Flipper at minimum wages. How we make a device for reading and emulating intercom keys…
I liked Flipper Zero's idea of storing and emulating electronic keys. This is quite convenient, you can get rid of half of my ring with all the keys, plus those keys that I usually don’t even carry will be at hand, including universal ones. But I didn't like its dimensions. In addition, all the other functionality, although interesting to me, is unnecessary for this task, and you also have to pay for it. At that moment, an idea appeared and, most importantly, a desire to independently implement such a thing.
I work at a center for gifted students, so I first gave this topic to one of my most advanced students as a project. But then I plunged into this project myself 😀
The final idea was to emulate TouchMemory, Em-Marine and Mifare classic keys in one device. I would like this device to be as compact as possible, ideally to fit on a bunch of keys.
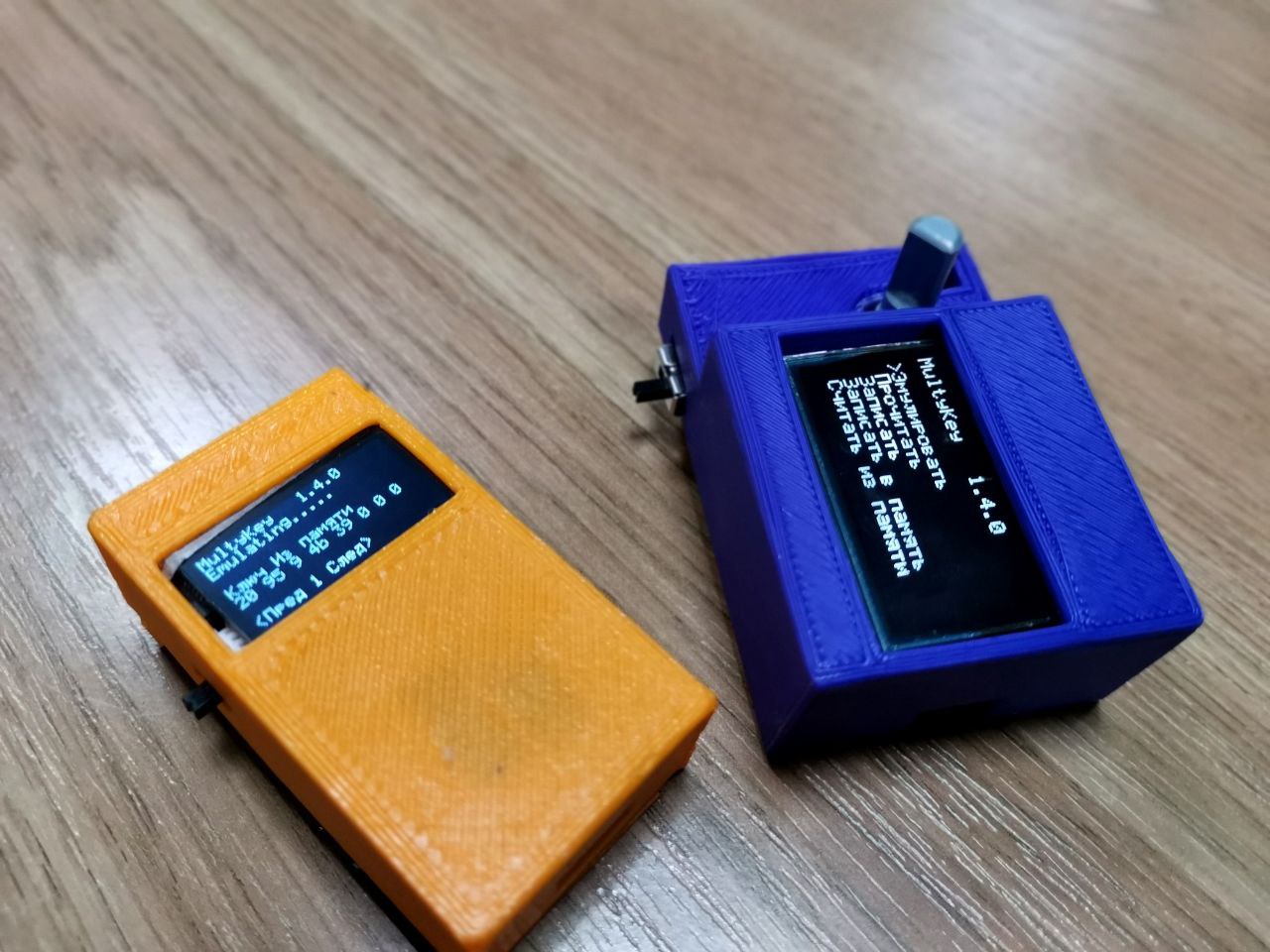
Let me first tell you what these standards are.
Common Key Standards
Em-Marine and Mifare classic are contactless keys that operate using wireless protocols at a frequency of 125 kHz for Em-Marine and 13.56 for mifare classic.
Em-Marine are old, rather stupid keys that store only their id, and are mainly used on turnstiles and intercoms (if they have not yet been replaced with Mifare classic).
Mifare classic operates at nfc frequencies, and, accordingly, it can be easily read by a modern phone, copied (unfortunately with limitations), and even sometimes emulated (not on all devices, often only with root rights, etc.).
I won’t go into too much detail about this standard; we haven’t gotten to the point of working with it yet.
TouchMemory is a class of electronic devices that have a single-wire communication protocol (1-Wire, but not always) and are housed in a standard metal case (usually tablet-shaped).
These are the same tablet keys that you lean against the intercom to enter.
About TouchMemory keys
Basically, the standard is presented in the form of Dallas keys and Russian folk Cyfral and Metacom.
Accordingly, we decided to start with these keys, because… they seemed to us the simplest. We decided to design this device on everyone’s favorite arduino, and code naturally in its environment.
To begin with, we decided to deal with dallas, after all, these are the most common keys. And they are usually supported by any intercom, even cyfral and metacom. These keys operate on the 1-wire protocol, the key itself is smart, can accept and process several standard commands, and stores only its unique ID of 8 bytes.
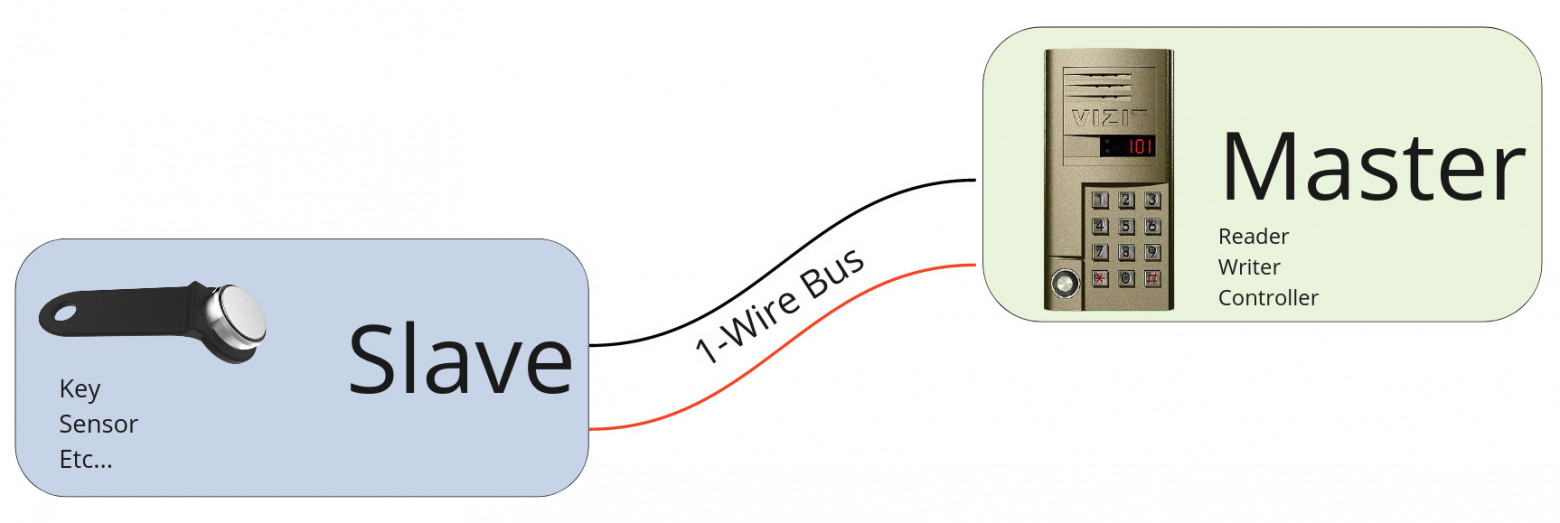
The 1-wire protocol works according to the Master-Slave model. In this topology, the Master device always initiates communication, and the Slave follows its instructions. When the key (Slave) contacts the intercom (Master), the key receives power, the chip inside the key turns on, the key is initialized, after which the intercom requests the key ID.
The intercom reads 8 bytes (64 bits) of information from the iButton to decide whether to open the door or not. The first byte is the family code. For ds1990 it is always 01, from it you can understand what kind of key it is (for example, for cyfral and metacom we will write their codes 10 and 20 here, in articles about them I will explain why this is so). Next are 6 bytes of the key itself and the last byte is the checksum. Sometimes the key code is written upside down, as in the diagram:

Connecting Arduino
To work with this protocol for Arduino, there is an excellent OneWire library, which allows Arduino to work as a Master device, and the firmware of almost all key duplicators on Arduino is written on it. The problem is that this library cannot work as a slave device, and, accordingly, it will not be possible to write emulation on it.
But first, it would be nice to at least read some key…
To provide the key with power, it is enough to pull the central contact to 5V through a resistor and the side contact to gnd. It is usually advised to use 2.2 kOhm, but, as practice has shown, you can use any from 500 Ohm to 5 kOhm, and even a voltage of 3.3 V is sufficient
To communicate with the dongle using this protocol, you also need to connect the central contact to some digital port. Next, open the example and download it.
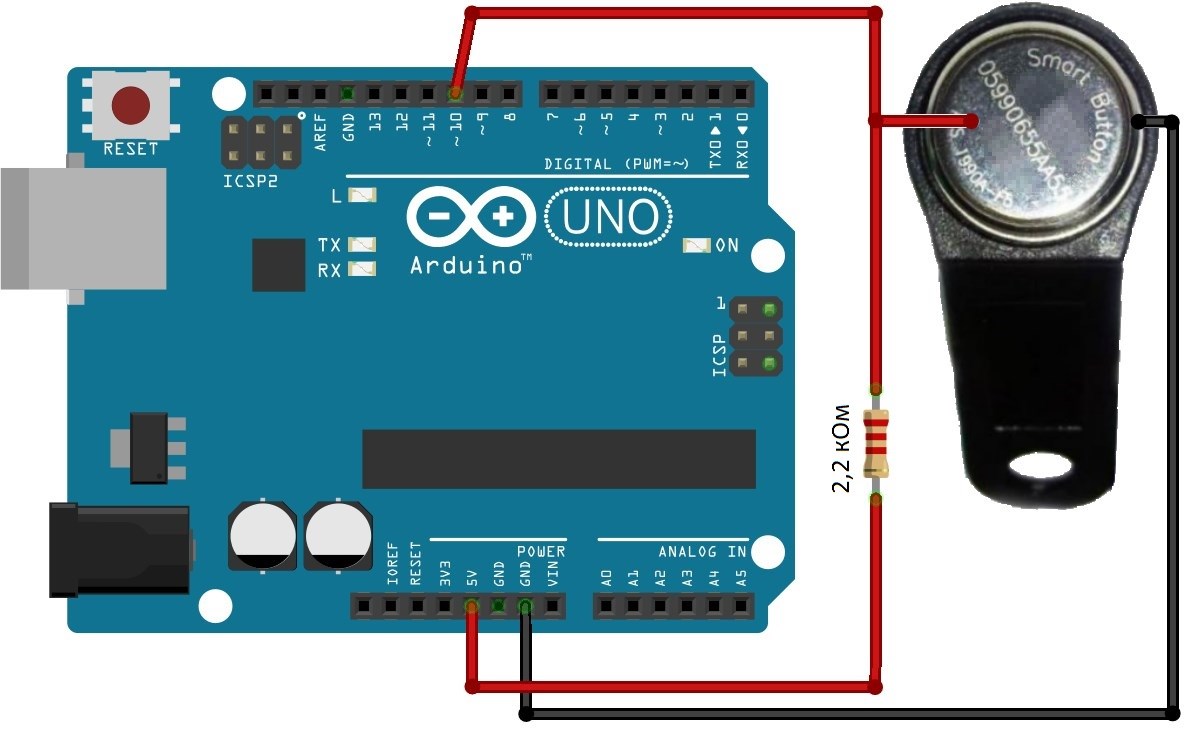
The reading itself takes place in just one line of code:
#include <OneWire.h>
#define iButtonPort 2
byte addr[8];
OneWire ibutton(iButtonPort);
void setup() {
Serial.begin(115200);
}
void loop() {
readKey();
}
void readKey() {
if (ibutton.search(addr)) { // Если устройство подключено - считываем
for (int i = 7; i > -1; i--) { // Запускаем цикл печати данных из массива
Serial.print(addr[i], HEX); // Печатаем нужный байт в шестнадцатиричном виде
Serial.print(" ");
}
Serial.println(); // В конце цикла переводим строку
ibutton.reset_search(); // Сбрасываем устройство
}
}
Let's get back to emulation
The search for a library for emulation was long, the first article I found was on RoboCraft: Arduino/CraftDuino and iButton emulator with unfinished code, and then the OneWireSlave library, which still cannot emulate ds1990 🙁
There was a desire to give up on everything and write my own library, but my laziness and perseverance led me to the OneWireHub library, which is based precisely on OneWireSlave :D. So she made great money.
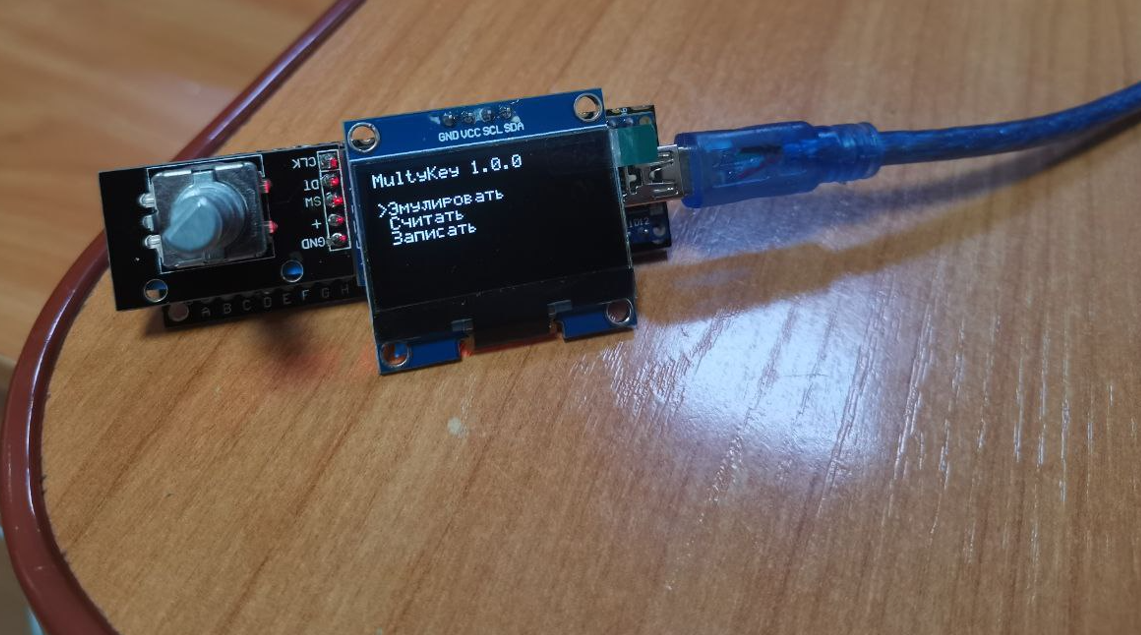

This library has an example of emulation, but for a bunch of devices in one sketch. I’ll probably give an example of a sketch with only key emulation.
#include "OneWireHub.h"
#include "DS2401.h" // Serial Number
#define iButtonPort 2
auto hub = OneWireHub(iButtonPort);
byte Key[8] = { 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x2F }; //универсальный ключ
auto ds1990A = DS2401(Key[0], Key[1], Key[2], Key[3], Key[4], Key[5], Key[6]); //crc считается автоматически
void setup() {
hub.attach(ds1990A); // всегда онлайн
}
void loop() {
hub.poll(); // необходимо периодически вызывать следующую функцию
}
As you can see, nothing complicated. But at this stage, problems already appeared with a lack of Arduino resources. It turns out that the atmega328p only has 2kb of RAM (more precisely SRAM) memory, and the buffer for working with OLED 128×64, which we decided to use, takes up exactly half.
In principle, it would be possible to optimize the firmware, tinker with the display, but there would definitely not be enough memory to work with Mifare Classic (although it is still far away), and I would like to write simpler code. Therefore, it was decided to switch to a more powerful controller.
Switching to ESP8266
And then the choice was between stm32f103 (or something similar) and esp8266. In fact, stm is more suitable for this project, it has more ports and useful hardware features, but stm32 is still poorly compatible with arduino code, so for now the choice has settled on esp8266.
The esp8266 already has 82 kB of SRAM memory and up to 160 MHz core frequency, which is even more than the stm32f103. Therefore, you don’t have to deny yourself anything, even paint pictures 😀
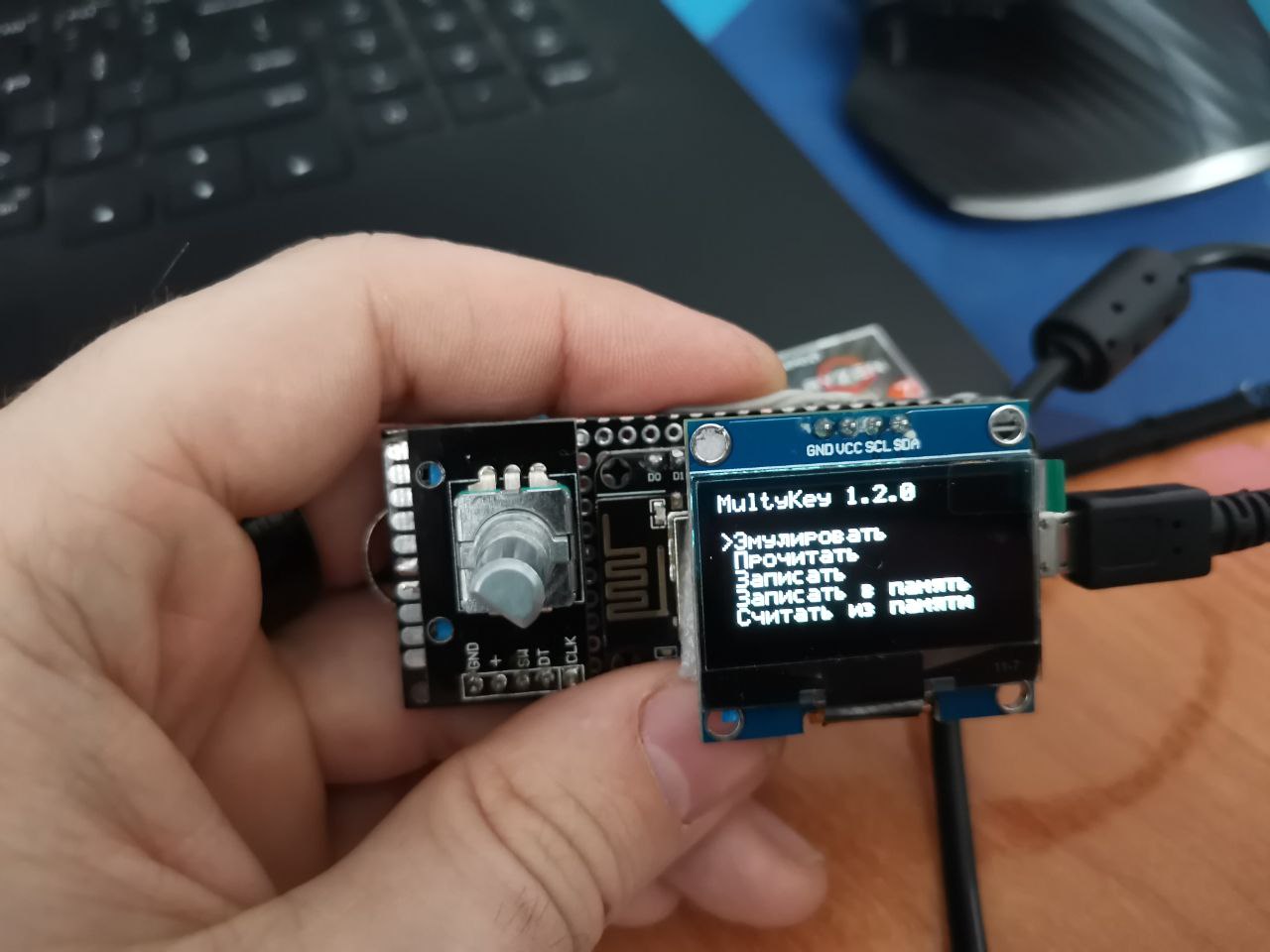
This piece of hardware was already able to read, write and emulate Dallas keys, as well as store them in memory. Also, of course, I added ten universal and frequently used keys into quick access 🙂
But it turned out not very compact 🙁
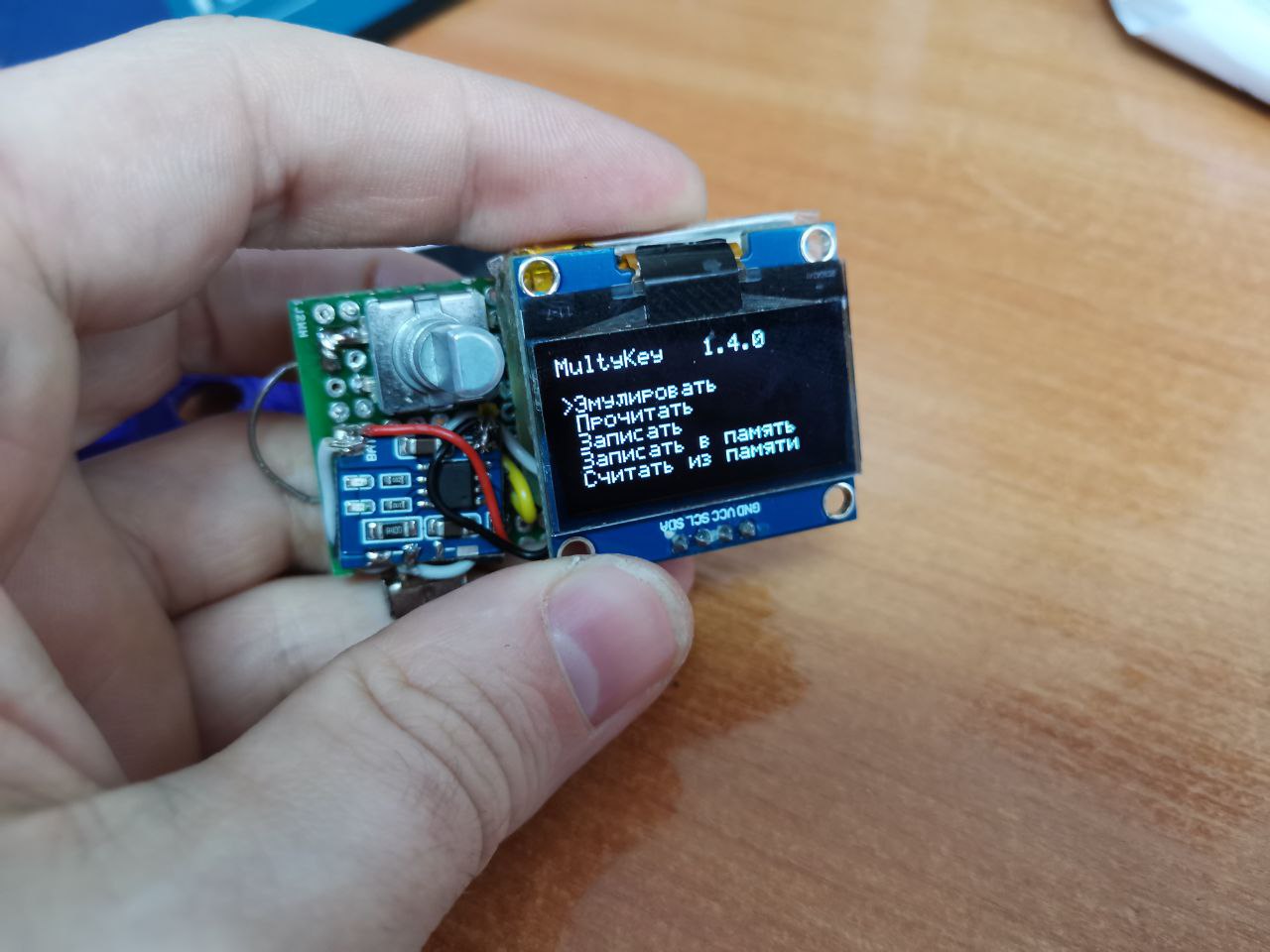
The next version was assembled on vemosMini v4, this is a more compact board based on esp8266. The device did not become significantly smaller, because… The screen was still quite large.
But now there is a battery with a charging board and a stylish purple case!
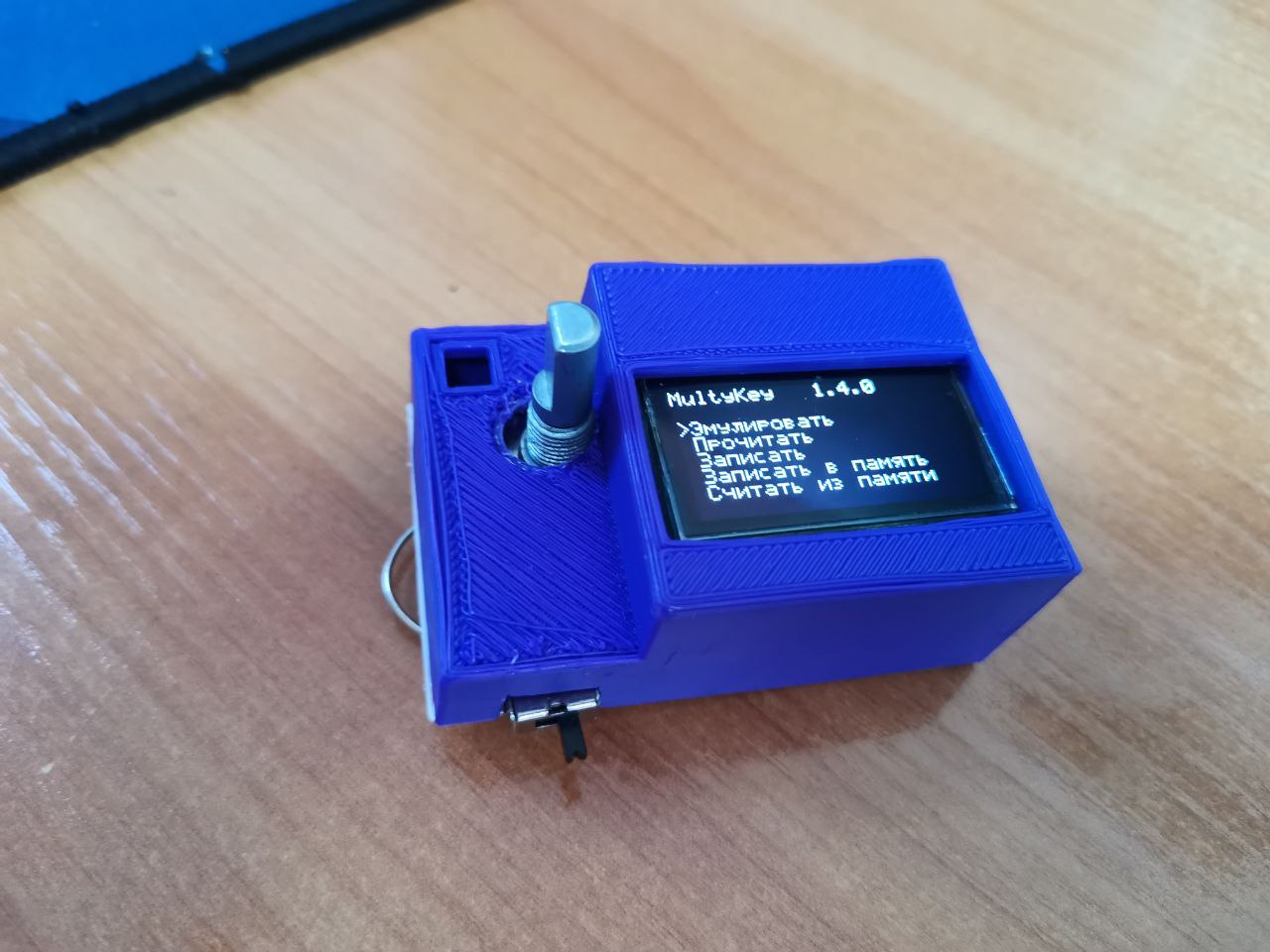
The next stage was the transition to a smaller OLED 1106_128x64. There is no need to display separate contacts for it, because it connects to a standard i2c connector on vemosMini v4 via wire. And with it we managed to achieve at least some compactness.
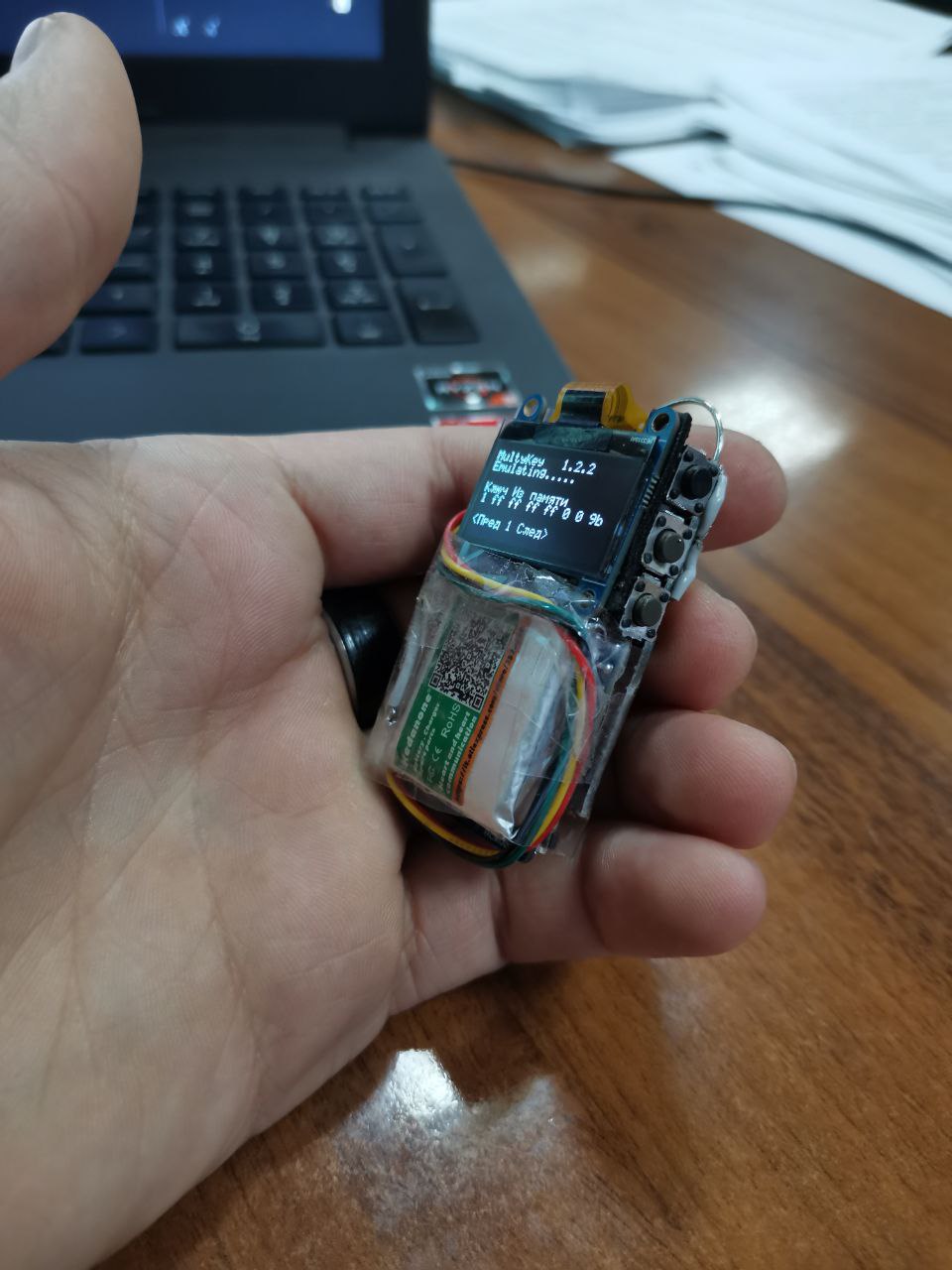
It turned out to be a bonus that GPIO2 on the vemosMini, as well as on the nodeMCU, is already pulled up to 3.3v through a resistor. Therefore, the device circuit has been simplified even more. True, not all boards have enough of this pull-up; sometimes you need to add a 1kOhm resistor at 3.3v.
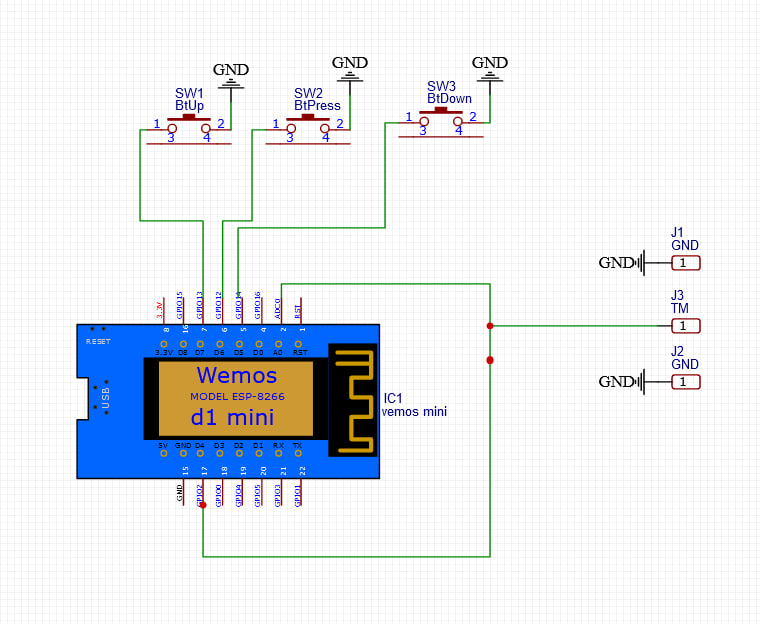
After some time, the building appeared. Crooked, of course, but for now this will do.


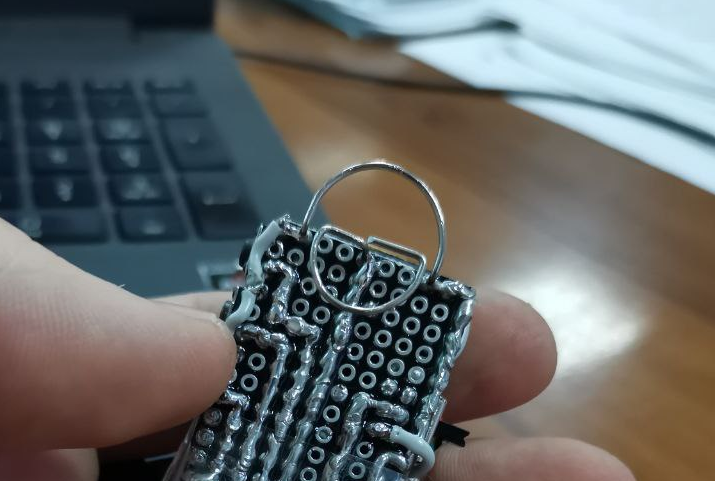
Yes, I forgot to mention the contact pad. We went through a bunch of options and settled on a form that allows you to conveniently read the key and has reliable and convenient contact with the intercom. This will most likely be changed in the future, but for now this is the most convenient option.
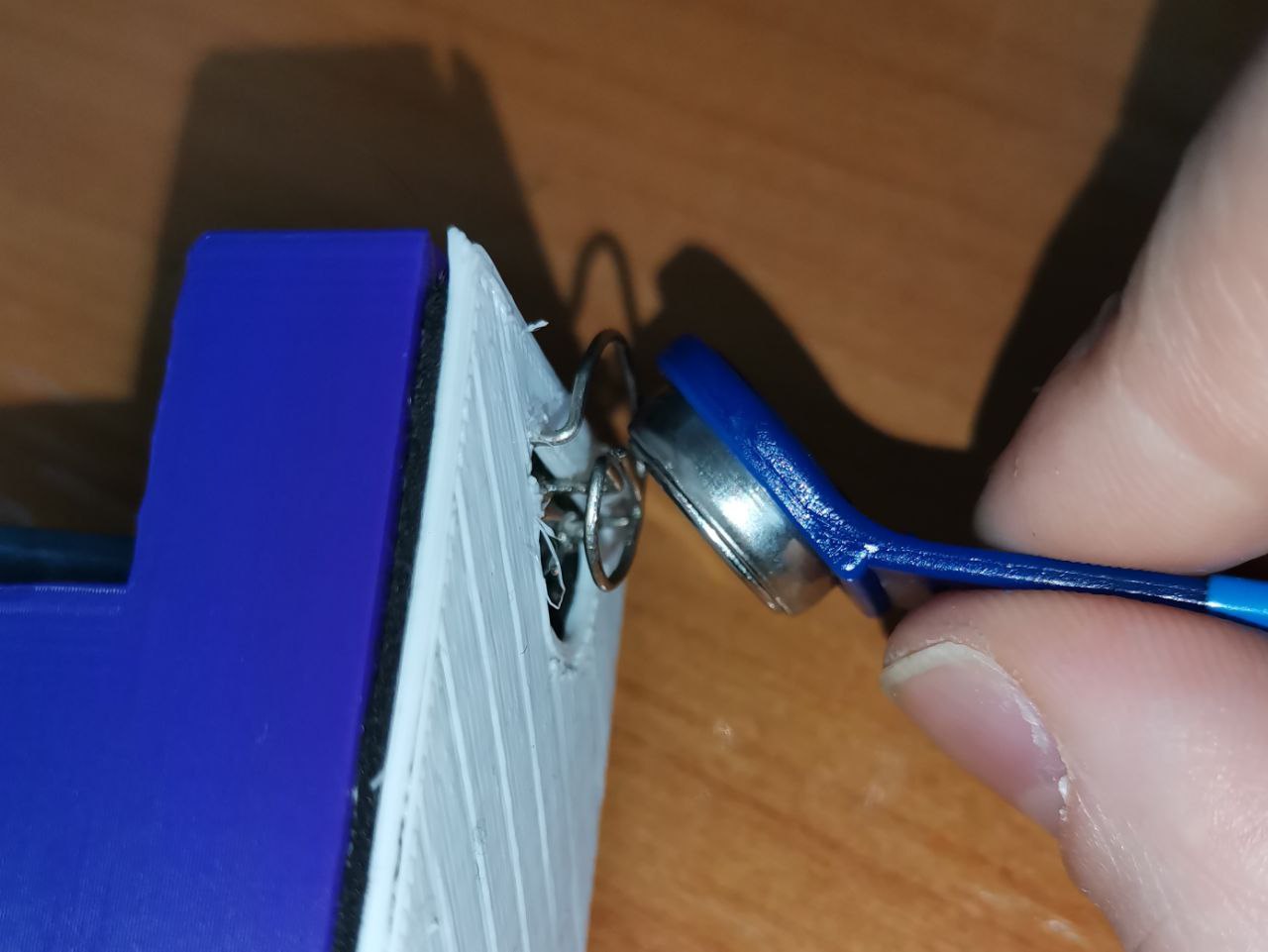
To achieve maximum compactness, of course, you need to assemble this thing on a printed circuit board, but the circuit diagram of the device is still changing all the time, and the signet is redrawn. I think that after defeating the contact keys, we will try to assemble a small batch of devices on printed circuit boards.

What has already happened
The next stage was the Cyfral and Metacom keys.
Spoiler! Everything worked out with Metacom, you can read, transcode and write to ds1990 blanks, write to specialized blanks and even emulate the original key! With Cyflal everything is worse, you can read and write in special. blanks, but the intercom does not accept the emulated key. It was also possible to read, write and emulate EM-Marine. But about all this in the following articles.