Deploy the application to the server via push in Git. Or how to transfer a website or bot to hosting in 3 steps
Once a project is created, it needs to be hosted somewhere so that it runs 24/7. In this article we will study two ways to deploy an application to a cloud server. In the first scenario, we will look at deployment by pushing code to a dedicated Git repository. In the second scenario, we will perform a similar operation, but we will upload the files through the GUI. Both methods require deployment in literally 5-10 minutes.
As an example application, let’s take the API for TODO notes in Python. You can use this method to transfer any of your websites or bots written in Java, Node.JS, etc. to hosting.
Here is the code for the application itself.
import os
import json
from flask import Flask, request, abort
from flask_cors import CORS
FILENAME = "/data/todo.json" if "AMVERA" in os.environ else "todo.json"
def get_data():
try:
with open(FILENAME, "r", encoding="utf-8") as f:
return json.load(f)
except FileNotFoundError:
return []
def save_data(data):
with open(FILENAME, "w", encoding="utf-8") as f:
json.dump(data, f)
app = Flask(__name__)
cors = CORS(app)
@app.route("/")
def index():
return "TODO App"
@app.route("/todo")
def get_all_todo():
return get_data()
@app.route("/todo/<int:id>")
def get_single_todo(id):
data = get_data()
if id < 0 or id >= len(data):
abort(404)
return data[id]
@app.route("/todo", methods=["POST"])
def add_new_todo():
new_todo = request.json
if new_todo is None:
abort(400)
data = get_data()
data.append(new_todo)
save_data(data)
return "OK", 201
@app.route("/todo/<int:id>", methods=["PUT"])
def update_todo(id):
data = get_data()
if id < 0 or id >= len(data):
abort(404)
updated_todo = request.json
if updated_todo is None:
abort(400)
data[id] = updated_todo
save_data(data)
return "OK"
if __name__ == "__main__":
app.run(port=8080)
Preliminary preparation for application deployment
To deploy the application we will use a specialized Russian cloud Amvera Cloud. First, register in it and click the “Create project” button. After registration, a starting balance (111 rubles) will appear on your balance, which will be enough for almost 3 weeks of operation of a small application. The balance will begin to be charged at a per-minute rate only when you deploy the application. But you can use other services with a GitOps approach, for example Heroku. The deployment mechanics will be similar.
Step 1 – Create a Dependency List File
Since our application is written in Python, let’s create a file with the dependencies we use. It is important to list all the libraries you have installed via pip, but you don’t need to list the standard libraries.
In the example we are using Flask and Gunicorn and our requirements.txt will look like this
Flask==2.2.2
Flask-CORS==3.0.10
gunicorn==20.1.0
Place the file at the root of the project
Step 2 – creating a configuration file
In order for the system to understand what exactly we are deploying and with what parameters, we need to write a configuration file.
The configuration file can be amvera.yml (for a limited number of environments) or dockerfile (for arbitrary environments). In our example we need amvera.yml. To create it, we’ll use generator.

The resulting yaml
meta:
environment: python
toolchain:
name: pip
run:
command: gunicorn --bind 0.0.0.0:5000 app:app
containerPort: 5000
Add the yaml file to the project root
Step 3 – transfer the site to hosting. Deploying an application via push to Git
For deployment applications, we will link our own repository or use the dedicated Amvera Git repository.
To do this, run the command in the project root: git init if the repository has not yet been initiated.
We link local and remote repositories using the command
git remote add amvera https://git.amvera.ru/ваш_юзернейм/ваш_проект
Sending commands
git add .
git commit -m "Initial commit"
git push amvera master (нужно авторизоваться используя учетные данные сервиса).
If all actions are completed successfully, you will see the status “Deployed successfully”

And if an error is observed, it can be tracked in the logs

Method number 2 – deploy the application via a graphical interface
An alternative way is to deploy by uploading files through the GUI. In some cases, this method of transferring a site to hosting is more intuitive. But with frequent updates, this path can be more labor-intensive than using Git commands in the console.
Below is a video example of deploying an application through the interface.
Step 1
Upload project files and a file with dependencies using the button in the “Repository” tab
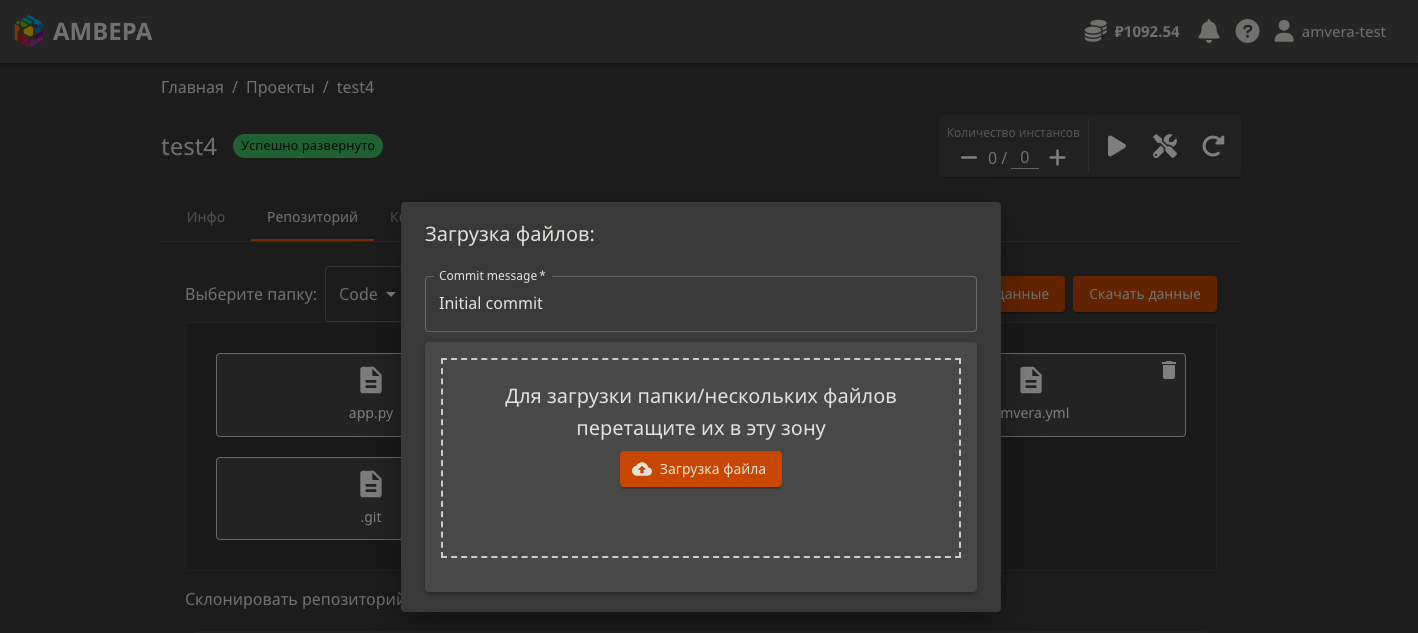
To see what exactly has loaded, you need to exit the window and wait a little (sometimes you need to reload the browser page).
Step 2
Go to the configuration tab and set the parameters similar to the yml generator.

If you have previously uploaded a yaml file through the Repository, its content will be automatically updated.
Next, click the buttons “Apply” (adds the configuration file to the root of the repository) and “Build” (starts the build).
Then we just wait about 5 minutes while the application is assembled and deployed on the server. If all is well, the project will move to the “Successfully Deployed” status. Let’s check if everything works correctly. If something doesn’t work, look at the errors in the logs in your personal account.
In total, we looked at 2 of the simplest ways to deploy an application to a server. In both cases, most of the time is spent on understanding the process a little for the first time and correctly specifying dependencies and parameters in the configuration file (port, paths, etc.). Subsequently, both methods make it possible to deploy updates in literally 1 minute.