data and integrate it into your bot’s analytics.
Example of integration with the OpenWeatherMap service to obtain weather data:
import requests
# Функция для получения данных о погоде
def get_weather(city):
api_key = 'YOUR_API_KEY'
base_url="https://api.openweathermap.org/data/2.5/weather"
params = {'q': city, 'appid': api_key, 'units': 'metric'}
response = requests.get(base_url, params=params)
weather_data = response.json()
return weather_data
# Обработчик команды для получения погоды
@bot.message_handler(commands=['weather'])
def get_weather_command(message):
user_id = message.from_user.id
user_city = 'Praha' # Замените на город, указанный пользователем
weather_data = get_weather(user_city)
# Обработка данных о погоде и отправка пользователю
temperature = weather_data['main']['temp']
description = weather_data['weather'][0]['description']
bot.send_message(user_id, f"Температура в {user_city}: {temperature}°C, {description}")
# Запуск бота
bot.polling()
This code demonstrates how the OpenWeatherMap API can be used to retrieve weather data from a user request and send the weather information back to the user.
Integration with notification and alert systems
Integrating your telegram bot with notification and alert systems allows you to instantly learn about important events and changes in your analytics. This may include sending notifications to your messenger when critical events occur or certain thresholds are reached.
Example of integration with the Pushbullet notification service:
import pushbullet
# Инициализация Pushbullet API
pb = pushbullet.Pushbullet('YOUR_API_KEY')
# Функция для отправки уведомления
def send_notification(title, body):
push = pb.push_note(title, body)
# Обработчик события, например, достижения определенного числа активных пользователей
if active_users >= 1000:
send_notification('Важное уведомление', 'Достигнуто 1000 активных пользователей!')
# Запуск бота
bot.polling()
In this example, when the bot reaches 1000 active users, it will send a notification using the Pushbullet service.
Using machine learning and text analytics
The integration of machine learning and text analytics allows you to automatically analyze and classify text messages from users, as well as predict their behavior. For example, you can use machine learning to determine the sentiment of user messages or classify queries.
An example of using the TextBlob library to analyze message sentiment:
from textblob import TextBlob
# Функция для анализа тональности текста
def analyze_sentiment(text):
blob = TextBlob(text)
sentiment_score = blob.sentiment.polarity
if sentiment_score > 0:
return "Позитивное сообщение"
elif sentiment_score < 0:
return "Негативное сообщение"
else:
return "Нейтральное сообщение"
# Обработчик сообщений
@bot.message_handler(func=lambda message: True)
def process_message(message):
user_id = message.from_user.id
user_message = message.text
log_user_activity(user_id)
sentiment = analyze_sentiment(user_message)
bot.send_message(user_id, f"Тональность вашего сообщения: {sentiment}")
# Запуск бота
bot.polling()
This code demonstrates how you can use the TextBlob library to analyze the sentiment of text messages and send a response to the user based on the analysis.
Integration with notification and alert systems, as well as the use of machine learning, allows you to more effectively monitor and analyze data from your telegram bot, as well as provide informative responses to users.
Automation of the analysis process
Automating data analysis in your telegram bot can greatly simplify the process of collecting and interpreting information. Developing scripts and creating schedules will allow you to conduct data analytics without constant intervention.
Development of scripts for automatic data processing
Let’s look at three code examples for automatic data processing in your telegram bot:
Example 1: Automatic aggregation of daily user activity data:
import sqlite3
from datetime import datetime, timedelta
# Подключение к базе данных
conn = sqlite3.connect('telegram_bot_data.db')
# Функция для агрегации данных о пользовательской активности за день
def aggregate_daily_activity():
today = datetime.now()
yesterday = today - timedelta(days=1)
cursor = conn.cursor()
cursor.execute("SELECT COUNT(*) FROM user_activity WHERE timestamp >= ? AND timestamp < ?", (yesterday, today))
daily_activity = cursor.fetchone()[0]
return daily_activity
# Расписание для выполнения скрипта раз в день
schedule.every(1).day.at("00:00").do(aggregate_daily_activity)
while True:
schedule.run_pending()
time.sleep(1)
This code uses the library schedule
to create a schedule that aggregates the previous day’s user activity data every day at midnight.
Example 2: Automatic analysis of the most popular user queries:
import sqlite3
from collections import Counter
# Подключение к базе данных
conn = sqlite3.connect('telegram_bot_data.db')
# Функция для анализа наиболее популярных запросов пользователей
def analyze_popular_queries():
cursor = conn.cursor()
cursor.execute("SELECT text FROM messages WHERE text NOT LIKE '/%'") # Исключаем команды
messages = cursor.fetchall()
message_texts = [message[0] for message in messages]
popular_queries = Counter(message_texts).most_common(10)
return popular_queries
# Расписание для выполнения скрипта раз в день
schedule.every(1).day.at("00:00").do(analyze_popular_queries)
while True:
schedule.run_pending()
time.sleep(1)
In this example, the code analyzes users’ text messages and determines the most popular queries.
Example 3: Automatically check bot performance and send reports:
import requests
# Функция для проверки производительности бота
def check_bot_performance():
# Оценка производительности бота, например, с использованием библиотеки locust
performance_result = run_locust_performance_test()
if performance_result['error_rate'] > 0.1:
send_performance_alert()
# Функция для отправки уведомления о проблемах с производительностью
def send_performance_alert():
chat_id = 'YOUR_CHAT_ID'
bot_token = 'YOUR_BOT_TOKEN'
message="Производительность бота снизилась. Проверьте работу."
url = f'https://api.telegram.org/bot{bot_token}/sendMessage'
data = {'chat_id': chat_id, 'text': message}
requests.post(url, data=data)
# Расписание для выполнения скрипта раз в час
schedule.every(1).hour.do(check_bot_performance)
while True:
schedule.run_pending()
time.sleep(1)
In this code, the script automatically checks the bot’s performance and sends a notification if performance is degraded.
Creating schedules for periodic analytics
For periodic data analytics in a telegram bot, you can create a schedule using the library schedule
, as shown in the examples above. When creating a schedule, consider the following points:
Determine the time intervals at which you want to run analytics (for example, once a day, once an hour).
Choose the right time to execute the script to minimize the impact on bot performance and user experience.
Consider sending notifications or reports to your messenger or email to receive information about the results of the analysis.
Regular automated analytics will help you quickly receive information about the dynamics of bot usage, user behavior and performance status. This will allow you to make more informed decisions and optimize the performance of your telegram bot.
Improving user experience
Understanding user behavior and preferences helps improve the user experience in your telegram bot. In this section, we’ll look at how to use the results of the analysis to personalize the user experience and optimize the bot’s performance.
Using analysis results to personalize the user experience
Personalization allows you to tailor each user’s experience based on their preferences and interaction history. Example code for personalizing messages:
# Функция для отправки персонализированных сообщений
def send_personalized_message(user_id, message):
user_info = get_user_info(user_id)
if user_info['preferred_language'] == 'ru':
message = translate_to_russian(message)
bot.send_message(user_id, message)
# Обработчик команды для запроса персонального приветствия
@bot.message_handler(commands=['hello'])
def send_personalized_hello(message):
user_id = message.from_user.id
hello_message="Hello, dear user!"
send_personalized_message(user_id, hello_message)
In this example, the bot sends a personalized greeting based on the user’s preferences.
Optimizing the bot’s performance based on analytics
Optimizing your bot’s performance is based on analyzing data about its usage and performance. Example code and optimization case:
Case: Improving the query response process using machine learning
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
# Функция для обучения модели машинного обучения на основе истории запросов пользователей
def train_bot_response_model():
messages = get_user_messages()
vectorizer = TfidfVectorizer()
message_vectors = vectorizer.fit_transform(messages)
similarity_matrix = cosine_similarity(message_vectors, message_vectors)
return similarity_matrix
# Функция для получения наилучшего ответа на запрос пользователя
def get_best_bot_response(user_message, similarity_matrix, messages):
vectorizer = TfidfVectorizer()
user_message_vector = vectorizer.transform([user_message])
similarity_scores = cosine_similarity(user_message_vector, messages)
best_match_index = similarity_scores.argmax()
return messages[best_match_index]
# Обработчик входящих сообщений
@bot.message_handler(func=lambda message: True)
def respond_to_user_message(message):
user_id = message.from_user.id
user_message = message.text
similarity_matrix = train_bot_response_model()
messages = get_user_messages()
best_response = get_best_bot_response(user_message, similarity_matrix, messages)
send_personalized_message(user_id, best_response)
In this example, the bot uses machine learning and text message analysis to determine the best response to a user’s query, improving the quality of responses and improving the user experience.
Data protection and privacy
Protecting user data and complying with Telegram API rules and data protection laws is extremely important to ensure user privacy and prevent leaks of confidential information. In this section, we’ll look at data security and compliance measures.
Measures to ensure the security of user data
Protecting user data should be the highest priority when developing and operating a telegram bot. Here are three code examples to ensure data security:
Example 1: Encrypting Data in a Database
import sqlite3
from cryptography.fernet import Fernet
# Генерация ключа шифрования
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Шифрование данных перед сохранением в базе данных
def encrypt_data(data):
encrypted_data = cipher_suite.encrypt(data.encode())
return encrypted_data
# Расшифровка данных при извлечении из базы данных
def decrypt_data(encrypted_data):
decrypted_data = cipher_suite.decrypt(encrypted_data).decode()
return decrypted_data
# Пример использования
conn = sqlite3.connect('secure_data.db')
cursor = conn.cursor()
sensitive_data="This is a secret message"
encrypted_data = encrypt_data(sensitive_data)
cursor.execute("INSERT INTO sensitive_info (data) VALUES (?)", (encrypted_data,))
conn.commit()
In this example, the data is encrypted before it is stored in the database and decrypted when it is retrieved.
Example 2: Restricting access to administrative functions
# Функция для проверки, является ли пользователь администратором
def is_admin(user_id):
admin_ids = [12345, 67890] # Список ID администраторов
return user_id in admin_ids
# Обработчик команды для администраторов
@bot.message_handler(commands=['admin_command'])
def admin_command(message):
user_id = message.from_user.id
if is_admin(user_id):
# Выполнение административной команды
else:
bot.send_message(user_id, "У вас нет доступа к этой команде.")
In this example, the commands intended for administrators
ators, are available only to certain users.
Example 3: Monitoring unauthorized access
import logging
# Настройка логирования для мониторинга активности бота
logging.basicConfig(filename="bot_activity.log", level=logging.INFO)
# Обработчик входящих сообщений
@bot.message_handler(func=lambda message: True)
def log_user_activity(message):
user_id = message.from_user.id
user_message = message.text
logging.info(f"User {user_id}: {user_message}")
In this example, user activity is logged to monitor and detect unauthorized access.
Compliance with Telegram API rules and data protection laws
When developing and using a Telegram bot, be sure to comply with the Telegram API rules, as well as data protection laws such as the General Data Protection Regulation (GDPR) in Europe, as well as the laws of your country. Keep libraries and dependencies up to date to ensure security and compliance with the latest standards.
Ensure that the bot does not collect or store sensitive user data without their consent, and always provide the user with information about the purposes and rules for processing their data.
Data protection and user privacy are key aspects of the development and operation of a telegram bot. Compliance with the highest security and legal standards will help build trust with users and prevent potential legal and reputational problems.
Implementations of data analytics on a bot
Let’s create a bot that collects and analyzes data about users, their activities and provides interesting reports based on this information. Let’s start by creating a bot and develop the analytics functionality step by step.
Step 1: Creating and setting up a telegram bot
The first step is to create a telegram bot and get its API key (token). To do this, you can contact the @BotFather bot on Telegram. Follow the instructions and get a token for your bot.
Step 2: Setting up the environment and installing libraries
Before you start, you need to install the necessary libraries such as telebot
, sqlite3
, pandas
, matplotlib
and others, depending on your needs.
pip install pyTelegramBotAPI
pip install pandas
pip install matplotlib
pip install sqlite3
Step 3: Initialize the bot and database
Create a file main.py
and add the following code to initialize the bot and database:
import telebot
import sqlite3
import pandas as pd
import matplotlib.pyplot as plt
# Инициализация бота
bot = telebot.TeleBot('YOUR_BOT_TOKEN')
# Инициализация базы данных
conn = sqlite3.connect('bot_data.db')
cursor = conn.cursor()
# Создание таблицы для хранения данных о пользователях
cursor.execute('''CREATE TABLE IF NOT EXISTS users (
user_id INTEGER PRIMARY KEY,
username TEXT,
first_name TEXT,
last_name TEXT,
join_date TEXT
)''')
# Создание таблицы для хранения данных об активности пользователей
cursor.execute('''CREATE TABLE IF NOT EXISTS user_activity (
user_id INTEGER,
activity_date TEXT,
activity_count INTEGER
)''')
conn.commit()
Step 4: Collect data about users and their activity
Now let’s add functionality to collect data about users and their activity. The bot will record information about users and also count their activity based on messages sent.
# Обработчик для записи информации о новых пользователях
@bot.message_handler(func=lambda message: message.new_chat_members is not None)
def record_new_users(message):
for user in message.new_chat_members:
user_id = user.id
username = user.username
first_name = user.first_name
last_name = user.last_name
join_date = message.date
cursor.execute('''INSERT INTO users
(user_id, username, first_name, last_name, join_date)
VALUES (?, ?, ?, ?, ?)''',
(user_id, username, first_name, last_name, join_date))
conn.commit()
# Обработчик для подсчета активности пользователей
@bot.message_handler(func=lambda message: True)
def count_user_activity(message):
user_id = message.from_user.id
activity_date = pd.to_datetime('today').date()
cursor.execute('''INSERT INTO user_activity
(user_id, activity_date, activity_count)
VALUES (?, ?, 1)
ON CONFLICT(user_id, activity_date)
DO UPDATE SET activity_count = activity_count + 1''',
(user_id, activity_date))
conn.commit()
Step 5: Report Generation and Data Visualization
Add functions for data analysis and reporting. In this example, we will create a report on daily user activity and create a graph for visualization.
# Функция для генерации отчета о ежедневной активности пользователей
def generate_daily_activity_report():
today = pd.to_datetime('today').date()
cursor.execute('''SELECT activity_date, SUM(activity_count)
FROM user_activity
WHERE activity_date >= ?
GROUP BY activity_date''',
(today - pd.DateOffset(days=7),))
data = cursor.fetchall()
df = pd.DataFrame(data, columns=['Date', 'Activity'])
return df
# Функция для построения графика активности пользователей
def plot_activity_report(df):
plt.figure(figsize=(10, 6))
plt.plot(df['Date'], df['Activity'], marker="o")
plt.xlabel('Date')
plt.ylabel('Activity Count')
plt.title('User Activity Over the Past 7 Days')
plt.grid(True)
plt.savefig('activity_report.png')
# Обработчик команды для запроса отчета
@bot.message_handler(commands=['activity_report'])
def send_activity_report(message):
df = generate_daily_activity_report()
plot_activity_report(df)
bot.send_message(message.chat.id, "Here is the user activity report for the past 7 days:")
with open('activity_report.png', 'rb') as photo:
bot.send_photo(message.chat.id, photo)
Of course, let’s complement the previous steps of this huge example of implementing data analytics on a telegram bot and add new steps:
Step 6: Generate and send reports in PDF format
Let’s add functionality to generate reports in PDF format and send them to users via a bot. We will use the library reportlab
to create PDF reports.
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
# Функция для создания PDF-отчета
def generate_pdf_report(df):
pdf_filename="activity_report.pdf"
c = canvas.Canvas(pdf_filename, pagesize=letter)
c.drawString(100, 750, 'User Activity Report')
c.drawString(100, 730, 'Date: {}'.format(pd.to_datetime('today').date()))
# Добавление графика в PDF-отчет
plt.savefig('activity_plot.png')
c.drawImage('activity_plot.png', 100, 500, width=400, height=300)
# Добавление таблицы с данными в PDF-отчет
data = [df.columns.values.tolist()] + df.values.tolist()
for i, row in enumerate(data):
for j, val in enumerate(row):
c.drawString(100 + j * 80, 500 - (i + 1) * 12, str(val))
c.showPage()
c.save()
return pdf_filename
# Обработчик команды для отправки PDF-отчета
@bot.message_handler(commands=['pdf_report'])
def send_pdf_report(message):
df = generate_daily_activity_report()
pdf_filename = generate_pdf_report(df)
bot.send_message(message.chat.id, "Here is the PDF user activity report for the past 7 days:")
with open(pdf_filename, 'rb') as pdf_file:
bot.send_document(message.chat.id, pdf_file)
The bot can now create PDF reports of user activity and send them to users when requested.
Step 7: Extending Data Analysis
Let’s add more functionality for data analysis, for example, analysis of popular words or keywords used by users.
from wordcloud import WordCloud
# Функция для анализа популярных слов в сообщениях пользователей
def generate_word_cloud():
cursor.execute('''SELECT username, message_text
FROM user_messages
JOIN users ON user_messages.user_id = users.user_id''')
data = cursor.fetchall()
text=" ".join([row[1] for row in data])
wordcloud = WordCloud(width=800, height=400).generate(text)
plt.figure(figsize=(10, 6))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.savefig('word_cloud.png')
# Обработчик команды для генерации облака слов
@bot.message_handler(commands=['word_cloud'])
def send_word_cloud(message):
generate_word_cloud()
bot.send_message(message.chat.id, "Here is the word cloud of popular words used by users:")
with open('word_cloud.png', 'rb') as photo:
bot.send_photo(message.chat.id, photo)
The bot can now create a word cloud to analyze popular words used by users in messages.
Step 8: Automate the process of analyzing and sending reports
Let’s add the ability to automatically generate reports and send them to the user on a schedule.
import schedule
import time
# Функция для отправки ежедневного отчета по расписанию
def send_daily_report():
df = generate_daily_activity_report()
pdf_filename = generate_pdf_report(df)
# Определите пользователя или чат, куда вы хотите отправить отчет
user_id_or_chat_id = 'YOUR_USER_OR_CHAT_ID'
bot.send_message(user_id_or_chat_id, "Here is the daily user activity report for the past 7 days:")
with open(pdf_filename, 'rb') as pdf_file:
bot.send_document(user_id_or_chat_id, pdf_file)
# Запуск задачи для отправки отчета ежедневно в определенное время (например, в 10:00)
schedule.every().day.at('10:00').do(send_daily_report)
# Функция для запуска планировщика
def run_scheduler():
while True:
schedule.run_pending()
time.sleep(1)
# Обработчик команды для запуска планировщика
@bot.message_handler(commands=['start_scheduler'])
def start_scheduler(message):
bot.send_message(message.chat.id, "Scheduler started. Daily reports will be sent at 10:00 AM.")
run_scheduler()
Now the bot can automatically generate and send scheduled reports.
This implementation of data analytics in a telegram bot becomes even more powerful with the addition of new features. You can customize it yourself, including analyzing other aspects of the data and creating different types of reports.
Conclusion
Researching and implementing data analytics in telegram bots provides many opportunities to gain a deeper understanding of user behavior and make more informed decisions.
Creating the analytical part of a telegram bot is an exciting process that can bring many benefits to both developers and users.
We also recommend you an article about the architecture of high-load telegram bots in Python.
Finally, I would like to recommend free webinar from colleagues at OTUS, where you’ll learn how to combine the power of SQL and the flexibility of Pandas for complex data analysis. You will also practice integrating SQL queries and Pandas, processing and storing analysis results in a database.