Development of a high-performance cache layer based on Redis in a telegram bot
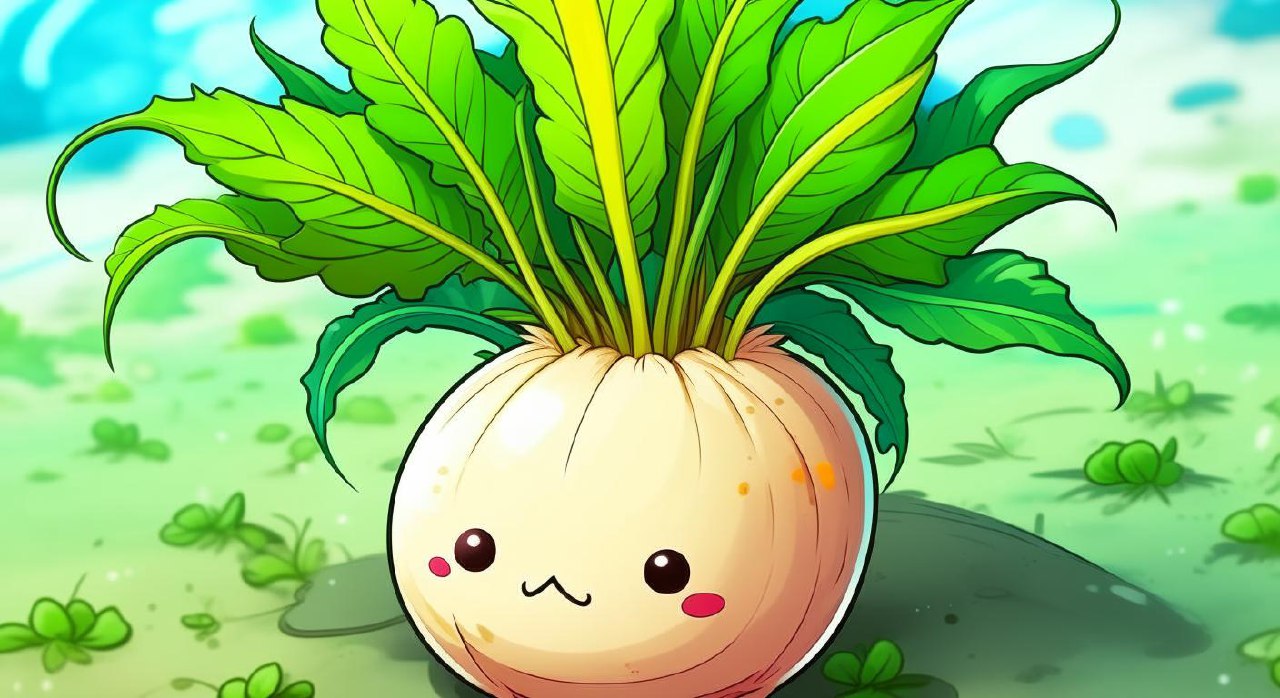
Instantaneous processes have become the gold standard. Users demand instant results, and telegram bots are no exception. Without data caching, bots can experience severe delays when processing requests. Cache layers in tebots are a key tool that can significantly speed up query processing by storing frequently requested data in memory for quick access.
Caching becomes especially important when the bot has many users and processes requests that require access to external resources, such as databases or external APIs.
Redis is a lightning-fast, high-performance in-memory data management system that is ideal for caching in telegram bots. This in-memory database is designed for speed and efficiency, allowing developers to store and retrieve data almost instantly. With Redis, you can store a variety of data such as text responses, images, audio files, and even more complex data structures, all with incredible access speed.
Redis also provides a rich set of features, including transaction support, publish/subscribe, exception management, and automatic data deletion, making it an excellent choice for creating a reliable and scalable cache layer.
Setting up the environment
Before you can start developing a high-performance cache layer based on Redis, you need to install and configure Redis on your server. Redis can be easily installed on most popular operating systems, including Linux, Windows and macOS:
Installing Redis:
For Linux users, you can install Redis through your distribution’s package manager. For example, on Ubuntu, run the following commands:
sudo apt update sudo apt install redis-server
If you are using Windows, you can download Redis from the official website and follow the installation instructions.
Starting Redis:
Configuration setup:
Redis has a configuration file, usually located at
/etc/redis/redis.conf
in Linux. You can configure parameters such as port, password and directory to store data in this file.
Check the server status:
You can verify that Redis is working by running the command:
redis-cli ping
If the server is running, you should see a “PONG” response.
To interact with Redis and your telegram bot, you need to create a bot and get an API token from BotFather in Telegram. This is the first step in creating your telegram bot. Next, you can use a Python library such as telebot
to control your bot.
An example of creating a bot using telebot
:
import telebot
# Замените 'YOUR_API_TOKEN' на реальный API-токен вашего бота
bot = telebot.TeleBot('YOUR_API_TOKEN')
# Пример обработчика команды /start
@bot.message_handler(commands=['start'])
def handle_start(message):
bot.send_message(message.chat.id, "Привет! Я ваш телеграм-бот.")
# Запуск бота
bot.polling()
Now that you have Redis and your telegram bot, you must configure their interaction. Redis provides a set of commands for storing and retrieving data that can be easily integrated into the bot. Here’s how to do it:
Connecting to Redis:
Enable Redis support in your Python project using the library
redis-py
:import redis r = redis.Redis(host="localhost", port=6379, db=0)
Saving data in Redis:
Use the command
set
to save data in Redis:r.set('ключ', 'значение')
Extracting data from Redis:
Your bot can now store and retrieve data from Redis for faster access and improved performance.
Development of the cache layer architecture
Redis is about choosing a suitable data structure to store information. Redis provides a variety of data types, each with its own unique features and benefits. Your choice will depend on the specifics of your telegram bot and data storage requirements.
Strings:
Strings are suitable for storing text data such as messages, images, audio files and other binary data. You can use the commands
SET
AndGET
to save and retrieve data. Example:r.set('user:123:avatar', 'avatar.jpg') avatar = r.get('user:123:avatar')
Hashes:
Hashes can be useful for storing structured data such as user profiles or configuration parameters. You can use the commands
HSET
AndHGET
to store and retrieve hash fields. Example:r.hset('user:123', 'name', 'John') name = r.hget('user:123', 'name')
Lists:
Lists are good for storing ordered data, such as message history. You can use the commands
LPUSH
AndLRANGE
to add and remove items from a list. Example:r.lpush('chat:456:messages', 'Hello') messages = r.lrange('chat:456:messages', 0, -1)
Sets:
Sets can be used to store unique values, such as the IDs of users in a group. You can use the commands
SADD
AndSMEMBERS
to add and remove elements from a set. Example:r.sadd('group:789:members', 'user:123') members = r.smembers('group:789:members')
Sorted Sets:
Ordered sets are suitable for storing rated or timestamped data, such as ratings or events in chronological order. You can use the commands
ZADD
AndZRANGE
for working with ordered sets. Example:r.zadd('leaderboard', {'user:123': 100, 'user:456': 80}) top_scores = r.zrange('leaderboard', 0, 2, withscores=True)
Choosing the right data structure in Redis is a key step when designing your cache layer, and it should fit the specific needs of your bot.
Having defined the data structure, the next step is to design the keys and data schema to store the information in Redis. Your Redis keys should be well organized and have a clear structure to make it easy to find and update data.
Key prefixes:
Use key prefixes to categorize data. For example, if you are storing information about users, you could use the prefix
user:123
for all data associated with user ID 123.
Using variables in keys:
Include variables in keys for various queries. For example, for a chat message history cache, the key may contain the chat ID:
chat:456:messages
.
Key expiration dates:
Consider the lifetime of keys so that data does not remain in Redis indefinitely. Redis allows you to set lifetimes for keys. Example:
r.setex('user:123:session', 3600, 'active')
In this case, the key
user:123:session
exists only for one hour.
Update keys:
Please consider how the data will be updated. If the data changes externally (for example, in the database), update the corresponding key in Redis to reflect the current state.
There are also several methods you can use in Redis to provide security and restrict access.
Access password:
Set a Redis server access password in the configuration to prevent unauthorized access. You can use the command
AUTH
for authentication.
Networks and privileges:
Redis configuration options allow you to restrict server access to specific networks only. This can be useful for isolating the Redis server from public networks.
Serialization and encryption:
When storing sensitive data, consider serializing and encrypting it before storing it in Redis. This will help protect your data from unauthorized access.
Application level access control:
For more detailed access control, you can implement an authorization and authentication system in your telegram bot, excluding access to data if the user does not have the appropriate rights.
Securing Redis is an important task that should be given special attention when designing a bot cache layer, especially if the data you store is sensitive or sensitive.
Working with data in Redis
Saving data in Redis is a key step in developing a cache layer for a telegram bot. Redis provides many commands for storing data in various data structures.
Saving Strings:
To save text data, images, audio files and other binary data, use the command
SET
. Example:r.set('user:123:avatar', 'avatar.jpg')
Saving hashes (Hashes):
To store structured data such as user profiles, use the command
HSET
. Example:r.hset('user:123', 'name', 'John')
Saving Lists:
To save organized data such as message history, use the command
LPUSH
. Example:r.lpush('chat:456:messages', 'Hello')
Saving Sets:
To store unique values, such as user IDs in a group, use the command
SADD
. Example:r.sadd('group:789:members', 'user:123')
Saving in Sorted Sets:
To store rating data, such as ratings, use the command
ZADD
. Example:r.zadd('leaderboard', {'user:123': 100, 'user:456': 80})
When storing data in Redis, consider the requirements of your telegram bot and the specifics of the data you store in the cache layer.
Redis provides a variety of commands to retrieve data from various structures. Here’s how to do it:
Extracting Strings:
To get text data or binary files, use the command
GET
. Example:avatar = r.get('user:123:avatar')
Retrieving hashes:
To get structured data, use the command
HGET
. Example:name = r.hget('user:123', 'name')
Extracting from Lists:
To get ordered data, use the command
LRANGE
. Example:messages = r.lrange('chat:456:messages', 0, -1)
Extracting from Sets:
To get unique values, use the command
SMEMBERS
. Example:members = r.smembers('group:789:members')
Extracting from Sorted Sets:
To get data with ratings, use the command
ZRANGE
. Example:top_scores = r.zrange('leaderboard', 0, 2, withscores=True)
When extracting data from Redis, please note that the data can be in different formats; they must be interpreted correctly in your telegram bot.
Updating data in Redis and resetting it is an equally important task when working with the cache layer. Redis provides commands for updating and deleting data:
Data update:
To update existing data, use the appropriate commands. For example, to update a hash value:
r.hset('user:123', 'name', 'NewName')
Deleting data:
To remove data from Redis, use the command
DEL
. For example, to delete a keyuser:123:avatar
:r.delete('user:123:avatar')
Key expiration:
If you want the key to be automatically deleted after a certain time, you can use the commands
EXPIRE
orEXPIREAT
:r.expire('user:123:session', 3600) # Устанавливает срок действия в 1 час
Refreshing and flushing data in Redis is an important part of keeping the data in your cache layer up to date. Also remember to regularly clean up stale data to avoid cluttering Redis memory with unnecessary information.
Performance optimization
Pipelines are a powerful tool for optimizing your experience with Redis. They allow you to group multiple Redis commands and send them in one request. This reduces the delay between sending and receiving responses to each command, making your system more productive.
An example of using a pipeline with a library redis-py
:
# Создание объекта пайплайна
pipeline = r.pipeline()
# Добавление команд в пайплайн
pipeline.set('user:123:name', 'John')
pipeline.set('user:123:age', 30)
pipeline.get('user:123:name')
pipeline.get('user:123:age')
# Выполнение пайплайна и получение результатов
results = pipeline.execute()
# Результаты содержат значения полученных ключей
name = results[2]
age = results[3]
Using pipelines is especially useful when you need to perform multiple Redis operations sequentially, as it reduces network latency.
To optimize performance, you should minimize the number of requests to Redis. One way to do this is to cache data in application memory and only make requests to Redis when necessary.
Example of data caching in Python using variables:
# Кэширование данных в переменных
cached_data = {}
def get_data_from_cache_or_redis(key):
if key in cached_data:
return cached_data[key]
else:
data = r.get(key)
cached_data[key] = data
return data
This approach reduces the load on Redis and reduces latency, since many requests will be satisfied from the application’s in-memory cache.
To maintain high performance of your telegram bot with a Redis cache layer, it is important to have a monitoring and profiling system. This will allow you to identify bottlenecks and performance issues.
Error handling and recovery
When developing a Redis-based cache layer for a telegram bot, it is necessary to pay attention to error handling and recovery mechanisms to ensure reliable operation of the system.
Working with Redis may cause various exceptions such as connection loss, timeout, authentication error and others. Good exception handling ensures the stability of your bot. Here is an example of how you can manage exceptions when working with the library redis-py
:
import redis
import telebot
try:
r = redis.StrictRedis(host="localhost", port=6379, db=0, password='your_password')
bot = telebot.TeleBot('your_bot_token')
# Ваш код для взаимодействия с Redis
except redis.exceptions.ConnectionError:
print("Ошибка подключения к Redis.")
except redis.exceptions.TimeoutError:
print("Превышено время ожидания при работе с Redis.")
except redis.exceptions.AuthenticationError:
print("Ошибка аутентификации при подключении к Redis.")
except Exception as e:
print(f"Произошла неизвестная ошибка: {e}")
Exception management helps prevent accidents and gives you the ability to respond appropriately to errors.
When Redis crashes, it is important to have recovery mechanisms in place so that your telegram bot continues to work without significant interruptions. Here’s what might help:
Retrays for errors:
In case of an error when performing an operation in Redis, you can implement a retry mechanism (retrying the operation). This may help with temporary glitches.
Using replication:
Redis supports replication, which allows you to back up your data. If the main server fails, the replica can become the main one and ensure continuous operation.
Using a Redis cluster:
Redis Cluster provides a mechanism for horizontal scaling and fault tolerance. Deploy a cluster to reduce the risk of failures.
Monitoring and alerts:
Use monitoring systems to quickly find out about Redis failures and problems. Set up alerts to be notified of problems in real time.
To ensure data security in Redis, you need to create regular backups. Redis provides the command SAVE
to create a point backup and the command BGSAVE
to create a background backup. Back up copies of your data regularly and store them in a safe place.
An example of creating a data backup using BGSAVE
:
r.bgsave()
To restore data from a backup, use the command BGRESTORE
:
r.bgrestore('your_backup_file')**VII. Расширение функциональности**
При разработке кеш-слоя на основе Redis для телеграм-бота, вы можете уйти дальше, чем простое кэширование текстовых данных. В этом разделе рассмотрим, как расширить функциональность кеш-слоя, работать с многозначными ключами и интегрировать Redis с другими сервисами.
*A. Кэширование не только текстовых данных*
Хотя кэширование текстовых данных может быть весьма полезным, Redis позволяет хранить и кэшировать разнообразные типы данных, включая числа, списки, множества и другие. Это особенно полезно, если ваш телеграм-бот работает с разнообразными данными. Вот как это можно сделать:
1. **Кэширование чисел:**
Redis может хранить целые и числа с плавающей запятой. Например, вы можете кэшировать вычисленные результаты или статистику:
```python
r.set('total_users', 1000)
```
2. **Кэширование списков и множеств:**
Redis поддерживает кэширование упорядоченных данных. Вы можете использовать команды `LPUSH`, `RPUSH`, `SADD` и другие для кэширования списков и множеств:
```python
r.lpush('recent_posts', 'post1')
r.sadd('online_users', 'user123')
```
3. **Кэширование бинарных данных:**
Redis может хранить бинарные данные, что полезно для кэширования изображений, аудиофайлов и других бинарных данных:
```python
r.set('image:123', b'\x89PNG\r\n...')
```
Кэширование разнообразных данных позволяет использовать Redis для различных целей, не ограничиваясь только текстовыми данными.
*B. Работа с многозначными ключами и наборами данных*
Вместо хранения данных в виде отдельных ключей, Redis предоставляет структуры данных для организации множества значений, что может быть полезным при работе с коллекциями данных:
1. **Многозначные ключи (Hashes):**
Redis предоставляет структуру данных "хэш", где каждый ключ может содержать несколько полей и их соответствующих значений. Это полезно, например, при хранении данных о профилях пользователей:
```python
r.hset('user:123', 'name', 'John')
r.hset('user:123', 'age', 30)
```
2. **Списки (Lists) и Множества (Sets):**
Redis также поддерживает списки и множества, которые могут использоваться для хранения упорядоченных данных или уникальных значений. Например, вы можете использовать список для хранения истории действий пользователя:
```python
r.lpush('user:123:actions', 'action1')
```
3. **Упорядоченные множества (Sorted Sets):**
Упорядоченные множества могут использоваться для хранения данных с оценками, таких как рейтинги или ранжирование:
```python
r.zadd('leaderboard', {'user:123': 100, 'user:456': 80})
```
Используйте структуры данных Redis в зависимости от вашего конкретного случая использования, чтобы эффективно хранить и извлекать данные.
*C. Интеграция с другими сервисами*
Redis можно интегрировать с другими сервисами, чтобы расширить функциональность вашего телеграм-бота. Например:
1. **Интеграция с базой данных:**
- Вы можете использовать Redis для кэширования данных из вашей базы данных, уменьшая нагрузку на базу данных и ускоряя доступ к данным.
2. **Интеграция с очередями сообщений:**
- Redis может быть использован для создания очередей сообщений, что полезно, например, при обработке асинхронных задач в вашем боте.
3. **Интеграция с поисковыми движками:**
- Redis может служить для кэширования и индексации данных для поиска.
Интеграция Redis с другими сервисами позволяет создать мощную инфраструктуру для вашего телеграм-бота и расширить его функциональность.
Расширение функциональности вашего кеш-слоя Redis позволяет вам эффективно управлять разнообразными данными и интегрировать Redis в вашу архитектуру бота для обеспечения более широкого спектра функций.
Data protection and recovery is a key component of your system’s resilience. Develop a data backup and recovery plan to minimize information loss when Redis fails.
Expansion of functionality
While caching text data can be quite useful, Redis allows you to store and cache a variety of data types, including numbers, lists, sets, and others. This is especially useful if your telegram bot works with a variety of data. Here’s how to do it:
Number caching:
Redis can store integers and floating point numbers. For example, you can cache calculated results or statistics:
r.set('total_users', 1000)
Caching lists and sets:
Redis supports caching of ordered data. You can use the commands
LPUSH
,RPUSH
,SADD
and others for caching lists and sets:r.lpush('recent_posts', 'post1') r.sadd('online_users', 'user123')
Caching binary data:
Redis can store binary data, which is useful for caching images, audio files and other binary data:
r.set('image:123', b'\x89PNG\r\n...')
Caching a variety of data allows Redis to be used for a variety of purposes, not just limited to text data.
Instead of storing data as individual keys, Redis provides data structures to organize multiple values, which can be useful when working with collections of data:
Multivalued keys (Hashes):
Redis provides a hash data structure where each key can contain multiple fields and their corresponding values. This is useful, for example, when storing user profile data:
r.hset('user:123', 'name', 'John') r.hset('user:123', 'age', 30)
Lists and Sets:
Redis also supports lists and sets, which can be used to store ordered data or unique values. For example, you can use a list to store a history of user actions:
r.lpush('user:123:actions', 'action1')
Sorted Sets:
Ordered sets can be used to store value-based data, such as ratings or rankings:
r.zadd('leaderboard', {'user:123': 100, 'user:456': 80})
Use Redis data structures depending on your specific use case to store and retrieve data efficiently.
Redis can be integrated with other services to expand the functionality of your telegram bot. For example:
Database integration:
You can use Redis to cache data from your database, reducing database load and speeding up data access.
Integration with message queues:
Redis can be used to create message queues, which is useful, for example, when processing asynchronous tasks in your bot.
Integration with search engines:
Integrating Redis with other services allows you to create a powerful infrastructure for your telegram bot and expand its functionality.
Extending the functionality of your Redis cache layer allows you to efficiently manage a variety of data and integrate Redis into your bot architecture to provide a wider range of functionality.
Implementation example
Example 1:
Let’s imagine that you have a telegram bot that provides information about the weather in various cities. To speed up access to weather data, you decide to use Redis as a cache layer. An example of integration of Redis and a telegram bot for caching weather data:
import telebot
import redis
import requests
# Инициализация телеграм-бота
bot = telebot.TeleBot('your_bot_token')
# Инициализация Redis
r = redis.StrictRedis(host="localhost", port=6379, db=0)
# Функция для получения погодных данных
def get_weather(city):
# Сначала проверим, есть ли данные в Redis
cached_data = r.get(f'weather:{city}')
if cached_data:
return cached_data.decode('utf-8')
else:
# Если данных нет в кеше, запросим их у внешнего API (например, OpenWeatherMap)
api_key = 'your_api_key'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
weather_description = data['weather'][0]['description']
temperature = data['main']['temp']
result = f'Погода в {city}: {weather_description}, Температура: {temperature}°C'
# Сохраняем полученные данные в Redis на 10 минут
r.setex(f'weather:{city}', 600, result)
return result
else:
return 'Не удалось получить данные о погоде'
# Обработка команды /weather
@bot.message_handler(commands=['weather'])
def send_weather(message):
city = message.text.replace('/weather', '').strip()
if city:
weather_data = get_weather(city)
bot.reply_to(message, weather_data)
else:
bot.reply_to(message, 'Пожалуйста, укажите город, для которого вы хотите узнать погоду.')
# Запуск бота
bot.polling()
In this example, the bot accepts the command /weather
with the name of the city. It first checks if there is weather data for that city in Redis. If there is data, the bot sends it to the user. If there is no data, the bot makes a request to the weather API, gets the data, stores it in Redis and then sends it to the user. Thus, the data is cached in Redis, which reduces the load on the external API and speeds up responses to user requests.
Example 2:
Let’s imagine that we have a telegram bot that provides information about films. We want to speed up access to popular movies by caching information about them using Redis.
import telebot
import requests
import redis
# Инициализация бота
bot = telebot.TeleBot('YOUR_BOT_TOKEN')
# Инициализация Redis
r = redis.StrictRedis(host="localhost", port=6379, db=0, decode_responses=True)
# Функция для получения информации о фильме из кэша или API
def get_movie_info(movie_id):
# Проверяем, есть ли информация о фильме в кеше Redis
cached_info = r.get(f'movie:{movie_id}')
if cached_info:
return cached_info
else:
# Если информации нет в кеше, запрашиваем ее у внешнего API
api_url = f'https://api.themoviedb.org/3/movie/{movie_id}'
api_params = {'api_key': 'YOUR_API_KEY'}
response = requests.get(api_url, params=api_params)
if response.status_code == 200:
# Получаем информацию о фильме
movie_info = response.json()
# Кэшируем информацию в Redis на 1 час
r.setex(f'movie:{movie_id}', 3600, movie_info)
return movie_info
else:
return None
# Обработчик команды /movie
@bot.message_handler(commands=['movie'])
def handle_movie_command(message):
# Получаем аргумент команды, который является идентификатором фильма
movie_id = message.text.split(' ')[1]
# Получаем информацию о фильме
movie_info = get_movie_info(movie_id)
if movie_info:
# Отправляем информацию о фильме пользователю
bot.send_message(message.chat.id, f"Название: {movie_info['title']}\n"
f"Описание: {movie_info['overview']}\n"
f"Рейтинг: {movie_info['vote_average']}")
else:
bot.send_message(message.chat.id, "Фильм не найден.")
# Запускаем бот
bot.polling()
In this example, we use Redis to cache movie information. When a user sends a command /movie
, we first check if the movie information is in the Redis cache. If the information is there, we get it from the cache and send it to the user. If the information is not in the cache, we make a request to an external API to obtain information about the movie, cache it in Redis for 1 hour and send it to the user. This helps reduce the load on the external API and speeds up data access for users.
Conclusion
Redis is a powerful tool for creating high-performance telegram bots, and knowing its capabilities will help you make your projects even more successful.
My colleagues from OTUS tell more useful information about application infrastructure in the framework of professional online courses. Each course has a number of free lessons that anyone can register for, here are the closest ones:
More free events available find in calendar.