Developing an Interface for a ResNet50() CNN Model with Keras
Greetings to all! My name is Soso and I want to share with you my journey into the world of machine learning and deep learning using TensorFlow and Keras. In this article, we will look at the process of classifying dog breeds from images using neural networks. Let’s explore how these powerful tools can help us automatically recognize dog breeds in photos.
Machine learning is one of the most exciting areas in modern data science. It allows computers to learn from experience and data, and is capable of performing complex tasks, including image classification. Image classification is the process of determining the category or class that each image belongs to.
For our dog breed classification project, we have chosen TensorFlow and Keras as our main tools. TensorFlow is a powerful and flexible platform for developing and training neural networks, while Keras provides a convenient and high-level interface for building deep learning models.
Preparing the environment and loading data
The first step is to install TensorFlow and Keras with the following commands:
pip install tensorflow
With the required packages installed, we proceed to set up our production environment by importing the required libraries and checking the TensorFlow version.
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions
from tensorflow.keras.preprocessing import image
import numpy as np
import matplotlib.pyplot as plt
print(tf.__version__)
Loading and preprocessing images
First, let’s load the image we’ll be working with:
from PIL import Image
img_path = "./samples/dog_1100x628.jpg"
img = np.asarray(Image.open(img_path))
plt.imshow(img)
plt.show()
Next, resize the image to 224×224 pixels, since most deep learning models expect this size:
img = image.load_img(img_path, target_size=(224, 224))
plt.imshow(img)
plt.show()
We will also convert the image to an array and create a batch from one image:
img_array = image.img_to_array(img)
print(img_array.shape)
img_batch = np.expand_dims(img_array, axis=0)
Models perform well when they receive data in a constant range. In this case, the image pixel values range from 0 to 255. So, if we run the preprocess_input() function from Keras on the input images, we will normalize each pixel to the standard range.
img_preprocessed = preprocess_input(img_batch)
print(img_preprocessed .shape)
For our project, we chose a pre-trained ResNet-50 model. Here is how we can load the model:
tf.keras.applications.resnet50.ResNet50(
include_top=True,
weights="imagenet",
input_tensor=None,
input_shape=None,
pooling=None,
classes=1000
)
model = tf.keras.applications.resnet50.ResNet50()
Table of parameters of the trained model:
model = tf.keras.applications.resnet50.ResNet50()
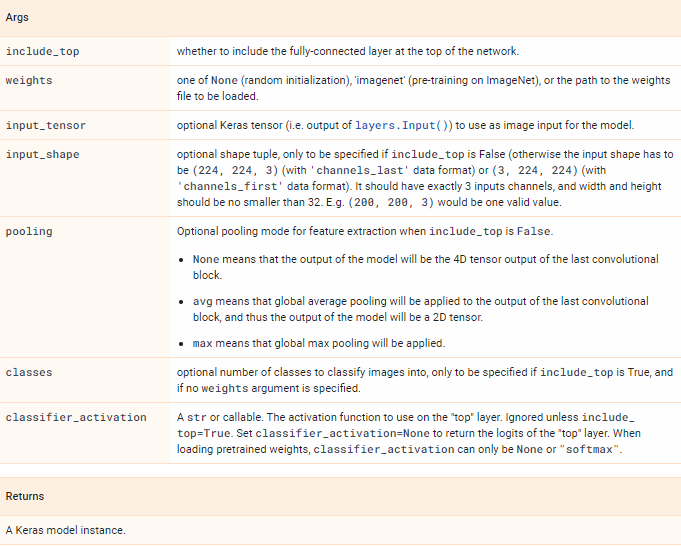
Run pretrained model (twice)
prediction = model.predict(img_preprocessed)
Application of the model and output of results
Now we can predict the dog breed based on our image:
print(decode_predictions(prediction, top=3)[0])
print(tf.keras.applications.imagenet_utils.decode_predictions(prediction, top=5))
Classification result output parameter table:
model = tf.keras.applications.resnet50.ResNet50()

After running the code, we will get the probabilities for the three most likely dog breeds found in the image.
The predicted categories for this image are different types of dogs:
golden_retriever -0.7459664;
vizsla-0.06819201;
flat-coated_retriever -0.041535184;
Chesapeake_Bay_retriever-0.035749573;
Labrador_retriever-0.018360337.
The entire program
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions
from tensorflow.keras.preprocessing import image
# Helper libraries
import numpy as np
import matplotlib.pyplot as plt
def classify(img_path):
img = image.load_img(img_path, target_size=(224, 224))
img_array = image.img_to_array(img)
img_batch = np.expand_dims(img_array, axis=0)
img_preprocessed = preprocess_input(img_batch)
model = tf.keras.applications.resnet50.ResNet50()
prediction = model.predict(img_preprocessed)
print(decode_predictions(prediction, top=3)[0])
classify("./samples/dog_1100x628.jpg")
We can Design the visual interface of the ResNet50() CNN Keras model using the Tkinter library. This unique the code allows us to recognize the object shown in the photo by simply selecting the image through the GUI.
from tkinter import *
from tkinter.filedialog import *
from tkinter.messagebox import *
from tkinter.ttk import Combobox
from tkinter.messagebox import showerror
from PIL import Image, ImageTk
import pandas as pd
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions
from tensorflow.keras.preprocessing import image
import numpy as np
import warnings
warnings.simplefilter(action='ignore', category=FutureWarning)
def start_image_fure():
try:
url=askopenfilename()
img=Image.open(url)
img=image.load_img(url, target_size=(224, 224))
render=ImageTk.PhotoImage(img, master=tk)
lab=Label(tk,image=render)
lab.image=render
lab.grid(row=0,column=0)
img_array=image.img_to_array(img)
img_batch=np.expand_dims(img_array, axis=0)
img_preprocessed=preprocess_input(img_batch)
model=tf.keras.applications.resnet50.ResNet50()
prediction=model.predict(img_preprocessed)
info.set(decode_predictions(prediction, top=5)[0][0][1:])
except:
showerror(title="Error", message="File opening error")
def close_win():
tk.destroy()
tk=Tk()
tk.title("Visual interface of the ResNet50 model")
main_menu=Menu(tk)
tk.config(menu=main_menu)
file_menu=Menu(main_menu)
main_menu.add_cascade(label="MENU", menu=file_menu)
file_menu.add_command(label="Open Image", command= start_image_fure)
file_menu.add_command(label="Exit", command= close_win)
info=StringVar(tk)
lab_1=Label(tk, textvariable=info, font=("Arial", 12, "bold "),foreground='black')
lab_1.grid(row=0,column=1)
x=(tk.winfo_screenwidth()-tk.winfo_reqwidth())/4 #центрирование формы
y=(tk.winfo_screenheight()-tk.winfo_reqheight())/16 #центрирование формы
tk.wm_geometry("+%d+%d" % (x, y))#центрирование формы
tk.geometry('550x280')
tk.mainloop()



This code creates a GUI window where you can select an image using the “Open Image” button. After selecting an image, it is displayed in the window and the ResNet50 model automatically classifies the object in the image. The classification result is displayed next to the image.
Tkinter gives us the ability to create simple graphical interfaces to interact with deep learning models and makes it easy to use the models in real time. This is a great way to present classification results and make them more accessible to a wider audience.
By using this code in combination with the previous steps, you will be able to create a complete dog breed classification project using neural networks and provide a user friendly visual interface. This can be especially useful when you want to demonstrate the capabilities of a model or integrate it into an application or web page.
conclusions
In this article, we looked at the dog breed classification process using TensorFlow and Keras. We installed the necessary packages, loaded and pre-processed the image, selected the pre-trained ResNet-50 model, and got the classification results. Machine learning and deep learning provide amazing opportunities in the field of object recognition in images, and I hope that this project will inspire you to explore and experiment on your own.
Additional Resources