ESG (Evolution of Social Groups) algorithm. C#
Dear Community,
It is with great pleasure that I join your ranks to share my own developments in the field of optimization, and first, a few words about myself. My education is engineering in the field of industrial automation, and in parallel, for a long time I have been programming and developing trading strategies. My language of communication with financial markets is MQL5.
However, I have always felt drawn to optimization. And so, thanks to perseverance and perseverance, I was able to adapt one of these algorithms for trading many years ago 🙂 This was a real discovery for me, and I was fascinated by how algorithms can influence results in the financial sector.
My current research focuses on metaheuristic population optimization algorithms. I strive to develop my own unique methods and immerse myself in this creative process every day. Let's share our ideas together, discuss new trends and bring the most daring projects to life. After all, it is the unification of intellects and creative efforts that allows us to achieve great results. Go…
Multipopulation And multi-swarm Optimization algorithms are powerful tools that rely on the use of multiple independent populations to solve optimization problems. These algorithms work in parallel, exchanging information about optimal solutions and exploring different regions of the parameter space.
In this article, we will consider the multi-population algorithm for the evolution of social groups ESG (Evolution of Social Groups) of our own design, which I wrote literally in one breath in a couple of hours, and we will study the principles of its operation.
Multi-population optimization algorithms are methods in which multiple independent groups of agents work to solve the same optimization problem. Each group of agents operates independently of the other groups and conducts its search operations in the parameter space. This approach allows you to explore different areas of space simultaneously and find optimal solutions. Unlike “mono” – population algorithms, where all agents work in one population, multi-population algorithms provide greater flexibility.
Multi-population optimization algorithms share the following principles:
Populations (groups). Groups of agents collaborate and share experiences on the best solutions.
Collective movement. Particles within groups move together in parameter space.
Local and global experience. Groups save the best solutions (local within the group and global).
Evolution and exchange of experience. The algorithm goes through iterations, updating groups with improved solutions and sharing experiences.
Let's look at the features of the ESG search strategy. In a group of particles called a “social group”, there is a certain central pattern of behavior. Particles can deviate from the center according to the distribution law. The more adaptive behavior pattern becomes the new center of the group, so the group moves in search of a stable behavior pattern. This is a multi-population algorithm that models the behavior of group members at a low level and the global behavior of groups at a high level.
Groups can stop developing and get stuck at local extrema; most metaheuristic algorithms suffer from this problem. To avoid getting stuck, the tactic of “expanding the sphere of influence of a social group” is used. The group's boundaries are expanded to open up new areas of search and diversify the population of members in the group, which helps avoid getting stuck at local extremes. When a new solution is found, the boundaries are reduced, prompting the group to refine the solution.
However, simply expanding the zone of influence with a fixed current position of the group center may not be effective. In such cases, it becomes necessary to move the center of the group to a new position. To realize the incentive to move the center of groups, it is enough to exchange successful experience between groups, so particles are able to borrow ideas from the centers of other groups. The influence of new ideas can either lead a group to new discoveries and improve its position in the solution space, or worsen it. The behavior model of social groups is presented in the figure.
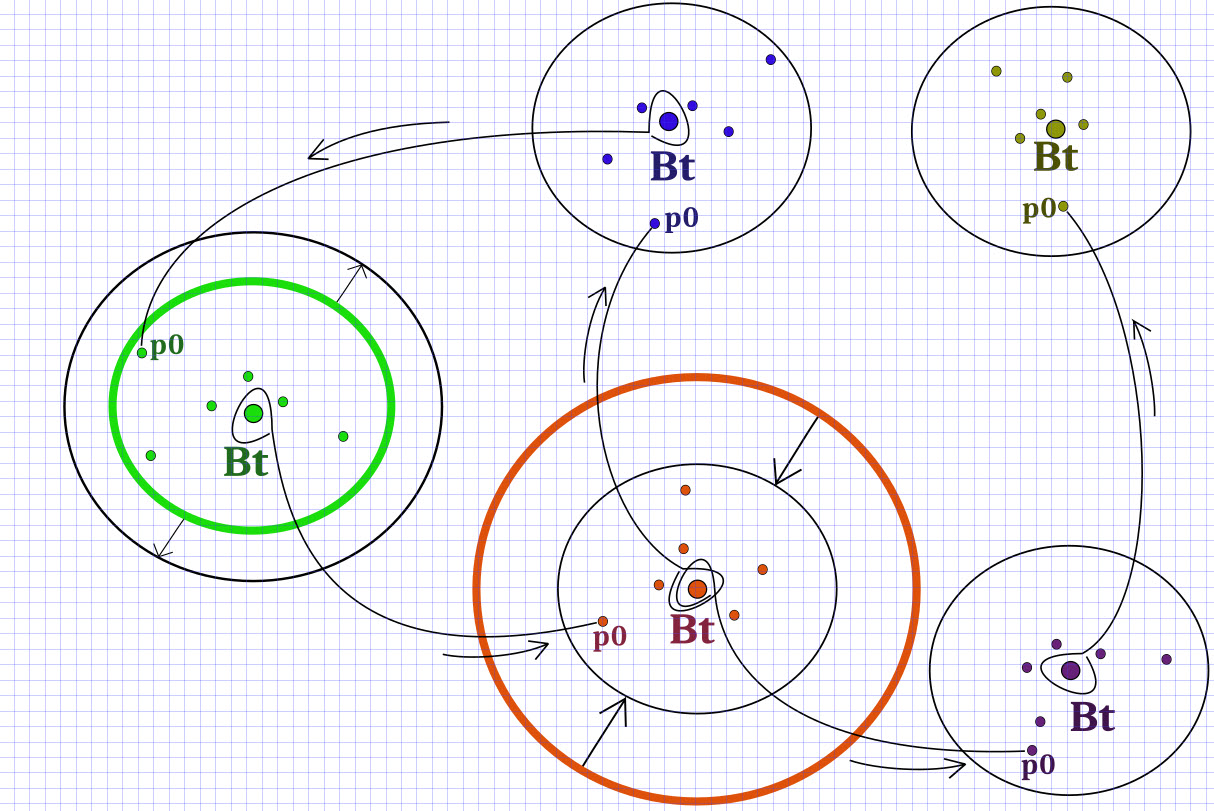
In the diagram above, the expansion of the group occurs in the absence of progress, narrowing – in the case of improvement of the solution, borrowing the “best ideas” (coordinates) from neighboring groups “Bt” (best of team) by particles “p0”.
The pseudocode of the ESG algorithm can be represented as follows:
Place group centers randomly in the search space.
Place group particles around the corresponding centers with a given distribution.
Calculate particle fitness values.
Update the global solution after step 3.
Update then the center of each group.
Expand the boundaries of groups if there is no improvement in the position of the center and reduce if the situation has been improved.
Place group particles around the corresponding centers with a given distribution.
Add information from the centers of “outgroups” to one particle of each group (the particle receives a set of coordinates from outgroups chosen at random).
Calculate the values of the particle fitness function.
Repeat from step 4 until the stopping criterion is met.
Implementation of the ESG algorithm in C#
The “Evolution of Social Groups” (ESG) algorithm in C# includes the following basic steps:
1. “Initialization”: The structures “S_Group” and “S_Agent” are created to represent groups and agents, respectively. The “C_AO_ESG” class initializes these structures and algorithm parameters.
2. “Optimization loop”: In a loop that continues until the stopping criterion is met, the following steps are performed:
“Fitness function calculation”: The fitness function value is calculated for each agent.
“Update Groups”: Groups are updated based on the current positions of the agents.
“Update positions”: Agent positions are updated depending on their group.
3. “Return the best solution”: After the optimization cycle is completed, the best solution found is returned.
Description of individual methods in the code of the “Evolution of Social Groups” (ESG) algorithm:
1. Init. This method initializes the algorithm parameters and initial population. It also divides the population into groups.
2. Moving. The method is responsible for the initial placement of groups in the search space and agents in groups.
3. Revision. Here the positions of the agents are updated; if there is no improvement, the radius of the group increases, or, on the contrary, decreases. This method also revises the agents' positions and updates the best solution found; if the agent is outside the boundaries, its position is adjusted.
4. SeInDiSp. The method is used to ensure that the value is within a given range. If the value is outside the range, it is adjusted to the nearest limit, respecting the search step.
5. RNDfromCI. This method generates a random number within a given range.
6. Scale. This method scales the input value from one range to another.
7. PowerDistribution. This method is used to generate new agent positions using a power-law distribution.
Below is the code for the Revision method, and all the code in C# with an example of use can be found Here.
public void Revision()
{
for (int i = 0; i < popSize; i++)
{
if (a[i].f > fB)
{
fB = a[i].f;
Array.Copy(a[i].c, cB, a[i].c.Length);
}
}
int cnt = 0;
bool impr = false;
for (int s = 0; s < groups; s++)
{
impr = false;
for (int p = 0; p < gr[s].sSize; p++)
{
if (a[cnt].f > gr[s].fB)
{
gr[s].fB = a[cnt].f;
Array.Copy(a[cnt].c, gr[s].cB, a[cnt].c.Length);
impr = true;
}
cnt++;
}
if (!impr) gr[s].sRadius *= expansionRatio;
else gr[s].sRadius = groupRadius;
if (gr[s].sRadius > 0.5) gr[s].sRadius = 0.5;
}
double coordinate = 0.0;
double radius = 0.0;
double min = 0.0;
double max = 0.0;
cnt = 0;
for (int s = 0; s < groups; s++)
{
for (int p = 0; p < gr[s].sSize; p++)
{
for (int c = 0; c < coords; c++)
{
if (RNDfromCI(0.0, 1.0) < 1.0)
{
radius = (rangeMax[c] - rangeMin[c]) * gr[s].sRadius;
min = gr[s].cB[c] - radius;
max = gr[s].cB[c] + radius;
if (min < rangeMin[c]) min = rangeMin[c];
if (max > rangeMax[c]) max = rangeMax[c];
coordinate = PowerDistribution(gr[s].cB[c], min, max, power);
a[cnt].c[c] = SeInDiSp(coordinate, rangeMin[c], rangeMax[c], rangeStep[c]);
}
}
cnt++;
}
}
cnt = 0;
for (int s = 0; s < groups; s++)
{
for (int c = 0; c < coords; c++)
{
int posSw = (int)RNDfromCI(0, groups);
if (posSw >= groups) posSw = groups - 1;
a[cnt].c[c] = gr[posSw].cB[c];
}
cnt += gr[s].sSize;
}
}
Those who read to the end will be interested in the fact that the algorithm is tested on Rastrigin’s test function, and in the code you can easily replace the function with any other one if desired; it is not part of the class. This algorithm architecture allows you to implement any user applications where optimization is required.
conclusions
The Evolution of Social Groups (ESG) algorithm is an effective optimization method based on the interaction of groups. It is adaptive, diverse and capable of finding optimal solutions to various problems. ESG can be applied in areas where parameter optimization is required, such as machine learning, optimal control, and combinatorial optimization.
The ESG architecture allows you to easily implement different optimization methods, combining their advantages. ESG is a flexible and self-sufficient solution for complex problems.
Features of the ESG algorithm:
Simple architecture and high portability to other programming languages.
High convergence on complex problems.
Extremely fast algorithm with low computational requirements.