Code analysis in Flutter applications and setting up a Gitlab CI assembly line for analysis
When changes per task exceed 7+-2 files, it becomes difficult to control the quality of the code. Then static code analyzers come to the rescue. A static analyzer is popular in the dart community dart_code_metrics. As part of this tutorial, you will learn what it is and how to set it up in a project and implement it in gitlab-ci to automatically run checks, for example, before code review or just before applying changes to the main branches.
Brief overview of the analyzer
The dart_code_metrics analyzer allows you to capture such code metrics as: cyclomatic complexity, holstead metrics, number of lines of code, maintainability index, and more. In general, these metrics will allow you to accurately say what state your project is in and help you adjust the timing of the implementation of any feature.
In addition to code metrics, dart_code_metrics can create rules for writing code. For example, dart_code_metrics helps prevent memory leaks:

In addition, the rules will help make the project safer with respect to null safety and prevent unexpected errors:
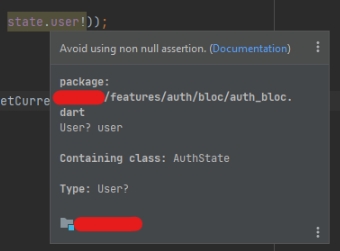
There are a lot of different rules in dart_code_metrics, which you can find in their documentation.
Code metrics and rules are configured in the analysis_options.yaml file. Example configuration file for dart_code_metrics:
Listing 1
analyzer:
plugins:
- dart_code_metrics
dart_code_metrics:
metrics-exclude:
- test/**
metrics:
cyclomatic-complexity: 20
number-of-parameters: 4
maximum-nesting-level: 5
rules-exclude:
- ./**/*.g.dart
rules:
- avoid-double-slash-imports
- avoid-duplicate-exports
- avoid-dynamic
- avoid-nested-conditional-expressions
This way you can add/change rules and metrics.
Setting dart_code_metrics in a project
First you need to add the dart_code_metrics dependency to the project:
Listing 2
flutter pub add --dev dart_code_metrics
flutter pub get
Then you need to configure the analyzer in the analysis_options.yaml file located in the project root. Right now I’m using the following configuration:
Listing 3
include: package:flutter_lints/flutter.yaml
linter:
rules:
analyzer:
plugins:
- dart_code_metrics
dart_code_metrics:
rules-exclude:
- ./**/*.g.dart
rules:
- avoid-double-slash-imports
- avoid-duplicate-exports
- avoid-dynamic
- avoid-nested-conditional-expressions
- avoid-non-null-assertion
- avoid-redundant-async
- avoid-unnecessary-conditionals
- avoid-unnecessary-type-assertions
- avoid-unnecessary-type-casts
- avoid-unrelated-type-assertions
- list-all-equatable-fields
- no-boolean-literal-compare
- prefer-enums-by-name
- prefer-first
- prefer-last
- prefer-immediate-return
- prefer-match-file-name
- prefer-moving-to-variable
- avoid-cascade-after-if-null
- binary-expression-operand-order
- double-literal-format
- no-boolean-literal-compare
- no-empty-block
- no-equal-then-else
- no-object-declaration
- prefer-conditional-expressions
- prefer-trailing-comma
- always-remove-listener
- avoid-returning-widgets
- avoid-shrink-wrap-in-lists
- prefer-correct-edge-insets-constructor
After you have configured the analyzer, restart the IDE and you will be able to observe new hints and notes. If you don’t see anything, you may have made a mistake while editing the analysis_options.yaml file. Also make sure you don’t forget to include dart_code_metrics in the list of parser plugins. Perhaps the problem may be related to the IDE itself, for example, for Android Studio it helps to change some file and wait or restart.
Setting up gitlab-ci for analysis in a repository
You can run the code analyzer in gitlab. This greatly saves the time of the code auditor, because the programmer will immediately see his mistakes before submitting the code for code review, even if the changes contain a large amount of code.
You can see the code comments in the pull request, it looks like this:

In order to run the analyzer, you need a runner. To do this, you need a computer with access to the network, on which the analysis will be performed. Gitlab sends a task to the runner and it runs on the machine.
You can view the list of runners for your project on the Repository > Settings > CI/CD page.
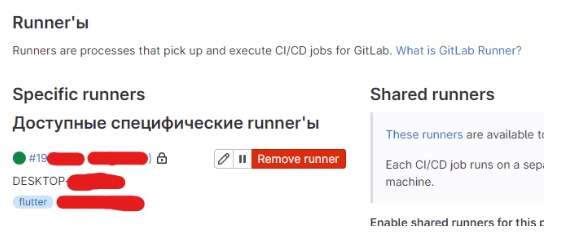
On the page https://docs.gitlab.com/runner/install/ you can see how to install runner on computer. On the page with runners you can also download the necessary installation files and keys.

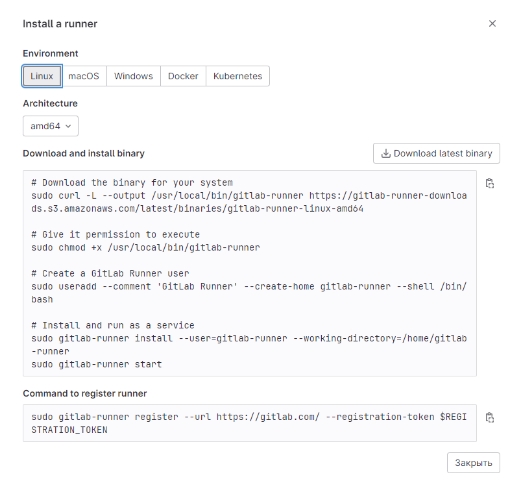
After configuring the runner, you can move on to configuring the pipelines. Pipeline is a set of stages and a description of the actions that need to be performed automatically. The same actions will be performed in runner.
To set up a pipeline in the root of the project, you need to create a .gitlab-ci.yml file. An example configuration file, you can use it in your project:
Listing 4
stages:
- code_quality
…
code_quality:
stage: code_quality
image: "cirrusci/flutter:3.3.0"
before_script:
- flutter pub get
- flutter pub global activate dart_code_metrics
- export PATH="$PATH:$HOME/.pub-cache/bin"
tags:
- flutter
script:
- flutter pub run dart_code_metrics:metrics analyze lib -r gitlab --no-verbose --no-congratulate > gl-code-quality-report.json || exit_code=$?
artifacts:
reports:
codequality: gl-code-quality-report.json
...
Please note that I assigned the flutter tag to the stage. The runner you installed should have the same tag. If your pipeline does not start, this may be the case.
If you did everything right, you will have a pipeline in your pull request:
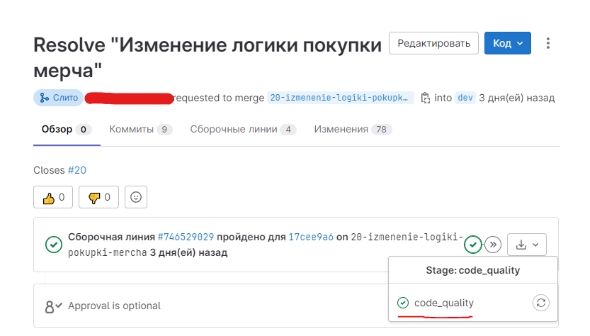
Accordingly, after execution, you will have the results of the analysis. Before that, the page should be reloaded.
Note that the results of the analysis are compared to the master branch. If you set up the analyzer for the first time in your branch, you will be shown all the comments that are in the project. After the analyzer is executed on the main branch, the check results of the side branches will be compared with the main branch and only the changes will be shown.
That’s all! Have a good day!
Especially for habr.com – Alexey Gubin | AppFox.ru